Python sys.stdin Attribute
-
Syntax of Python
sys.stdin
Attribute -
Example 1: Use
sys.stdin
to Read Data From the Command Line -
Example 2: Use
sys.stdin
to Read Data From a Text File
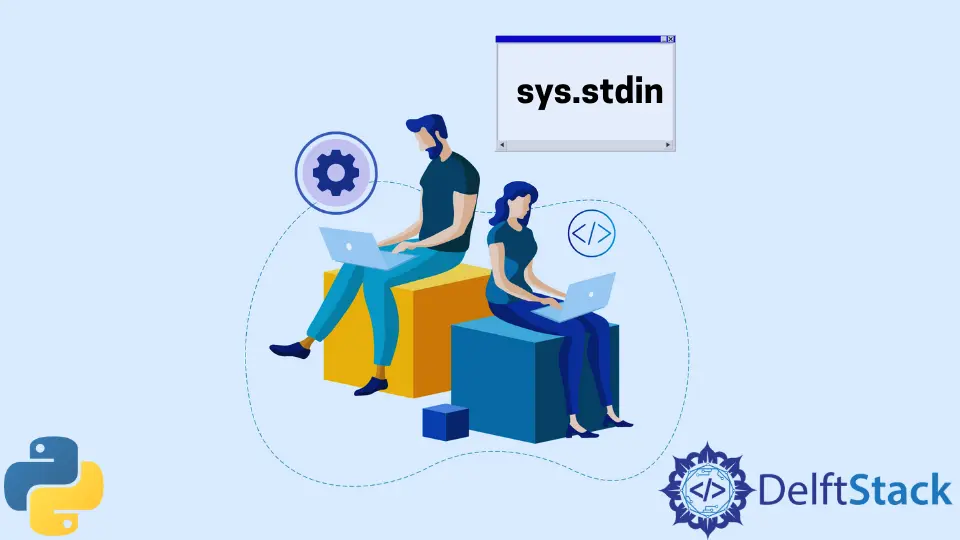
The sys
module offers several methods that help interact with the Python interpreter, and various variables maintained and used by the interpreter. This module has an attribute sys.stdin
, which is a file object like every other file object in Python.
The only difference is that sys.stdin
is automatically opened by Python before the program even starts, and other file objects are opened by the programmer whenever needed. The sys.stdin
is connected to the command line or TTY (TeleTYpe) and reads user inputs from it.
Internally, it is connected to the file descriptor 0
. On a Windows machine, it is the file handler 0
.
Since it needs some data source, and by default, the terminal is the data source, we can also change it and redirect it to read data from a text file.
The built-in input()
method in Python programming language that is mostly used to take user inputs also uses sys.stdin
behind the scenes.
Syntax of Python sys.stdin
Attribute
for line in sys.stdin:
# do something with the input [line]
With this syntax, the for
loop executes indefinitely, waiting for the user’s input. After processing the information, it again goes back to waiting.
Hence, a developer must include a strategy to terminate the loop without breaking the application. We’ll talk about two approaches ahead in this article.
Parameters
Since sys.stdin
is a file object, it does not accept any parameters.
Returns
Since sys.stdin
is a file object, it does not return anything. It’s a file object that we can use to read command line inputs from the user or a file.
Example 1: Use sys.stdin
to Read Data From the Command Line
import sys
print("Enter your favorite subjects:")
try:
for line in sys.stdin:
print(line)
except KeyboardInterrupt:
print("Program terminated using (Ctrl + C)!")
Output:
Enter your favorite subjects:
Computer Science
Computer Science
Psychology
Psychology
History
History
Mathematics
Mathematics
Program terminated using (Ctrl + C)!
The Python code above executes indefinitely and processes the user input until the execution is halted using a keyboard interrupt.
One can raise a key interrupt using Ctrl+C. The above code uses try
and except
blocks.
Sometimes, the use of try
and except
blocks is criticized because they can make codebases bloated and impact readability.
Furthermore, these blocks are meant to catch exceptions and are expected to be handled well without breaking the application, which is not a piece of cake on many occasions. Hence, developers use an approach that associates a unique keyword or text with some particular task.
Whenever a program encounters this special keyword, something unique happens, which in our case, is the termination of the infinite loop. Refer to the following code to understand this approach.
import sys
print("Enter your favorite subjects:")
for line in sys.stdin:
if line.strip().lower() == "exit":
print("Program terminated using a special text or keyword!")
break
else:
print(line)
Output:
Enter your favorite subjects:
Computer Science
Computer Science
Psychology
Psychology
History
History
Mathematics
Mathematics
EXIT
Program terminated using a special text or keyword!
In the above Python code, we associated the string "exit"
with the termination of the infinite loop. If the user types "exit"
, the program will terminate.
Note that we do perform some sanitation over the input to verify if it’s a termination keyword or not. The strip()
removes all the leading and ending spaces, and lower()
converts the string to lowercase.
If the input matches with the string "exit"
, the break
instruction executes and terminates the infinite loop and the program.
Example 2: Use sys.stdin
to Read Data From a Text File
To read data from a text file, create a file, data.txt
and add the following.
Computer Science
Psychology
History
Mathematics
EXIT
Save the file and the upcoming Python code in main.py
. Note that we can use any names to name files.
Next, to use this file to read inputs, we have to use the following terminal command to execute the Python script.
python main.py < data.txt
This command tells the Python interpreter to use data.txt
for user inputs for the Python script main.py
.
import sys
print("Enter your favorite subjects:")
for line in sys.stdin:
if line.strip().lower() == "exit":
print("Program terminated using a special text or keyword!")
break
else:
print(line, end="")
Output:
Enter your favorite subjects:
Computer Science
Psychology
History
Mathematics
Program terminated using a special text or keyword!
At a time, a single line from the text file is read and processed. When the script encounters the string EXIT
, it terminates the loop and the program.
One can also read all the data at once and store it in a list of strings. The sys.stdin.readlines()
method lets us read all inputs at once and returns a list of strings.
Refer to the following Python code to understand this approach.
import sys
data = sys.stdin.readlines()
print(data)
for line in data:
print(line)
Output:
[
'Computer Science\n',
'Psychology\n',
'History\n',
'Mathematics\n',
'EXIT'
]
Computer Science
Psychology
History
Mathematics
EXIT