How to Print Quotes in Python
- Print Quotes in Python Using the Escape Character
- Print Quotes in Python Using Single Quotes to Enclose Double Quotes
- Print Quotes in Python Using Double Quotes to Enclose Single Quotes
- Print Multiline Quotes in Python Using Triple Single Quotes
- Print Multiline Quotes in Python Using Triple Double Quotes
- Print Double Quotes With String Variables in Python
- Conclusion
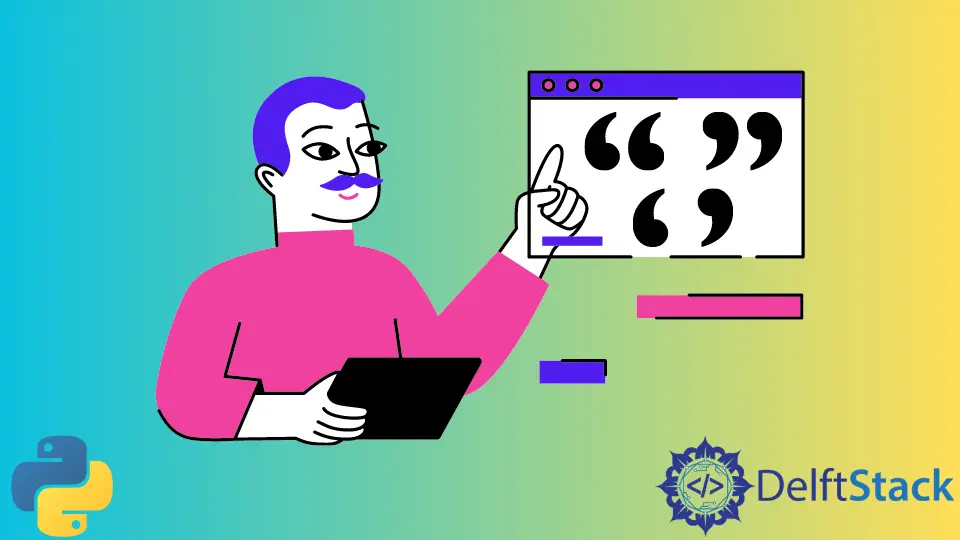
Handling quotes within Python strings is an essential skill for developers, especially when dealing with dynamic content or multiline expressions.
This article delves into practical applications, demonstrating how to use escape characters, leverage different types of quotes, and employ triple quotes for multiline expressions.
Print Quotes in Python Using the Escape Character
In Python, the escape character (\
) is used to include special characters within strings. When encountered in a string, it signals that the character following it should be treated differently.
This becomes particularly useful when working with quotes that contain characters such as single quotes ('
) or double quotes ("
).
Using the Escape Character for Single Quotes
Consider the following scenario where a quote contains a single quote:
quote_with_single_quote = (
"It's not whether you get knocked down, it's whether you get up."
)
print(quote_with_single_quote)
Output:
It's not whether you get knocked down, it's whether you get up.
In this example, the backslash (\
) before each single quote signals to Python that the following single quote is part of the string, not its closing delimiter. This allows the entire quote to be printed correctly.
Using the Escape Character for Double Quotes
Similarly, the escape character can be employed when dealing with quotes that contain double quotes:
quote_with_double_quote = (
'She said, "The journey of a thousand miles begins with a single step."'
)
print(quote_with_double_quote)
Output:
She said, "The journey of a thousand miles begins with a single step."
Here, the backslash before each double quote informs Python to treat them as literal characters within the string, preventing any interference with the string’s boundaries.
Print Quotes in Python Using Single Quotes to Enclose Double Quotes
In Python, we can define strings using either single quotes ('
) or double quotes ("
). This flexibility allows developers to strategically use one type of quote to encapsulate strings containing the other.
Let’s delve into the practical application of using single quotes to enclose double quotes.
Consider the scenario where a quote itself contains double quotes:
quote_with_double_quote = (
'She said, "The journey of a thousand miles begins with a single step."'
)
print(quote_with_double_quote)
Output:
She said, "The journey of a thousand miles begins with a single step."
Here, the entire quote is enclosed within single quotes, allowing the use of double quotes within the string without conflicting with the string’s boundaries.
Handling Single Quotes
This approach becomes particularly useful when the quote also includes single quotes:
quote_with_single_and_double_quotes = (
"He exclaimed, \"It's not about the destination, it's about the journey.\""
)
print(quote_with_single_and_double_quotes)
Output:
He exclaimed, "It's not about the destination, it's about the journey."
By utilizing a backslash (\
) as an escape character, single quotes within the quote are handled seamlessly.
Multiline Quotes
For multiline quotes that contain double quotes, the single quotes method remains concise:
multiline_quote = '"Success is not final,\nfailure is not fatal:\nIt is the courage to continue that counts."'
print(multiline_quote)
Output:
"Success is not final,
failure is not fatal:
It is the courage to continue that counts."
The simplicity of using single quotes makes the code readable, especially when dealing with multiline quotes.
Print Quotes in Python Using Double Quotes to Enclose Single Quotes
Now, let’s delve into the practical application of using double quotes to enclose single quotes within a string.
Consider the following scenario where a quote itself contains a single quote:
quote_with_single_quote = (
"He said, 'The only way to do great work is to love what you do.'"
)
print(quote_with_single_quote)
Output:
He said, 'The only way to do great work is to love what you do.'
Here, the entire quote is enclosed within double quotes, allowing the use of single quotes within the string without conflicting with the string’s boundaries.
Handling Double Quotes
This approach becomes particularly useful when the quote also includes double quotes:
quote_with_double_and_single_quotes = (
"She remarked, 'Life's too short to be anything but happy.'"
)
print(quote_with_double_and_single_quotes)
Output:
She remarked, 'Life's too short to be anything but happy.'
By utilizing escape characters or without them, double quotes within the quote are handled seamlessly.
Multiline Quotes
For multiline quotes that contain single quotes, the double quotes method remains concise:
multiline_quote = "'In the middle of difficulty lies opportunity.'\n"
multiline_quote += "'You must be the change you wish to see in the world.'"
print(multiline_quote)
Output:
'In the middle of difficulty lies opportunity.'
'You must be the change you wish to see in the world.'
The simplicity of using double quotes makes the code readable, especially when dealing with multiline quotes.
Print Multiline Quotes in Python Using Triple Single Quotes
Triple single quotes in Python are primarily employed for defining multiline strings. This approach not only simplifies the code but also enhances readability, making it particularly useful when working with lengthy quotes or preserving line breaks.
Consider a basic example of a quote using triple single quotes:
basic_multiline_quote = '''"Success is not final,
failure is not fatal:
It is the courage to continue that counts."'''
print(basic_multiline_quote)
Output:
"Success is not final,
failure is not fatal:
It is the courage to continue that counts."
In this example, the entire quote is enclosed within triple single quotes, allowing the preservation of line breaks and providing a visually appealing output.
Handling Single Quotes
Triple single quotes gracefully handle quotes that contain single quotes:
quote_with_single_quote = (
"""The 'greatest' glory in living lies not in never falling,"""
)
quote_with_single_quote += """ but in rising every time we fall."""
print(quote_with_single_quote)
Output:
The 'greatest' glory in living lies not in never falling, but in rising every time we fall.
By utilizing triple single quotes, the code remains concise and readable even when the quote spans multiple lines.
Mixing Single and Double Quotes
When a quote contains both single and double quotes, the triple single quotes method still maintains readability:
mixed_quotes_quote = """He exclaimed, "To be yourself in a world that's constantly trying to make you something else"""
mixed_quotes_quote += ''' is the greatest accomplishment."'''
print(mixed_quotes_quote)
Output:
He exclaimed, "To be yourself in a world that's constantly trying to make you something else is the greatest accomplishment."
Print Multiline Quotes in Python Using Triple Double Quotes
Triple double quotes in Python are a powerful tool for creating multiline strings. This technique simplifies code and enhances readability, making it particularly useful for quotes with multiple lines or containing both single and double quotes.
Consider a basic example of a quote using triple-double quotes:
basic_multiline_quote = """Success is not final,
failure is not fatal:
It is the courage to continue that counts."""
print(basic_multiline_quote)
Output:
Success is not final,
failure is not fatal:
It is the courage to continue that counts.
In this example, the entire quote is enclosed within triple-double quotes, allowing the preservation of line breaks and providing a visually appealing output.
Handling Single Quotes
Triple double quotes gracefully handle quotes that contain single quotes:
quote_with_single_quote = (
"""She said, 'The greatest glory in living lies not in never falling,"""
)
quote_with_single_quote += """ but in rising every time we fall.'"""
print(quote_with_single_quote)
Output:
She said, 'The greatest glory in living lies not in never falling, but in rising every time we fall.'
By utilizing triple-double quotes, the code remains concise and readable even when the quote spans multiple lines.
Mixing Single and Double Quotes
When a quote contains both single and double quotes, the triple-double quotes method still maintains readability:
mixed_quotes_quote = """He exclaimed, "To be yourself in a world that is constantly trying to make you something else"""
mixed_quotes_quote += """ is the greatest accomplishment.\""""
print(mixed_quotes_quote)
Output:
He exclaimed, "To be yourself in a world that is constantly trying to make you something else is the greatest accomplishment."
Print Double Quotes With String Variables in Python
Printing quotes dynamically in Python often involves incorporating variables within the quote. However, when the quote itself contains double quotes, it introduces a challenge, as using double quotes to enclose the string variable may lead to syntax errors.
Consider the following example, where a string variable is used within a quote containing double quotes:
dynamic_quote_variable = "Imagination"
quote_with_variable = f"{dynamic_quote_variable} is more important than knowledge."
print(quote_with_variable)
Output:
Imagination is more important than knowledge.
In this example, an f-string
is utilized to incorporate the value of dynamic_quote_variable
within the quote. However, if the quote itself contained double quotes, using them to enclose the entire string would result in a syntax error.
Escaping Double Quotes
To handle quotes containing double quotes, we can use escape characters within the string:
quote_with_double_quotes = 'He said, "Imagination is more important than knowledge."'
print(quote_with_double_quotes)
Output:
He said, "Imagination is more important than knowledge."
While this method works, it can become cumbersome when dealing with complex quotes or dynamic content.
Using Single Quotes to Enclose Double Quotes
An alternative approach is to use single quotes to enclose the entire string, allowing double quotes within the quote itself:
dynamic_quote_variable = "Imagination"
quote_with_variable = (
f'{dynamic_quote_variable} said, "Imagination is more important than knowledge."'
)
print(quote_with_variable)
Output:
Imagination said, "Imagination is more important than knowledge."
By using single quotes for the outer string, you can seamlessly include double quotes in the quote itself.
Conclusion
In Python, mastering the art of quotes within strings is crucial for code clarity and flexibility. Whether employing escape characters, choosing between single and double quotes, or embracing triple quotes for multiline elegance, Python provides a robust toolkit.
By incorporating these practices, you can ensure readability and adaptability in their code, allowing them to express quotes seamlessly and handle diverse quotation scenarios effectively.