How to Remove Last Character From String in JavaScript
-
Use the
substring()
Function to Remove the Last Character From a JavaScript String -
Use the
slice()
Method to Remove the Last Character From a JavaScript String -
Use the
replace()
Method to Remove the Last Character From a JavaScript String
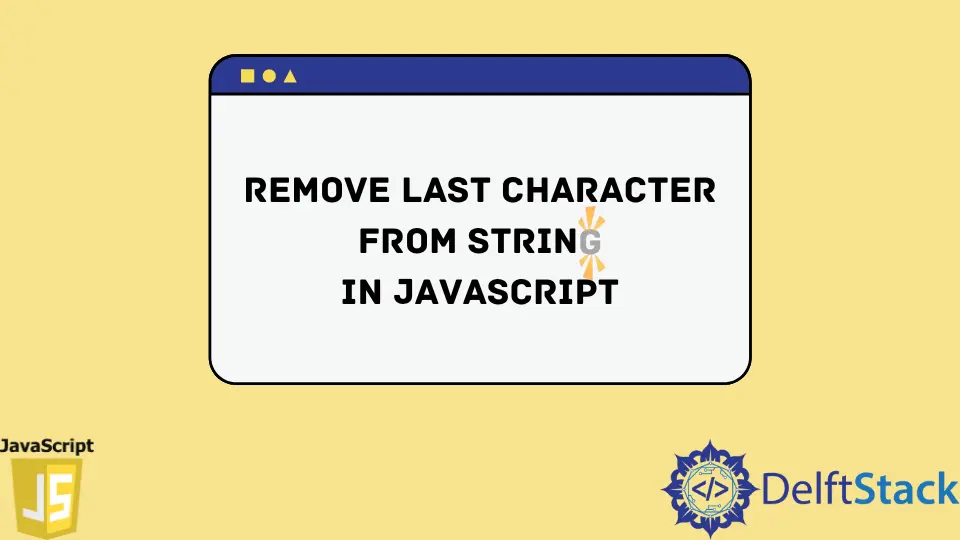
There are situations where we have to trim/chop/remove a character from a string. Since a string in JavaScript is immutable, we can not modify an existing string, but we have to create a new string with the required changes. We can remove the last character using various methods such as regular expression, getting the substring excluding the last character, etc. We will see different ways to remove the last character from a JavaScript string.
Use the substring()
Function to Remove the Last Character From a JavaScript String
We use the substring()
method to return the part of a string between two indexes. If only one index is provided, it is treated as the starting index, and the function returns the substring from starting index to the last index of the string. To remove only the last character from the string, we have to provide the ending index as len - 1
where len
is the length of the string. Since len-1
is the last index of the string, we want the string before the character at that index.
const str = 'DelftStacks';
const str2 = str.substring(0, str.length - 1);
console.log(str2);
Output:
DelftStack
A function similar to substring()
is substr()
; it also takes two arguments, but the second argument in the case of substr()
is the length of the substring. We can use it to achieve the same results by the following code.
const str = 'DelftStacks';
const str2 = str.substr(0, str.length - 1);
console.log(str2);
Output:
DelftStack
Although it is equivalent to the substring
method, it is still avoided because it is considered a legacy method, and it is much more preferable to use the substring()
method.
Use the slice()
Method to Remove the Last Character From a JavaScript String
The slice()
method works pretty similarly to the substring()
method. It also takes the starting index start_index
and ending index end_index
as arguments. The significant difference is that we can use negative indexes as arguments in the function. A negative index is brought to the normal range by adding the value str.length
. We can chop off the last character by passing 0
as starting index and -1
as the ending index.
const str = 'DelftStacks';
const str2 = str.slice(0, -1);
console.log(str2);
Output:
DelftStack
Use the replace()
Method to Remove the Last Character From a JavaScript String
We can also remove the last character from a string by using the replace()
function. It takes a regular expression as an input and the replacement for the results of the regular expression. $
character is used to match the end of the input. The .
is used to match a single character. Thus, the regular expression /.$/
can be used to get the last character from a string. After this, we replace it with ''
i.e. blank, to remove it from the string.
const str = 'DelftStacks';
const str2 = str.replace(/.$/, '');
console.log(str2);
Output:
DelftStack
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn