Python os.stat() Method
Musfirah Waseem
Jan 30, 2023
Python
Python OS
-
Syntax of Python
os.stat()
Method -
Example Codes: Working With the
os.stat()
Method -
Example Codes: Retrieving Some Elements of the
os.stat()
Method
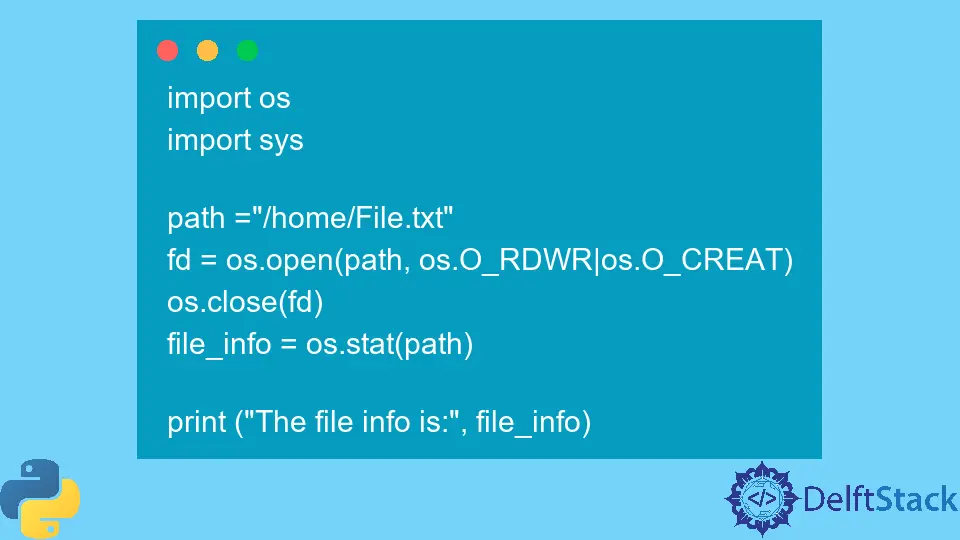
Python os.stat()
method is an efficient way of finding information about a file or a symlink. It is an alias to the os.lstat()
method.
Syntax of Python os.stat()
Method
os.stat(path address)
Parameters
path address |
It is an address object of a file system path or a symlink. The object can either be an str or bytes. |
Return
The return type of this method is all the information about the specified file. The following details are returned.
Return Type | Description |
---|---|
st_dev |
Device ID where the file resides. |
st_ino |
inode number of the file system. |
st_mode |
Permission access to the file. |
st_nlink |
Number of hard links for the file. |
st_uid |
User ID of the file’s owner. |
st_gid |
Group ID of the file’s owner |
st_rdev |
Device ID of a special file. |
st_size |
Total file size in bytes. |
st_blksize |
Total block size for the file system. |
st_blocks |
Number of blocks allocated to the file. |
st_atime |
Last time, the file was accessed. |
st_mtime |
Last time, the file was modified. |
st_ctime |
Last time, the file status was changed. |
Example Codes: Working With the os.stat()
Method
import os
import sys
path = "/home/File.txt"
fd = os.open(path, os.O_RDWR | os.O_CREAT)
os.close(fd)
file_info = os.stat(path)
print("The file info is:", file_info)
Output:
The file info is: os.stat_result(st_mode=33279, st_ino=305353, st_dev=2049, st_nlink=1, st_uid=0, st_gid=0, st_size=33, st_atime=1660396896, st_mtime=1660396896, st_ctime=1660396896)
The method os.stat()
can support any path relative to a given file descriptor.
Example Codes: Retrieving Some Elements of the os.stat()
Method
import os
import sys
path = "/home/File.txt"
fd = os.open(path, os.O_RDWR | os.O_CREAT)
os.close(fd)
file_info = os.stat(path)
print("The device ID is:", file_info[2])
print("The user ID is:", file_info[4])
Output:
The device ID is: 2049
The user ID is: 0
The information returned by the os.stat()
method is in the form of an array, starting from the index number 0. Some elements of this method can be retrieved by specifying the index number.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Musfirah Waseem
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn