Python os.fstat() Method
-
Syntax of Python
os.fstat()
Method -
Example 1: Use
os.fstat()
to Get Status of a File -
Example 2: Use
os.stat()
to Get Status of a File - Conclusion
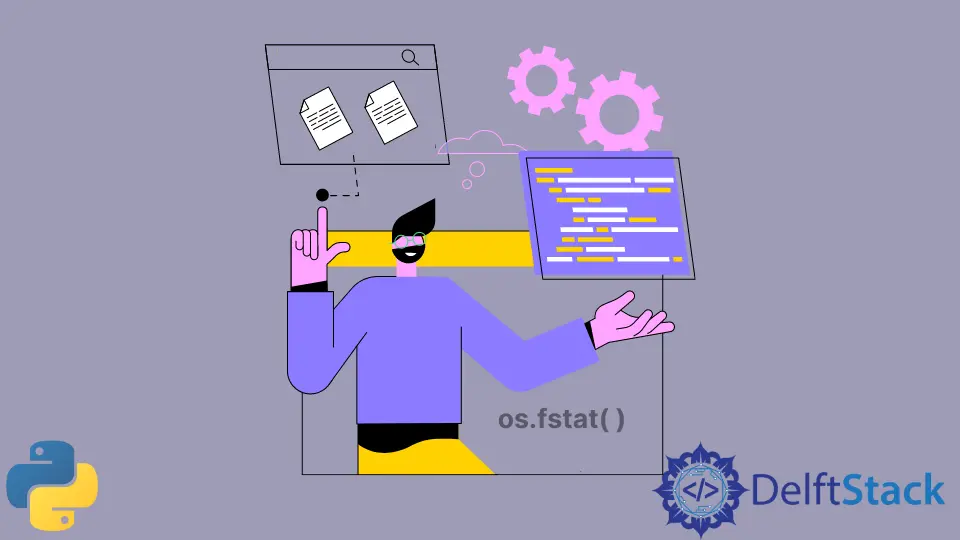
The fstat()
method belongs to the os
module in Python and is employed to retrieve information about a file given its file descriptor.
A file descriptor is a low-level integer representing an open file in an operating system. This method returns an object with file information, such as mode, size, and timestamps, providing a comprehensive snapshot of the file’s attributes
A file descriptor is used to track an open file and perform several operations such as reading, writing, appending, deleting, etc. Additionally, in Python 3.3 and above, os.fstat()
method is equivalent to os.stat()
method.
Syntax of Python os.fstat()
Method
os.fstat(fd)
Parameters
Type | Description | |
---|---|---|
fd |
integer | A file descriptor associated with a valid resource. |
Returns
The fstat()
method returns a stat_result
object that represents the state of a file descriptor. The stat_result
is a class.
The following are some of the essential attributes of the stat_result
class, along with some details.
Attribute | Description |
---|---|
st_mode |
The file type and file permissions or mode bits. |
st_ino |
The file index or the inode number on Windows and Linux, respectively. |
st_dev |
An identifier for the system on which the file exists. |
st_nlink |
Number of hard links of the opened file. |
st_uid |
The user identifier for the opened file’s owner. |
st_gid |
The group identifier for the opened file’s owner. |
st_size |
File’s size in bytes. In the case of a symbolic link, it is the length of the file system path. |
st_atime |
Most recent access time in seconds. |
st_mtime |
Most recent modification time in seconds. |
st_ctime |
Creation time in seconds on Windows and most recent metadata modification time on Unix in seconds. |
st_atime_ns |
Most recent access time in nanoseconds. |
st_mtime_ns |
Most recent modification time in nanoseconds. |
st_ctime_ns |
Creation time in nanoseconds on Windows and most recent metadata modification time on Unix in nanoseconds. |
Apart from the attributes stated above, a few more attributes are platform dependent and may not be available in all operating systems.
To learn about them, refer to the official documentation here.
Example 1: Use os.fstat()
to Get Status of a File
The Python code snippet below is a concise demonstration of using the os.open
and os.fstat
functions to interact with a file.
This code is particularly focused on creating a file if it doesn’t already exist and subsequently obtaining file information using os.fstat
.
file.txt
:
This is a text file.
Code:
import os
fd = os.open("file.txt", os.O_CREAT) # Create file if it doesn't exist
print(os.fstat(fd))
os.close(fd)
The Python code snippet demonstrates a streamlined process of file interaction, focusing on the creation or opening of a file using the os.open
function.
By specifying os.O_CREAT
as the second argument, the code ensures the file "file.txt"
is created if it does not already exist, resulting in a file descriptor (fd
).
Subsequently, the os.fstat
function is employed with the file descriptor, generating an os.stat_result
object that encapsulates file details such as size, permissions, and timestamps. The retrieved file information is then printed using the print
statement, offering insights into the file’s attributes.
Finally, the file is closed using os.close
to release system resources associated with the file descriptor.
Output:
The Python code above uses the os.open()
method to read a file. It creates the file if it doesn’t exist.
When this code is executed, it will output information about the file "file.txt,"
by stat_result
object including its size, permissions, and timestamps.
The specific details may vary based on the system and the existing attributes of the file.
Note: The values in output snippet is an example only. Values that will be printed differs in every file.
Example 2: Use os.stat()
to Get Status of a File
For this example, the Python code snippet revolves around file handling, utilizing the os
module for file creation, information retrieval, and proper resource management.
file.txt
:
This is a text file.
import os
fd = os.open("file.txt", os.O_CREAT) # Create file if it doesn't exist
print(os.stat(fd))
os.close(fd)
By employing the os.open
function with the os.O_CREAT
flag, the code ensures the file’s existence, and the resulting file descriptor (fd
) becomes a low-level integer representing the open file.
Subsequently, the os.fstat
function is utilized with the file descriptor to generate an os.stat_result
object, encapsulating essential file details such as size, permissions, and timestamps.
The obtained information is then printed using the print
statement, providing a concise overview of the file’s attributes.
Finally, the code closes the file through the os.close
function, releasing associated system resources.
Output:
As mentioned above, the os.stat()
method is equivalent to os.fstat()
method. Hence, the output is the same.
Conclusion
In conclusion, the os.fstat
method in Python’s os
module proves to be an invaluable tool for retrieving detailed information about files and directories based on their file descriptors.
This method enhances file handling capabilities, offering a comprehensive snapshot of attributes such as size, permissions, and timestamps. The provided examples showcase its versatility in both file and directory scenarios, emphasizing its role in file creation, information retrieval, and proper resource management.
The syntax, parameters, and returns of the os.fstat
method have been elucidated, empowering Python developers to harness its capabilities for various file-related tasks. This method, alongside its counterpart os.stat
, contributes significantly to Python’s file manipulation capabilities.