Python sys.stdout Method
-
Syntax of Python
sys.stdout
Method -
Example Codes: Use the
sys.stdout
Method in Python -
Example Codes:
sys.stdout.write()
vsprint()
Method -
Example Codes: Use the
sys.stdout.write()
Method to Display a List -
Example Codes: Use the
sys.stdout.flush()
Method in Python -
Example Codes: Use the
sys.stdout.encoding()
Method in Python -
Example Codes: Duplicate
sys.stdout
to a Log File
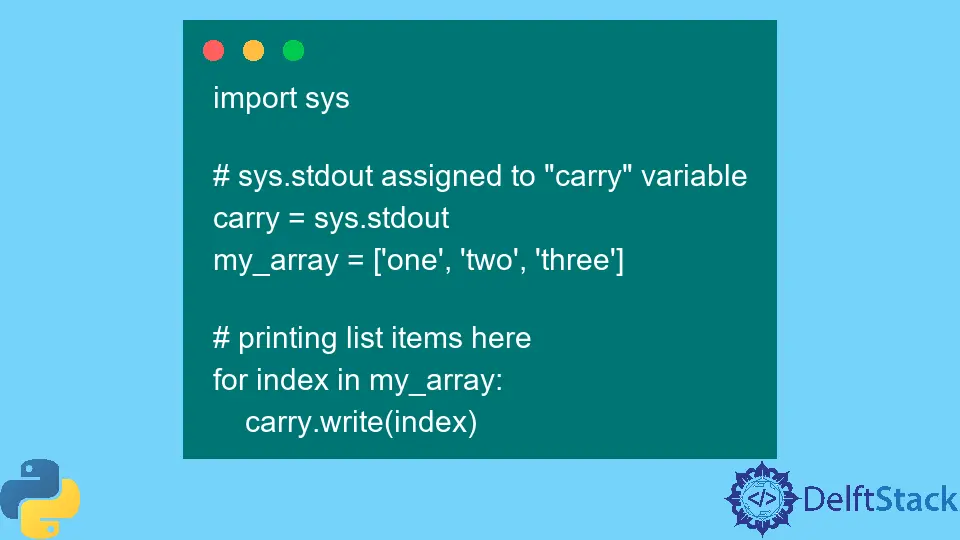
One of the methods in the sys
module in Python is stdout
, which uses its argument to show directly on the console window.
These kinds of output can be different, like a simple print statement, an expression, or an input prompt. The print()
method, which has the same behavior, first converts to the sys.stdout()
method and then shows the result on the console.
Syntax of Python sys.stdout
Method
sys.stdout
Parameters
No parameter is involved. We use sys.stdout
for the output file object.
Return
This method doesn’t return any value but only shows output directly on the console.
Example Codes: Use the sys.stdout
Method in Python
# import the sys module to use methods
import sys
sys.stdout.write("This is my first line")
sys.stdout.write("This is my second line")
Output:
This is my first line This is my second line
It will return the passed argument in the sys.stdout.write()
method and display it on the screen.
Example Codes: sys.stdout.write()
vs print()
Method
import sys
# print shows new line at the end
print("First line ")
print("Second line ")
# displays output directly on console without space or newline
sys.stdout.write("This is my first line ")
sys.stdout.write("This is my second line ")
# for inserting new line
sys.stdout.write("\n")
sys.stdout.write("In new line ")
# writing string values to file.txt
print("Hello", "World", 2 + 3, file=open("file.txt", "w"))
Output:
First line
Second line
This is my first line This is my second line
In new line
# file.txt will be created with text "Hello World 5" as a string
We use the sys.stdout.write()
method to show the contents directly on the console, and the print()
statement has a thin wrapper of the stdout()
method that also formats the input. So, by default, it leaves a space between arguments and enters a new line.
After Python version 3.0, print()
method not only takes stdout()
method but also takes a file argument. To give a line space, we pass the "\n"
to the stdout.write()
method.
Example Codes: Use the sys.stdout.write()
Method to Display a List
import sys
# sys.stdout assigned to "carry" variable
carry = sys.stdout
my_array = ["one", "two", "three"]
# printing list items here
for index in my_array:
carry.write(index)
Output:
# prints a list on a single line without spaces
onetwothree
The output shows that the stdout.write()
method gives no space or new line to the provided argument.
Example Codes: Use the sys.stdout.flush()
Method in Python
import sys
# import for the use of the sleep() method
import time
# wait for 5 seconds and suddenly shows all output
for index in range(5):
print(index, end=" ")
time.sleep(1)
print()
# print one number per second till 5 seconds
for index in range(5):
# end variable holds /n by default
print(index, end=" ")
sys.stdout.flush()
time.sleep(1)
Output:
0 1 2 3 4 # no buffer
0 1 2 3 4 # use buffer
The sys.stdout.flush()
method flushes the buffer. It means that it will write things from buffer to console, even if it would wait before it does that.
Example Codes: Use the sys.stdout.encoding()
Method in Python
# import sys module for stdout methods
import sys
# if the received value is not None, then the function prints repr(receivedValue) to sys.stdout
def display(receivedValue):
if receivedValue is None:
return
mytext = repr(receivedValue)
# exception handling
try:
sys.stdout.write(mytext)
# handles two exceptions here
except UnicodeEncodeError:
bytes = mytext.encode(sys.stdout.encoding, "backslashreplace")
if hasattr(sys.stdout, "buffer"):
sys.stdout.buffer.write(bytes)
else:
mytext = bytes.decode(sys.stdout.encoding, "strict")
sys.stdout.write(mytext)
sys.stdout.write("\n")
display("my name")
Output:
"my name"
The method sys.stdout.encoding()
is used to change the encoding for the sys.stdout
. In the method display()
, we use it to evaluate an expression inserted in an interactive Python session.
There is an exception handler with two options: if the argument value is encodable, then encode with the backslashreplace
error handler. Otherwise, if it is not encodable, it should encode with the sys.std.errors
error handler.
Example Codes: Duplicate sys.stdout
to a Log File
import sys
# method for multiple log saving in txt file
class multipleSave(object):
def __getattr__(self, attr, *arguments):
return self._wrap(attr, *arguments)
def __init__(self, myfiles):
self._myfiles = myfiles
def _wrap(self, attr, *arguments):
def g(*a, **kw):
for f in self._myfiles:
res = getattr(f, attr, *arguments)(*a, **kw)
return res
return g
sys.stdout = multipleSave([sys.stdout, open("file.txt", "w")])
# all print statement works here
print("123")
print(sys.stdout, "this is second line")
sys.stdout.write("this is third line\n")
Output:
# file.txt will be created on the same directory with multiple logs in it.
123
<__main__.multipleSave object at 0x00000226811A0048> this is second line
this is third line
To store output console results in a file, we can use the open()
method to store it. We store all the console outputs in the same log file.
This way, we can store any output printed to the console and save it to the log file.