在 Python 中將列印輸出重定向到檔案
-
在 Python 中使用
write()
函式將輸出列印到檔案 -
在 Python 中使用
print()
函式將輸出列印到檔案 -
在 Python 中使用
sys.stdout
將輸出列印到檔案 -
在 Python 中使用
contextlib.redirect_stdout()
函式將輸出列印到檔案
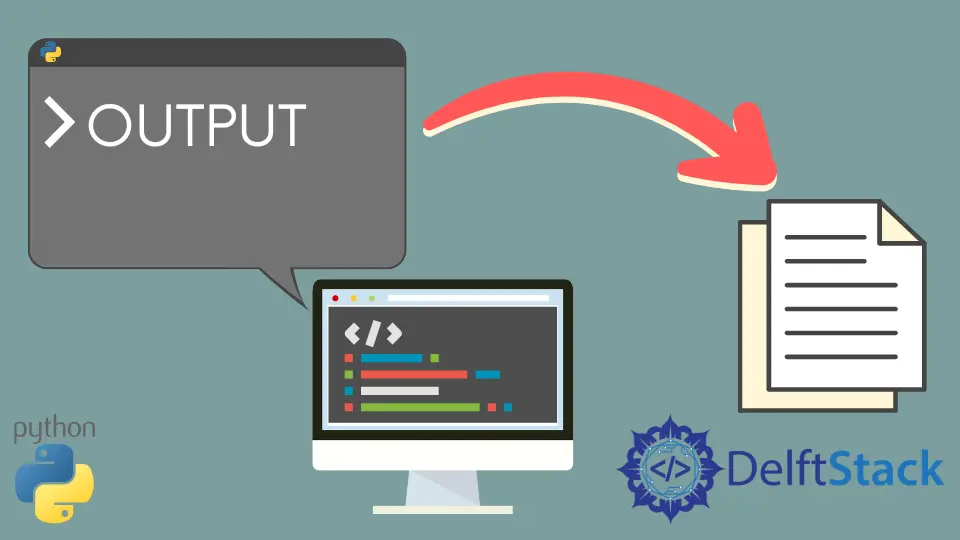
在檔案處理中還有另一種任務可以使用 python 完成,即將輸出重定向到外部檔案。基本上,標準輸出可以列印到使用者自己選擇的檔案中。有很多方法可以執行此操作。
在本教程中,我們將看到一些在 Python 中將輸出重定向到檔案的方法。
在 Python 中使用 write()
函式將輸出列印到檔案
這是一個內建的 Python 函式,可幫助將指定文字寫入或新增到檔案中。w
和 a
是此函式中的 2 個操作,它們將在檔案中寫入或新增任何文字。w
用於當使用者想在檔案中寫入任何內容之前清空檔案時使用。而當使用者只想向檔案中的現有文字新增一些文字時,使用 a
。
例子:
with open("randomfile.txt", "a") as o:
o.write("Hello")
o.write("This text will be added to the file")
請注意,此處使用 open()
函式來開啟檔案。程式碼中的 a
表示文字已新增到檔案中。
在 Python 中使用 print()
函式將輸出列印到檔案
在這個方法中,首先,我們呼叫 open()
函式來開啟所需的檔案。之後 print()
函式用於列印檔案中的文字。使用 w
運算子或 a
運算子始終是使用者的選擇。
例子:
with open("randomfile.txt", "w") as external_file:
add_text = "This text will be added to the file"
print(add_text, file=external_file)
external_file.close()
請注意,在使用 open()
開啟檔案後,close()
函式也用於關閉上述程式碼中的檔案。呼叫 close()
函式後,無法讀取檔案,也無法寫入任何其他內容。如果使用者在呼叫 close()
函式後嘗試對檔案進行任何更改,則會引發錯誤。
在 Python 中使用 sys.stdout
將輸出列印到檔案
sys
模組是一個內建的 Python 模組,使用者使用它來處理 Python 中執行時環境的各個部分。要使用 sys.stdout
,需要先匯入 sys
模組。
sys.stdout
用於當使用者希望將輸出直接顯示到螢幕的主控制檯時。輸出的形式可以是多種多樣的,例如,它可以是輸入提示、列印語句或只是一個表示式。在此方法中,我們將在文字檔案中列印一條語句。
例子:
import sys
file_path = "randomfile.txt"
sys.stdout = open(file_path, "w")
print("This text will be added to the file")
注意,在使用 sys.stdout
作為物件開啟和列印文字檔案中的語句之前,必須由使用者定義明確的檔案路徑,否則無法對檔案進行任何操作。
在 Python 中使用 contextlib.redirect_stdout()
函式將輸出列印到檔案
contextlib
模組通常與 with
語句一起使用。
contextlib.redirect_stdout()
函式通過設定上下文管理器幫助將 sys.stdout
臨時重定向到某個檔案。
例子:
import contextlib
file_path = "randomfile.txt"
with open(file_path, "w") as o:
with contextlib.redirect_stdout(o):
print("This text will be added to the file")
如你所見,with
語句與 contextlib
模組的操作一起使用。
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn