How to Print % Sign in Python
-
Print
%
Sign in Python -
Print
%
Sign With Escape Characters in Python -
Print
%
Sign With thestr.format()
Function in Python
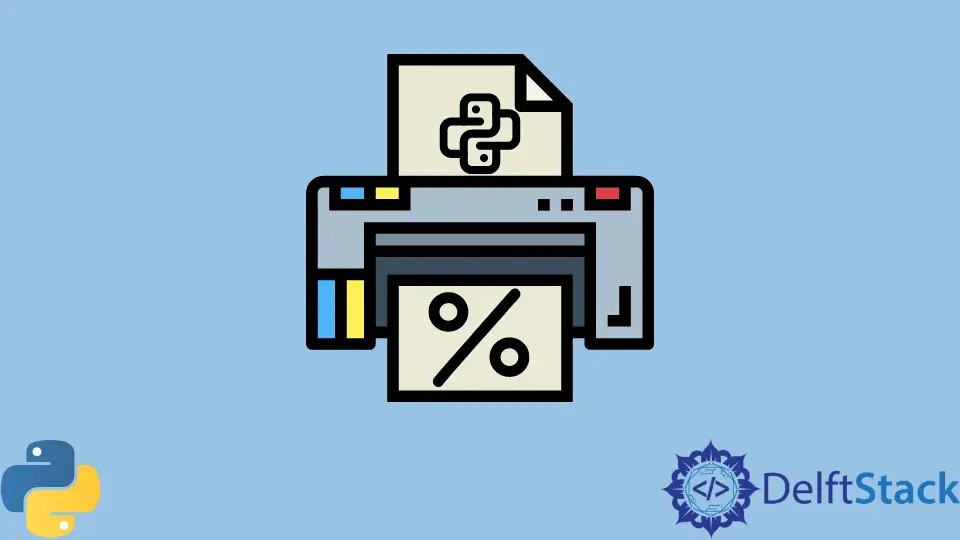
This tutorial will introduce the methods to print the %
sign with Python’s print()
function.
Print %
Sign in Python
Normally, as shown in the following code snippet, we don’t need any special tricks to write the %
sign to the console with the print()
function in Python.
print("This is the % sign")
Output:
This is the % sign
We printed the %
sign to console with Python’s print()
function. The issue occurs when we write a string with the %s
placeholder and another %
sign in the same print()
function. The following code runs without an error.
text = "some text"
print("this is %s" % text)
Output:
this is some text
But, when we try to combine the above two codes, the following resultant code snippet gives us an error.
text = "some text"
print("this is a % sign with %s" % text)
Error:
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-4-6006ad4bf52e> in <module>()
1 text = "some text"
----> 2 print("this is a % sign with %s"%text)
TypeError: not enough arguments for format string
This error is thrown because we haven’t used any escape character for writing the %
sign.
Print %
Sign With Escape Characters in Python
In this case, our escape character is also a %
character instead of a \
character. The following code snippet shows us how to escape a %
sign with another %
escape character.
text = "some text"
print("this is a %% sign with %s" % text)
Output:
this is a % sign with some text
We printed both %
sign and a string variable with the print()
function and %
escape character in the above code.
Print %
Sign With the str.format()
Function in Python
We can also use string formatting to print a %
sign with a string variable inside a single print()
function. The str.format()
function can be used for this purpose. The str
is a string that contains our output with {}
in place of string variables, and the format()
function contains all the string variables that we want to print. The following code snippet shows how to print the %
sign with string formatting in Python.
text = "some text"
print("this is a % sign with {0}".format(text))
Output:
this is a % sign with some text
In the above code, we used str.format()
function to write a %
sign with a string variable inside a single print()
function in Python.
This method is preferable because the previous method has been deprecated and will soon become obsolete.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn