Python sys.argv List
-
Syntax of Python
sys.argv
List -
Example Codes: Use of the
sys.argv
List -
Example Codes: Addition/Subtraction Using the
sys.argv
List -
Example Codes: Working With the
sys.argv[1]
Method -
Example Codes: Check if
sys.argv
Is Empty -
Example Codes:
IndexError: List Index Out of Range
When Usingsys.argv
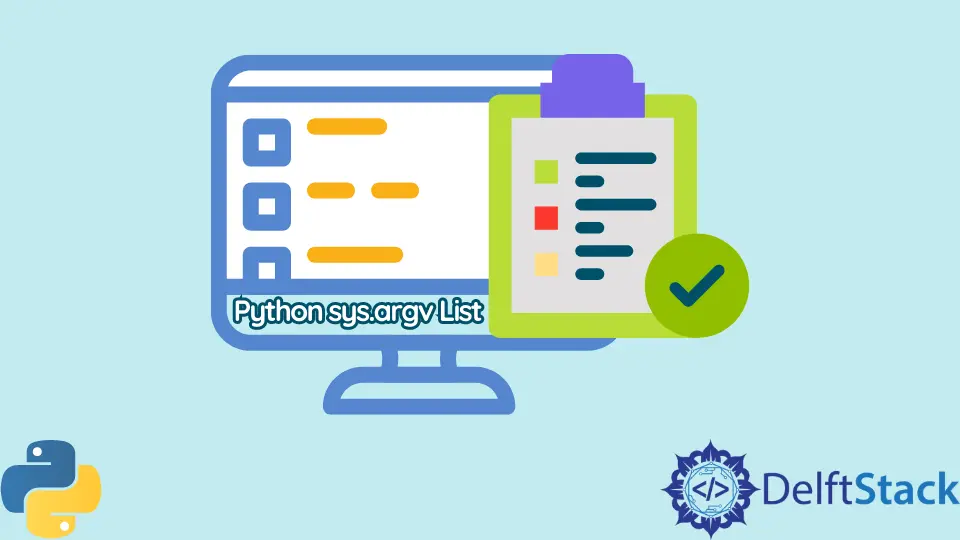
To interact with the run time environment in Python, we use the variables and functions provided by the sys
module. The sys
module also provides functions and variables to communicate with the interpreter.
We use sys.argv()
to have a list of command line arguments passed to the Python script. The sys.argv()
list retrieves like an array, and we use the sys
module.
So, the first element of argv
or the argv[0]
is the name of the Python script we are working on. It depends on the operating system type to output the full pathname or not for the argv[0]
array element.
Hence, in Python, sys.argv()
is an array of command line arguments. We can use the len()
method to check the array’s length.
Syntax of Python sys.argv
List
sys.argv[list]
Parameter
No parameter is passed to this method. Although, a list of command line arguments is passed to the Python script.
Return
It does not return any value.
Example Codes: Use of the sys.argv
List
As we know, sys.argv
is a built-in command that enlists all the command line arguments. We use this command to retrieve the first index element, the length of the array, and to convert and show the elements in the array as a list of strings.
In the example below, the source code will show the above findings and the additional arguments output.
# import the sys module to use sys.argv
import sys
# print the first element as name of the file
print("First argument output: ", sys.argv[0])
# check the length of list
print("Length of the arguments: ", len(sys.argv))
print("Show the arguments are: ", str(sys.argv))
# add more arguments on indexes 1 and 2
sys.argv.insert(1, "Second item")
sys.argv.insert(2, 123)
print("First argument output: ", sys.argv[0])
# check the length again
print("Length of the arguments: ", len(sys.argv))
# new list printed
print("Show the arguments are: ", str(sys.argv))
Output:
First argument output: C:\Users\lenovo\.spyder-py3\temp.py
Length of the arguments: 1
Show the arguments are: ['C:\\Users\\lenovo\\.spyder-py3\\temp.py']
# the first argument is the file name
First argument output: C:\Users\lenovo\.spyder-py3\temp.py
# two arguments added later
Length of the arguments: 3
# total 3 arguments shown here
Show the arguments are: ['C:\\Users\\lenovo\\.spyder-py3\\temp.py', 'Second item', 123]
Example Codes: Addition/Subtraction Using the sys.argv
List
With sys.argv
on runtime, we take the input from the user as arguments and output the sum and subtraction to the console. There is no limit on the number of arguments given as the loop controls the condition by counting the arguments first in the source code below.
import sys
add = 0.0
diff = 0.0
# check the no. of arguments
n = len(sys.argv)
# loop executes for the total no of elements in the list
for i in range(1, n):
# adding and subtracting arguments provided from command line argument
add += float(sys.argv[i])
diff -= float(sys.argv[i])
# output sum and difference
print("the sum is :", add)
print("the difference is :", diff)
Write this line on the command prompt:
python FileName.py 10 5 2
Output:
the sum is : 17
the difference is : 3
Example Codes: Working With the sys.argv[1]
Method
We have executed the program using the command line and the arguments in the above source code. So, when we provide arguments with the Python statement on the command line, the arguments get stored in a list known as sys.argv
.
So, if we run the below line on the command prompt, it stores the hello
and world
to the list. Then, the sys.argv[0]
has the file’s name, and the length of sys.argv
is 3.
python myfile.py hello world
The output of sys.argv
:
# sys.argv zeroth index always has the file name
sys.argv[0] = fileName.py
# first and second index values
sys.argv[1] = hello
sys.argv[2] = world
Example Codes: Check if sys.argv
Is Empty
The above discussion shows that the arguments passed on the command line using the sys.argv
command is stored in a list. Later, we will use the list to retrieve the elements from the list using the len()
method.
Similarly, if sys.argv
has no elements or is empty, we can check it using the following source code.
import sys
# check if the list is empty
if len(sys.argv) > 1:
# if the list is not empty
length_check = sys.argv[1]
else:
# if the list is empty
length_check = "Nothing inside a list"
print(length_check)
Output:
# this output shows that the sys.argv contains an empty list
Nothing inside a list
Example Codes: IndexError: List Index Out of Range
When Using sys.argv
In the above program, we checked if the sys.argv
is empty or not. This may output the IndexError: list index out of range
.
This happens when we access the elements of the sys.argv
list, but no index is available. The Python interpreter outputs the IndexError
on the console.
To handle such a situation, we check the elements in a list first and then access the elements accordingly. The output shows that the list is empty and returns the file’s name instead.
import sys
def myfunction(element):
print("Your element is =" + element)
def main(args):
# check if there is an element that existed
if len(sys.argv) > 1:
# pass the first element on the list if not empty
myfunction(args[1])
# otherwise, it returns the name of the file
else:
myfunction(args[0])
if __name__ == "__main__":
main(sys.argv)
Output:
# output shows that sys.argv list is empty and returns the name of the file
Your element is = C:\Users\lenovo\.spyder-py3\untitled0.py
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn