在 Python 中将打印输出重定向到文件
-
在 Python 中使用
write()
函数将输出打印到文件 -
在 Python 中使用
print()
函数将输出打印到文件 -
在 Python 中使用
sys.stdout
将输出打印到文件 -
在 Python 中使用
contextlib.redirect_stdout()
函数将输出打印到文件
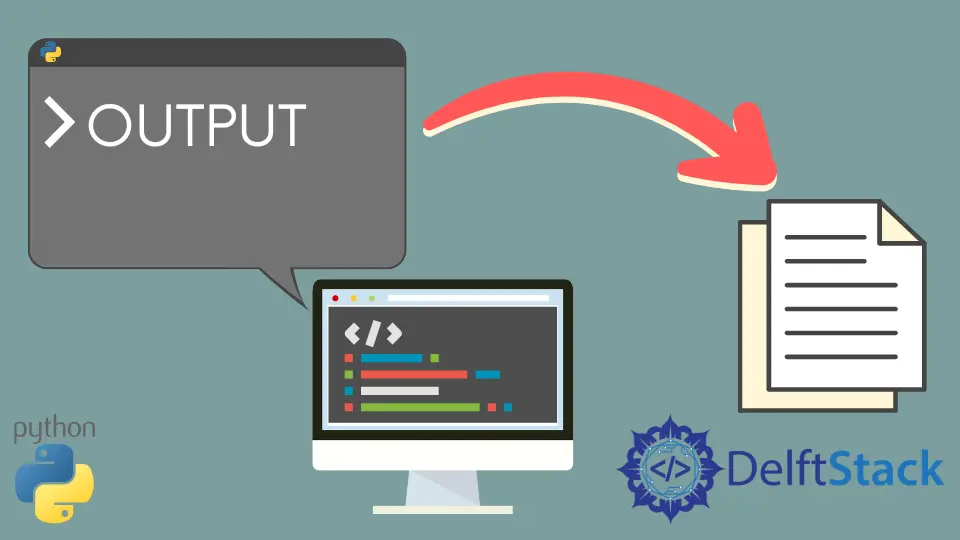
在文件处理中还有另一种任务可以使用 python 完成,即将输出重定向到外部文件。基本上,标准输出可以打印到用户自己选择的文件中。有很多方法可以执行此操作。
在本教程中,我们将看到一些在 Python 中将输出重定向到文件的方法。
在 Python 中使用 write()
函数将输出打印到文件
这是一个内置的 Python 函数,可帮助将指定文本写入或添加到文件中。w
和 a
是此函数中的 2 个操作,它们将在文件中写入或添加任何文本。w
用于当用户想在文件中写入任何内容之前清空文件时使用。而当用户只想向文件中的现有文本添加一些文本时,使用 a
。
例子:
with open("randomfile.txt", "a") as o:
o.write("Hello")
o.write("This text will be added to the file")
请注意,此处使用 open()
函数来打开文件。代码中的 a
表示文本已添加到文件中。
在 Python 中使用 print()
函数将输出打印到文件
在这个方法中,首先,我们调用 open()
函数来打开所需的文件。之后 print()
函数用于打印文件中的文本。使用 w
运算符或 a
运算符始终是用户的选择。
例子:
with open("randomfile.txt", "w") as external_file:
add_text = "This text will be added to the file"
print(add_text, file=external_file)
external_file.close()
请注意,在使用 open()
打开文件后,close()
函数也用于关闭上述代码中的文件。调用 close()
函数后,无法读取文件,也无法写入任何其他内容。如果用户在调用 close()
函数后尝试对文件进行任何更改,则会引发错误。
在 Python 中使用 sys.stdout
将输出打印到文件
sys
模块是一个内置的 Python 模块,用户使用它来处理 Python 中运行时环境的各个部分。要使用 sys.stdout
,需要先导入 sys
模块。
sys.stdout
用于当用户希望将输出直接显示到屏幕的主控制台时。输出的形式可以是多种多样的,例如,它可以是输入提示、打印语句或只是一个表达式。在此方法中,我们将在文本文件中打印一条语句。
例子:
import sys
file_path = "randomfile.txt"
sys.stdout = open(file_path, "w")
print("This text will be added to the file")
注意,在使用 sys.stdout
作为对象打开和打印文本文件中的语句之前,必须由用户定义明确的文件路径,否则无法对文件进行任何操作。
在 Python 中使用 contextlib.redirect_stdout()
函数将输出打印到文件
contextlib
模块通常与 with
语句一起使用。
contextlib.redirect_stdout()
函数通过设置上下文管理器帮助将 sys.stdout
临时重定向到某个文件。
例子:
import contextlib
file_path = "randomfile.txt"
with open(file_path, "w") as o:
with contextlib.redirect_stdout(o):
print("This text will be added to the file")
如你所见,with
语句与 contextlib
模块的操作一起使用。
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn