How to Print Subscripts to the Console Window in Python
- Print Subscripts to the Console Window Using the Unicode Method in Python
-
Print Subscripts to the Console Window Using the
\N{}
Escape Sequence in Python
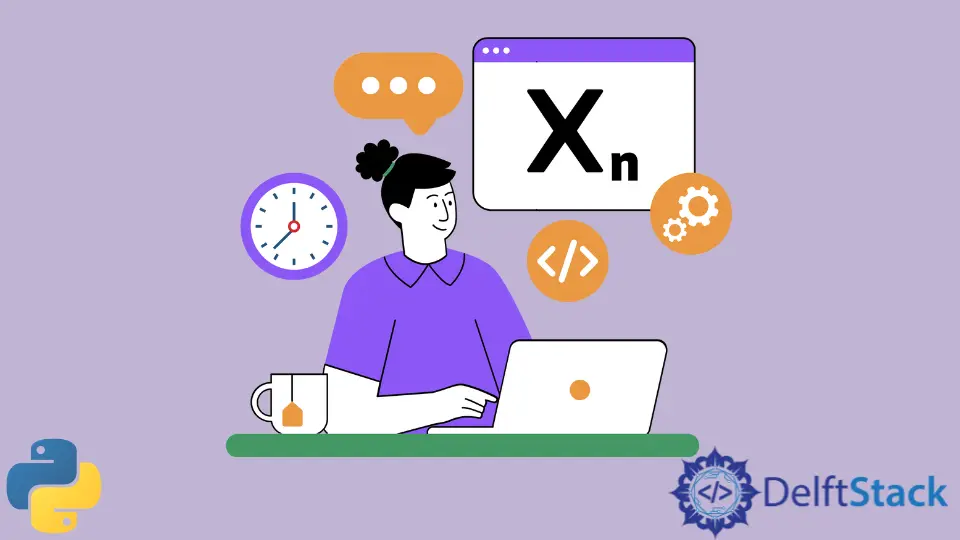
This tutorial will discuss how to print subscripts to the console in Python.
Print Subscripts to the Console Window Using the Unicode Method in Python
There is no direct way to print subscripts to the console in Python. We need to refer to this link to see the Unicode representations of the characters we want to put in the subscript or superscript notation.
We then write that representation inside our print()
function with the \u
escape character to tell the interpreter we are writing in Unicode notation.
The Unicode representation of numbers starts with \u208
followed by the desired number, and the Unicode representation of alphabets starts with \u209
followed by the index of that alphabet.
The following code displays the implementation of this solution in Python.
print(u"H\u2082SO\u2084")
print("x\u2091")
Output:
H₂SO₄
xₑ
We printed subscript numbers in the first line with \u2082
and \u2084
; and subscript alphabet in the second line with \u2091
. The first line’s output is self-explanatory because the last number in the sequence gets printed in the subscript each time.
In the second line, 1 is the last character of the sequence, whereas we get e
as the subscript. It is because e
has index 1 in this Unicode representation.
Print Subscripts to the Console Window Using the \N{}
Escape Sequence in Python
If remembering the index of every character and symbol is getting difficult, we can use this approach to ease our difficulty and make the code a little more readable.
We need to remember the aliases of the symbols we want to print for this method to work. This link provides a handy guide to all the aliases in Unicode.
We can write these aliases inside the \N{}
escape sequence to print them to the console.
The following code example demonstrates a working implementation of this method in Python.
print("CO\N{subscript two}")
print("C\N{LATIN SUBSCRIPT SMALL LETTER N}")
Output:
CO₂
Cₙ
We printed a number in the subscript notation to the console in the first line. We just specified the Unicode name subscript two
in the \N{}
escape sequence.
This Unicode name is case-insensitive, which means we can use either uppercase or lowercase Unicode names.
We printed a letter in the subscript notation to the console in the second line. We used the Unicode name LATIN SUBSCRIPT SMALL LETTER N
inside the \N{}
escape sequence.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn