How to Determine the Size of an Object in Python
- Using the sys.getsizeof() Function
- Using the pympler Module
- Measuring Memory Usage with tracemalloc
- Conclusion
- FAQ
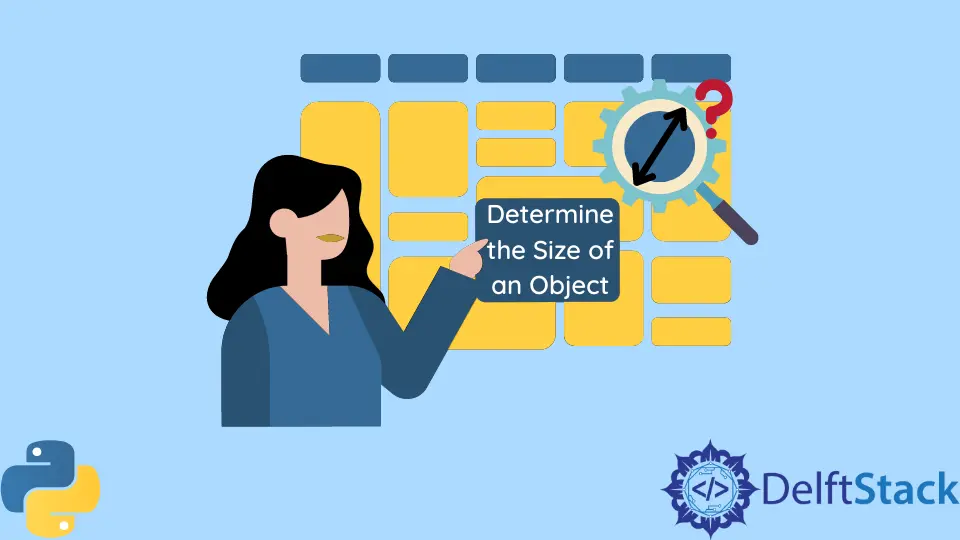
Determining the size of an object in Python is a fundamental skill that every programmer should master. Whether you’re working with simple data types or complex structures, understanding how to measure memory usage can help you optimize your code and improve performance.
In this tutorial, we will explore various methods to determine the size of objects in Python. From built-in functions to third-party libraries, we’ll cover everything you need to know to effectively measure memory consumption. By the end of this article, you’ll be equipped with the tools to analyze and manage the size of your Python objects, leading to more efficient coding practices.
Using the sys.getsizeof() Function
One of the simplest ways to determine the size of an object in Python is by using the built-in sys
module’s getsizeof()
function. This function returns the size of an object in bytes. It’s a straightforward method and works well for most built-in types, including integers, strings, and lists.
Here’s how you can use it:
import sys
my_string = "Hello, World!"
my_list = [1, 2, 3, 4, 5]
my_dict = {'key1': 'value1', 'key2': 'value2'}
string_size = sys.getsizeof(my_string)
list_size = sys.getsizeof(my_list)
dict_size = sys.getsizeof(my_dict)
print(f"Size of string: {string_size} bytes")
print(f"Size of list: {list_size} bytes")
print(f"Size of dictionary: {dict_size} bytes")
Output:
Size of string: 27 bytes
Size of list: 56 bytes
Size of dictionary: 240 bytes
The sys.getsizeof()
function provides a quick way to check the size of an object. However, it’s worth noting that this function only returns the size of the object itself and does not account for the sizes of objects that are referenced within it. For example, if you have a list containing other lists or dictionaries, the sizes of these nested objects will not be included in the output. This limitation can lead to underestimating the total memory usage, especially for complex data structures.
Using the pympler Module
For a more comprehensive analysis of object sizes, you can use the pympler
library. This third-party library provides a more detailed view of memory usage, including the sizes of nested objects. To use pympler
, you first need to install it via pip:
pip install pympler
Once installed, you can use the asizeof
function from the pympler.asizeof
module to get the total size of an object, including its contents.
Here’s an example:
from pympler import asizeof
my_complex_list = [1, 2, [3, 4], {'key': 'value'}]
complex_list_size = asizeof.asizeof(my_complex_list)
print(f"Total size of complex list: {complex_list_size} bytes")
Output:
Total size of complex list: 104 bytes
The asizeof
function provides a more accurate measurement by recursively calculating the sizes of all objects contained within the specified object. This means if your list contains other lists or dictionaries, their sizes will also be included in the total. This is particularly useful for developers working with data structures where memory usage is a concern. By utilizing pympler
, you can gain insights into the memory footprint of your applications, allowing for better optimization and resource management.
Measuring Memory Usage with tracemalloc
Another powerful tool for measuring memory usage in Python is the tracemalloc
module. This module is built into Python and provides a way to trace memory allocations in your program. It’s particularly useful for identifying memory leaks and understanding memory consumption over time.
To use tracemalloc
, you need to start tracing memory allocations at the beginning of your code, and then you can take snapshots of the memory usage at different points in time.
Here’s how you can implement it:
import tracemalloc
tracemalloc.start()
my_list = [i for i in range(1000)]
current, peak = tracemalloc.get_traced_memory()
print(f"Current memory usage: {current / 10**6} MB; Peak was {peak / 10**6} MB")
tracemalloc.stop()
Output:
Current memory usage: 0.017 MB; Peak was 0.017 MB
In this example, we start by calling tracemalloc.start()
, which begins tracking memory allocations. After creating a list of 1000 integers, we call tracemalloc.get_traced_memory()
to retrieve the current and peak memory usage. The values are displayed in megabytes for easier interpretation. Finally, we stop the tracing with tracemalloc.stop()
. This method is particularly useful for profiling memory usage in larger applications, as it allows you to pinpoint where memory is being allocated and how much is being used at various stages of your program’s execution.
Conclusion
Understanding how to determine the size of an object in Python is essential for writing efficient code. Whether you choose to use the built-in sys.getsizeof()
function, the more comprehensive pympler
library, or the powerful tracemalloc
module, each method has its unique advantages. By mastering these techniques, you can better manage memory usage in your applications, optimize performance, and ultimately create more robust Python programs. Start implementing these methods today and take control of your Python memory management.
FAQ
-
How do I install the pympler library?
You can install pympler using pip by running the command pip install pympler in your terminal. -
What is the difference between sys.getsizeof() and pympler’s asizeof()?
sys.getsizeof() returns the size of the object itself, while pympler’s asizeof() includes the sizes of nested objects as well. -
Can tracemalloc help with memory leaks?
Yes, tracemalloc is useful for identifying memory leaks by tracking memory allocations and providing insights into memory usage over time. -
Is it necessary to import any libraries to use sys.getsizeof()?
No, sys.getsizeof() is a built-in function in Python, so you don’t need to install or import any external libraries. -
How can I measure the size of custom objects in Python?
You can measure the size of custom objects using sys.getsizeof() or by implementing the sizeof() method in your class to customize its size calculation.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn