Python os.lstat() Method
-
Syntax of Python
os.lstat()
Method -
Example Codes: Working With the
os.lstat()
Method -
Example Codes: Retrieve Some Elements of the
os.lstat()
Method - Conclusion
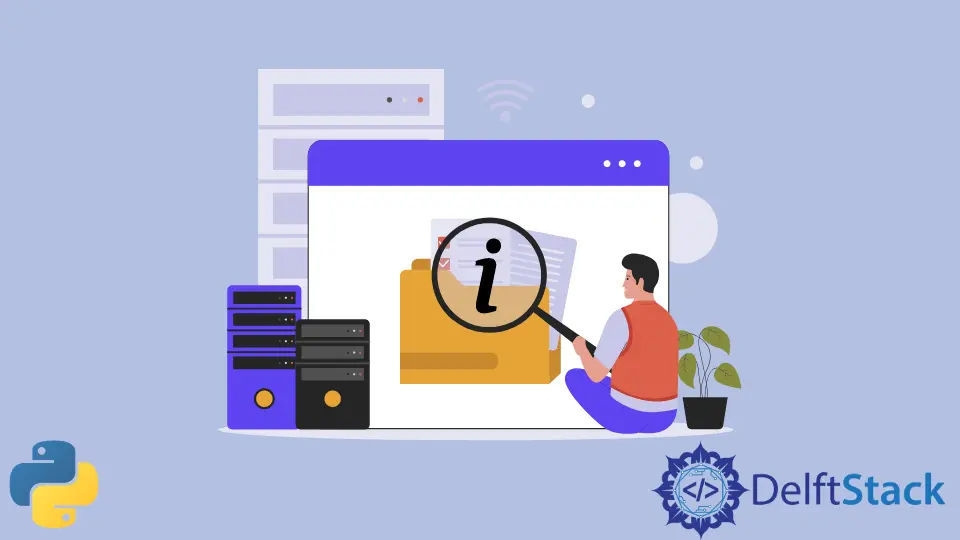
Python os.lstat()
method is an efficient way of finding information about a file. It is an alias to the os.stat()
method that doesn’t support symbolic paths.
When working with files, developers often need to gather information about them, ranging from their size to the last modification time.
The os.lstat()
method provides a snapshot of a file’s status, including its metadata. This can be particularly useful for tasks like monitoring file changes, analyzing storage usage, or ensuring proper permissions.
Syntax of Python os.lstat()
Method
os.lstat(path address)
Parameters
path address |
It is an address object of a file system path or a symlink . The object can either be an str or bytes. |
Return
The return type of this method is all the information about the specified file. The following details are returned.
Return Type | Description |
---|---|
st_dev |
Device ID where the file resides. |
st_ino |
inode number of the file system. |
st_mode |
Permission access to the file. |
st_nlink |
Number of hard links for the file. |
st_uid |
User ID of the file’s owner. |
st_gid |
Group ID of the file’s owner |
st_rdev |
Device ID of a special file. |
st_size |
Total file size in bytes. |
st_blksize |
Total block size for the file system. |
st_blocks |
Number of blocks allocated to the file. |
st_atime |
Last time, the file was accessed. |
st_mtime |
Last time, the file was modified. |
st_ctime |
Last time, the file status was changed. |
Example Codes: Working With the os.lstat()
Method
This example code aims to demonstrate file handling using the os
module, specifically focusing on the os.open()
and os.lstat()
functions.
The primary function of the code is to open a file (or create it if it doesn’t exist), close the file, and then retrieve and display information about the file using os.lstat()
.
SampleFile.txt
:
This is a sample file.
Example Code:
import os
path = "SampleFile.txt"
fd = os.open(path, os.O_RDWR | os.O_CREAT)
os.close(fd)
file_info = os.lstat(path)
print("The file info is:", file_info)
The os
and sys
were imported to use their function in the code snippet.
The variable path
is assigned the string SampleFile.txt
representing the file path. You can replace this with the actual path of the file you want to manipulate.
The os.open()
function is used to open or create a file specified by the given path
. The flags os.O_RDWR | os.O_CREAT
indicate that the file should be opened for both reading and writing (os.O_RDWR
), and if the file does not exist, it should be created (os.O_CREAT
).
After closing the file using os.close(fd)
to release system resources associated with the file, the os.lstat()
function is then used to retrieve information about the file specified by path
.
The resulting file_info
is a stat_result
object containing details such as file size, permissions, timestamps, etc. Finally, it prints the file_info
to see the generated details visually.
Output:
The file info is: os.stat_result(st_mode=33279, st_ino=303997, st_dev=2049, st_nlink=1, st_uid=0, st_gid=0, st_size=33, st_atime=1660394495, st_mtime=1660394495, st_ctime=1660394495)
This output provides a comprehensive overview of the file’s attributes, demonstrating the practical use of os.lstat()
in file manipulation and information retrieval.
The method os.lstat()
can support any path relative to a given file descriptor.
Example Codes: Retrieve Some Elements of the os.lstat()
Method
This example code is designed to showcase how to manipulate files using the os
module, specifically focusing on the retrieval of file information using os. stat ()
and accessing specific attributes of the file.
In this case, the code opens or creates a file specified by the path
, closes it immediately, retrieves information about the file using os.lstat()
, and then prints the device ID and user ID associated with the file.
SampleFile.txt
:
This is a sample file.
Example Code:
import os
import sys
path = "SampleFile.txt"
fd = os.open(path, os.O_RDWR | os.O_CREAT)
os.close(fd)
file_info = os.lstat(path)
print("The device ID is:", file_info[2])
print("The user ID is:", file_info[4])
The os
and sys
were imported to use their function in the code snippet.
The variable path
is assigned the string SampleFile.txt
representing the file path. You can replace this with the actual path of the file you want to manipulate.
The os.open()
function is used to open or create a file specified by the given path
. The flags os.O_RDWR | os.O_CREAT
indicate that the file should be opened for both reading and writing (os.O_RDWR
), and if the file does not exist, it should be created (os.O_CREAT
).
After closing the file using os.close(fd)
to release system resources associated with the file, the os.lstat()
function is then used to retrieve information about the file specified by path
. The resulting file_info
is a stat_result
object containing various details about the file.
Lastly, the code prints the device ID (st_dev
) and user ID (st_uid
) extracted from the file_info
object. These values provide information about the device on which the file resides and the user ID of the file’s owner, respectively.
Output:
The device ID is: 2049
The user ID is: 0
The information returned by the os.lstat()
method is in the form of an array, starting from the index number 0
.
Some elements of this method can be retrieved by specifying the index number.
Conclusion
In conclusion, the os.lstat()
method in Python proves to be a powerful tool for accessing comprehensive information about files. By providing a snapshot of a file’s status, including metadata such as size, permissions, and timestamps, this method facilitates efficient file manipulation and analysis.
In our examples, we demonstrated its usage in both a general context, showcasing file creation and information retrieval, and a specific scenario, highlighting the extraction of device ID and user ID.
Whether monitoring file changes, analyzing storage usage, or ensuring proper permissions, the os.lstat()
method stands as a valuable asset in a Python developer’s toolkit, offering insights into the intricacies of file systems.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn