Python sys.maxsize() Method
-
Syntax of Python
sys.maxsize
Method -
Example Codes: Use of the
sys.maxsize()
Method -
Example Codes: Check the Maximum Size of a List Using the
sys.maxsize()
Method -
Example Codes: The
sys.maxsize()
vs.sys.maxint
Methods -
Example Codes: Use
csv.field_size_limit(sys.maxsize)
in Python - Conclusion
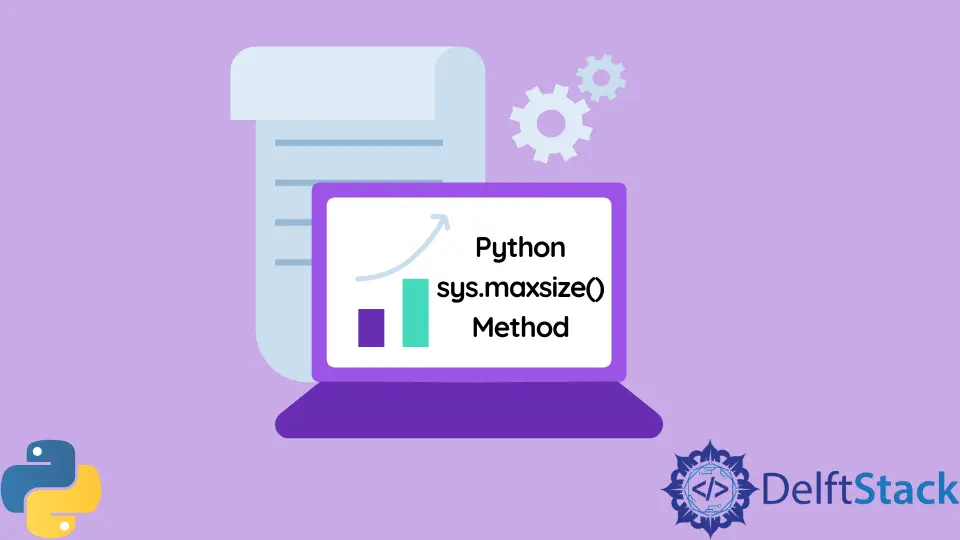
In Python, one such tool that may not be immediately apparent to beginners but holds great significance is the sys.maxsize
attribute. This attribute, found in the sys
module, represents the maximum size of a Python int
– essentially the largest integer that can be represented on your system.
Understanding sys.maxsize
becomes crucial when dealing with platform-independent code or scenarios where integer overflow could be a concern.
This method returns the largest value a variable of Py_ssize_t
can hold.
The Py_ssize_t
is an integer that gives the maximum value a variable can take. The sizes are different depending on the bits of your operating system.
The 32-bit has a size of (2 power 31)-1
, and 64-bit has a (2 power 63)-1
size.
Syntax of Python sys.maxsize
Method
sys.maxsize()
Parameter
This method does not take any parameters.
Return
This method returns the maximum size value of Py_ssize_t
depending on the platform type.
Example Codes: Use of the sys.maxsize()
Method
To implement the method sys.maxsize()
and check the maximum size value, we can import the sys
module and use the method maxsize()
. Depending on the platform architecture type, the sys.maxsize()
method returns its maximum value size on the console.
Below is the implementation of the 32-bit and 64-bit OS and running the same sys.maxsize()
method.
32-Bit Platform
The code snippet showcases the usage of sys.maxsize
to retrieve and display the maximum size of an integer on a 32-bit platform.
Code:
# import the sys module to use the maxsize() method
import sys
# returns the maximum size
size = sys.maxsize
print("The maximum size of a 32-bit platform is:", size)
The code snippet begins by importing the sys
module to access its functionality.
Following this, the maxsize
method from the sys
module is used to obtain the maximum size of an integer on the system, and the result is stored in the variable size
.
The final step involves printing a message to the console, conveying information about the maximum integer size on a 32-bit platform.
Output:
The maximum size of a 32-bit platform is: 2147483647
The value 2147483647
is generated as the maximum size of an integer on a 32-bit platform.
64-Bit Platform
The code snippet showcases the usage of sys.maxsize
to retrieve and display the maximum size of an integer on a 64-bit platform.
Code:
import sys
# returns the maximum size
size = sys.maxsize
print("The maximum size of a 64-bit platform is:", size)
The code snippet above is the same, only with the 32-bit code snippet. Only the result will differ based on what platform is being used in executing the script.
Output:
The maximum size of a 64-bit platform is: 9223372036854775807
The value 9223372036854775807
is generated as the maximum size of an integer on a 64-bit platform.
Example Codes: Check the Maximum Size of a List Using the sys.maxsize()
Method
To check the maximum size of our system, we can use the range()
method to pass the maximum size of a list and then check its length. Similarly, in the second example, we exceeded the maximum size, and the Python interpreter catches the exception and returns the int too large to convert to C ssize_t
error.
In the below examples, we can observe the effect of having a limit on Py_ssize_t
. It is impossible to index a list with a greater element than its size as it won’t accept a non-Py_ssize_t
.
Regarding the dictionary data structure, Py_ssize_t
uses the hash because Python does not use a LinkedList for its implementation. Similarly, we cannot have more size in the dictionary than the size of Py_ssize_t
.
With Maximum Size
The code snippet below demonstrates how to create a list with the maximum possible length on the given platform.
Code:
import sys
size = sys.maxsize
# creates the max length
list = range(size)
# returns the length of a list
print("The maximum length of a list:", len(list))
print("List is created successfully")
The code begins by importing the sys
module to access system-specific functionalities.
It then uses sys.maxsize
to determine the maximum size of an integer on the system, assigning it to the variable size
.
Subsequently, a list is created with the maximum size using the range()
function. The script proceeds to retrieve and display the length of the created list with the len()
function.
Finally, a success message is printed, confirming the successful creation of the list.
Output:
The maximum length of a list: 9223372036854775807
List is created successfully
It outputs that the list is created successfully. The maximum length of a list is 9223372036854775807
.
With Greater Than the Maximum Size
The code snippet below showcases how to handle exceptions related to list creation when the desired size exceeds the maximum limit defined by sys.maxsize
.
Code:
import sys
size = sys.maxsize
# handles the exception
try:
# creates a list with maximum size + 1
list = range(size + 1)
# check the maximum size
print(len(list))
print("List is created successfully")
# exception if the size goes beyond the maximum size
except Exception as exception:
print("Exception caught: ", exception)
print("List is not created due to above exception")
The code begins by importing the sys
module for system-specific functionalities and determines the maximum size of an integer using sys.maxsize
, assigning it to the variable size
.
The subsequent code is encapsulated in a try-except
block, preparing for potential exceptions during list creation. Within the try
block, an attempt is made to create a list exceeding the maximum size using range(size + 1)
.
If successful, the code prints the length of the list, confirming its creation.
If an exception occurs, the except
block catches it, prints an error message and indicates that the list was not created due to the encountered exception.
Output:
Exception caught: Python int too large to convert to C ssize_t
List is not created due to above exception
The error triggered is handled successfully, and prints List is not created due to above exception.
Example Codes: The sys.maxsize()
vs. sys.maxint
Methods
The sys.maxint()
method no longer supports Python 3 as integers. If we use this method or constant, we will get the following AttributeError: module 'sys' has no attribute 'maxint'
.
To overcome this in Python 3.0, another constant, sys.maxsize
, was introduced, which we know returns the maximum value of Py_ssize_t
. In Python 3, int and long int are merged.
The first implementation shows the example of AttributeError
, and the second source code reveals a better understanding of the maxint
.
Attribute Error
This code snippet addresses an issue related to the use of sys.maxint
in Python.
In Python 3 and newer versions, sys.maxint
has been deprecated, and an alternative approach is required to find the minimum value in a list.
Code:
import sys
li = [20, 2, 23, 88, 3, 63, 12]
# sys.maxint is not supported in python 3. We need to use python version < 3.0
min_value = sys.maxint
for i in range(0, len(li)):
if li[i] < min_value:
min_value = li[i]
print("Min value : " + str(min_value))
The code begins by importing the sys
module for system-specific functionalities.
It defines a list, attempting to initialize min_value
with the deprecated sys.maxint
, which is not supported in Python 3 and newer versions.
The code then iterates through the list to find the minimum value by comparing each element with the current min_value
.
Finally, the minimum value found in the list is printed, but AttributeError: module 'sys' has no attribute 'maxint'
will occur.
Output:
AttributeError: module 'sys' has no attribute 'maxint'
It encounters an issue due to the use of the deprecated sys.maxint
in Python 3 and newer versions.
To address this, an alternative method, compatible with the Python 3 syntax, is needed to ensure the code works correctly and adheres to the latest language specifications.
maxint
Implementation
The code snippet below delves into the exploration of integer sizes in Python using the sys.maxsize
attribute.
It aims to showcase the maximum representable integer, as well as the behavior when attempting to go beyond these limits.
Code:
import sys
max_int = sys.maxsize
min_int = sys.maxsize - 1
long_int = sys.maxsize + 1
print("Maximum integer size is : " + str(max_int) + " , " + str(type(max_int)))
print("Maximum integer size-1 is :" + str(max_int) + " , " + str(type(min_int)))
print("Maximum integer size+1 is :" + str(max_int) + " , " + str(type(long_int)))
The code starts by importing the sys
module for system-specific functionalities.
It defines three variables (max_int
, min_int
, and long_int
) to explore integer sizes, utilizing sys.maxsize
.
The script concludes by printing the values of these variables along with their respective data types, providing insights into the representation and limits of integers in Python.
Output:
Maximum integer size is : 9223372036854775807 , <class 'int'>
Maximum integer size-1 is :9223372036854775807 , <class 'int'>
Maximum integer size+1 is :9223372036854775807 , <class 'int'>
The maximum integer size for the max_int
, min_int
, and long_int
is 9223372036854775807
.
By exploring variations like subtracting one or adding one to this maximum value, it illustrates the behavior and limits associated with integer representations in the Python programming language.
Understanding these limits is crucial when working with large numbers or scenarios where integer overflow might be a concern.
Example Codes: Use csv.field_size_limit(sys.maxsize)
in Python
In Python, when we read the CSV file containing huge fields, it may throw an exception saying _csv.Error: field larger than field limit
. The appropriate solution would be not to skip a few fields and their lines.
To analyze the CSV, we need to increase the field_size_limit
. For this, we need to implement the following source code.
myfile.csv
:
1,2,3
import sys
import csv
maximum_Integer = sys.maxsize
while True:
try:
# Open the CSV file within the loop to avoid premature closure
with open("myfile.csv", newline="") as f:
reader = csv.reader(f)
# Iterate through rows and print them
for row in reader:
print(row)
# Attempt to set the field_size_limit
csv.field_size_limit(maximum_Integer)
# Break out of the loop if successful
break
except OverflowError:
# Reduce the size if there is an overflow error
maximum_Integer = int(maximum_Integer / 10)
The code imports the sys
and csv
modules, initializing a variable (maximum_Integer
) with the system’s maximum integer value.
It enters a while
loop to read a CSV file with potentially large fields.
Inside the loop, it dynamically adjusts the field_size_limit
based on the current maximum_Integer
, reducing it by dividing by 10 if an OverflowError
occurs.
This adaptive approach ensures the successful reading of CSV files with large fields, handling potential overflow issue.
As the output, the fields of myfile.csv
will be printed.
Conclusion
In Python, sys.maxsize
plays a crucial role in determining the maximum size of a Python integer, which is essential for handling platform-independent code and addressing potential integer overflow concerns.
This attribute, found in the sys
module, reflects the largest integer representation on the system. Utilizing it in scenarios like CSV processing showcases its significance, allowing dynamic adjustments to the field size limit.
Additionally, exploring integer sizes with sys.maxsize
unveils insights into platform-specific limitations.
The examples provide practical demonstrations, emphasizing the importance of understanding and effectively utilizing sys.maxsize
in Python programming.
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn