How to Redirect Print Output to a File in Python
- Understanding File Writing in Python
-
How to Write to File in Python Using the
open()
andwrite()
Functions -
How to Write to File in Python Using the
print()
Function Withfile
Parameter -
How to Write to File in Python Using
sys.stdout
-
How to Write to File in Python Using the
contextlib.redirect_stdout()
Function -
How to Write to File in Python Using the
writelines()
Function -
How to Write to File in Python Using Context Managers With
pathlib
- Conclusion
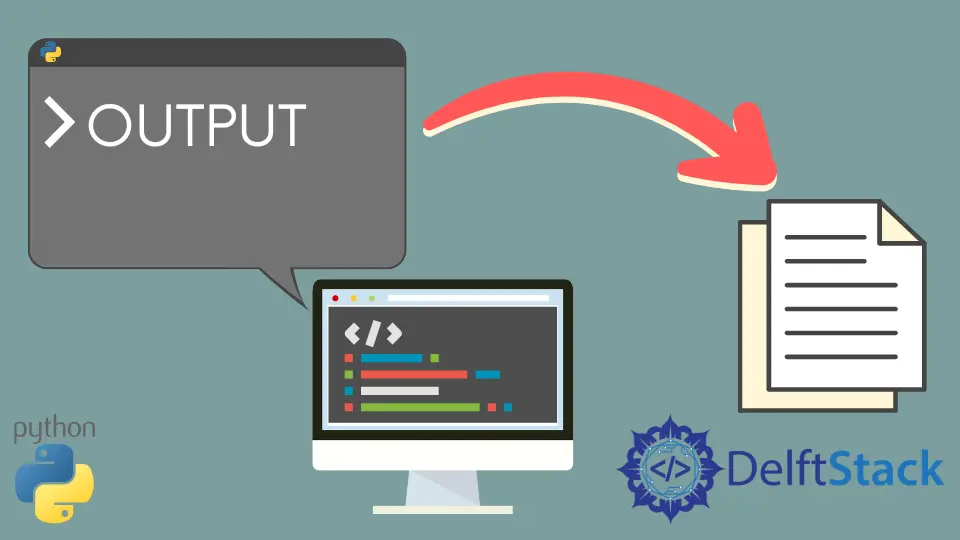
File handling is a basic aspect of programming, and Python offers an array of methods to efficiently write data into files. Whether you’re dealing with text or binary files, logs, or structured data, understanding the various techniques for file writing empowers you to handle diverse scenarios adeptly.
In this article, we’ll delve into multiple approaches Python offers to write data into text files. From the foundational use of open()
, write()
, and print()
to leveraging advanced functionalities like pathlib
, contextlib
, and sys.stdout
, each method is tailored to cater to different needs and preferences.
Understanding File Writing in Python
In Python, the process of file writing encompasses the insertion of various types of data into a file, such as text, binary data, or structured information. This operation adheres to a sequence of core steps for successful file manipulation:
Opening a File
The initial step involves utilizing the open()
function, which acts as a gateway to either create a new file or access an existing one.
When calling open()
, it’s essential to specify the file path along with the desired mode, denoted by parameters like 'w'
for writing or 'a'
to append content to an existing file. This operation establishes the connection between the program and the file, setting the stage for subsequent data insertion.
Writing Data
Once the file is opened with the appropriate mode, Python provides several methods to write data into the file. The write()
method directly inserts text or information into the file, while the print()
function, augmented with the file
parameter, streamlines the process by redirecting the output to the specified file object.
Additionally, the writelines()
method proves advantageous for writing sequences of strings or lines into the file, efficiently handling multiple data entries.
More methods for writing data into the file will be discussed further in this article.
Closing the File
Properly closing the file after performing writing operations is crucial. It ensures that resources associated with the file are released and changes are finalized.
Utilizing the with
statement in conjunction with open()
guarantees that the file is automatically closed upon exiting the block of code, enhancing efficiency and mitigating potential resource leaks or data corruption.
Example:
with open("example.txt", "w") as file:
file.write("This is an example of Python write to file.")
# File is automatically closed outside the 'with' block
The simple script above utilizes Python’s with
statement to open a file named example.txt
in write mode and write the text "This is an example of Python write to file."
into it.
Crucially, the with
statement ensures automatic closure of the file once the writing operation concludes, eliminating the need for explicit file closure and enhancing code readability and reliability by guaranteeing proper file handling, even in the presence of errors.
Closing the File Explicitly
Explicitly closing the file using the close()
method is an alternative approach, but utilizing the with
statement for file handling ensures more robust and error-resistant code.
file = open("example.txt", "w")
file.write("This is an example of Python closed file.")
file.close() # Explicitly closing the file
The Python script first opens a file named example.txt
in write mode writes the text "This is an example of Python closed file."
into it and explicitly closes the file using the close()
method.
Unlike the with
statement, which automatically manages file closure, this method requires manual handling of file closure. While functional, manual closure increases the risk of forgetting to close the file, potentially leading to resource issues if not handled meticulously.
Understanding and adhering to these fundamental steps—opening a file, inserting data using appropriate methods, and ensuring it’s properly closed—facilitates efficient and reliable file-writing operations in Python, allowing developers to manage data storage and manipulation seamlessly.
How to Write to File in Python Using the open()
and write()
Functions
File manipulation is an important aspect of programming, especially when it comes to storing data persistently. Python simplifies file operations with its intuitive file-handling capabilities.
Among the fundamental methods for reading and writing data into files, the open()
function combined with the write()
function stands as a cornerstone.
the open()
Function
The open()
function serves as a gateway to interact with files in Python. Its basic syntax is:
open(
file,
mode="r",
buffering=-1,
encoding=None,
errors=None,
newline=None,
closefd=True,
opener=None,
)
file
: The file path or name to be opened.mode
: Specifies the file opening mode. The default mode is'r'
(read).buffering
: Optional buffer size for file reading or writing.encoding
,errors
,newline
: Optional parameters to handle text files.closefd
,opener
: Advanced parameters for low-level file descriptor manipulation.
Writing With write()
Function
Once a file is opened for writing, the write()
function is used to insert data into the file.
write()
is a built-in function that helps in either writing binary files or adding a specified text into a file. It allows you to write strings, bytes, or byte arrays directly into the file.
'w'
and 'a'
are the 2 operations in this function that will write or add any text in a file. w
is used when the user wants to empty the file before writing anything in it. Whereas a
is used when the user just wants to add some text to the existing content in the file.
Its syntax is straightforward:
file.write(string)
file
: The file object obtained through theopen()
function.string
: The data or string to be written into the file.
Python Write to File
Let’s look at the following example of how to write textual data into a file using the write()
function:
# Open a file in write mode
with open("text_file.txt", "w") as file:
file.write(
"This is an example of writing text to a file using the write() function."
)
Here, the provided string "This is an example..."
will be written into the file text_file.txt
as the file name. If the file doesn’t exist, it will be created. If the file exists, its file contents will be replaced by an empty string and the new text.
Writing Multiple Lines
The write()
function doesn’t automatically add line breaks. To write multiple lines, including newline characters (\n
) in line endings, is essential:
# Open a file in write mode
with open("multiple_lines.txt", "w") as file:
file.write("Line 1\n")
file.write("Line 2\n")
file.write("Line 3\n")
This code snippet writes three lines of text into a file with multiple_lines.txt
as the file name, each terminated by a newline character (\n
), ensuring separation between lines.
Writing to a File Using write()
With open()
in Append Mode
Appending data to an existing file can be achieved by using 'a'
as a mode argument in the open()
function. Subsequent write()
calls will add content to the end of the file.
Example:
with open("randomfile.txt", "a") as o:
o.write("Hello")
o.write("This text will be added to the file")
The open()
function with 'a'
mode opens the file in append mode, allowing content to be added to the end of the file without overwriting existing data (append data).
How to Write to File in Python Using the print()
Function With file
Parameter
The print()
function in Python allows for the output of data to various streams, including files, by specifying the file
parameter. This parameter directs the function’s output to the specified file object.
The syntax for using the print()
function with the file
parameter to write to a file is as follows:
print(*objects, sep=" ", end="\n", file=sys.stdout, flush=False)
Here, the file
parameter is used to specify the file object where the output should be directed. The parameters within the print()
function have the following significance:
*objects
: Represents zero or more objects to be printed. Commas can separate multiple objects.sep=' '
: Defines the separator between the objects being printed. By default, it’s a space character.end='\n'
: Specifies the string to be printed at the end. By default, it’s a new line character (\n
).file=sys.stdout
: Redirects the output to the specified file object. By default, it directs the output to the standard output (sys.stdout
).flush=False
: If set toTrue
, flushes the output buffer. By default, it’s set toFalse
.
In this method, first, we call the open()
function to open the desired file. After that, the print()
function is used to print the text in the file by specifying the file=external_file
parameter within the function.
It is always the user’s choice to either use the w
operator or the a
operator.
Example:
with open("randomfile.txt", "w") as external_file:
add_text = "This text will be added to the file"
print(add_text, file=external_file)
external_file.close()
This code redirects the standard output stream (sys.stdout
) to a file named "randomfile.txt"
and writes the specified text into that file, effectively adding the single line "This text will be added to the file"
as its content.
Note that before using the sys.stdout
as an object to open and print the statement in the text file, a definite path of the file must be defined by the user. Otherwise, none of the operations can be performed on the file.
How to Write to File in Python Using the contextlib.redirect_stdout()
Function
The contextlib.redirect_stdout()
function redirects the standard output to a target file-like object within a specified context. This context manager ensures that any output generated within its scope is directed to the designated file, enabling streamlined file writing without modifying the print statements or standard output streams directly.
The contextlib
module is generally used with the with
statement. The contextlib.redirect_stdout()
function helps to redirect the sys.stdout
to some file on a temporary basis by setting up a context manager.
Let’s delve into an example demonstrating the redirection of output to a file using redirect_stdout()
:
import contextlib
file_path = "randomfile.txt"
with open(file_path, "w") as o:
with contextlib.redirect_stdout(o):
print("This text will be added to the file")
As you can see, the with
statement is used with the operations of the contextlib
module.
In this example, the output generated by the print()
statement within the redirect_stdout()
context manager is written into the file named redirected_output.txt
. All standard output within the context block is seamlessly redirected to the specified file object obtained through open()
.
How to Write to File in Python Using the writelines()
Function
The writelines()
function is a method specifically designed to write a sequence of strings to a file. It is particularly useful when dealing with lists or sequences of strings that need to be written consecutively into a file.
The syntax for using the writelines()
function in Python to write to a file is as follows:
file.writelines(lines)
Here, file
represents the file object obtained through the open()
function, and lines
is an iterable containing strings or byte objects to be written into the file.
The writelines()
function expects an iterable as its argument, where each element of the iterable should be a string or a byte object that will be written to the file.
This function is used to write a list of strings to a file. It doesn’t automatically add line breaks, so they need to be included in the list of elements if needed.
Let’s dive into an example of how to use writelines()
to write multiple lines into a file:
# List of strings to be written to a file
lines = ["Line 1\n", "Line 2\n", "Line 3\n"]
# Open a file in write mode
with open("file.txt", "w") as file:
file.writelines(lines)
In this example, the list lines
containing three strings is written into the file multi_lines.txt
using the writelines()
function. Each string in the list represents a line, and the \n
character adds a new line after each line.
How to Write to File in Python Using Context Managers With pathlib
pathlib
is a module introduced in Python 3.4 that offers classes representing filesystem paths. Its intuitive interface facilitates file system interaction by treating paths as objects, enabling concise and platform-independent code.
Python’s pathlib
module provides an object-oriented interface for file paths. It can be used along with context managers for file I/O.
Let’s explore how to use pathlib
along with context managers for file writing:
from pathlib import Path
# Writing to a file using pathlib
with Path("file.txt").open("w") as file:
file.write("Writing with pathlib.")
In this example, a Path
object representing the file path (file.txt
) is created using Path('file.txt')
. The open()
method of the Path
object is used with the 'w'
as the mode parameter to open the file for writing, and the write()
method is employed to insert new data into the file within the context manager.
Conclusion
File handling is an indispensable aspect of software development, and Python offers a diverse range of methodologies to facilitate efficient and flexible file-writing operations. From the foundational open()
and write()
functions to advanced techniques involving print()
with the file
parameter, sys.stdout
, contextlib.redirect_stdout()
, writelines()
, and the amalgamation of pathlib
with context managers, each method brings its strengths and use cases to the table.
Understanding these varied approaches empowers developers to tailor their file writing operations to specific requirements, whether it involves writing text, appending data, handling different data or file types, or redirecting output to different streams or files.
Now that you have learned to write files with data, read this article on how to read a file into a string in Python.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn