How to Check if Array Contains Value in JavaScript
-
Using the
.indexOf()
Function to Check if Array Contains Value in JavaScript -
Using the
.includes()
Function to Check if Array Contains Value in JavaScript -
Using the
.find()
Function to Find Element in an Array in JavaScript -
Using the
.filter()
Function to Search Elemnt in an Array in JavaScript -
Using Simple
for
Loop to Search for an Element - Note
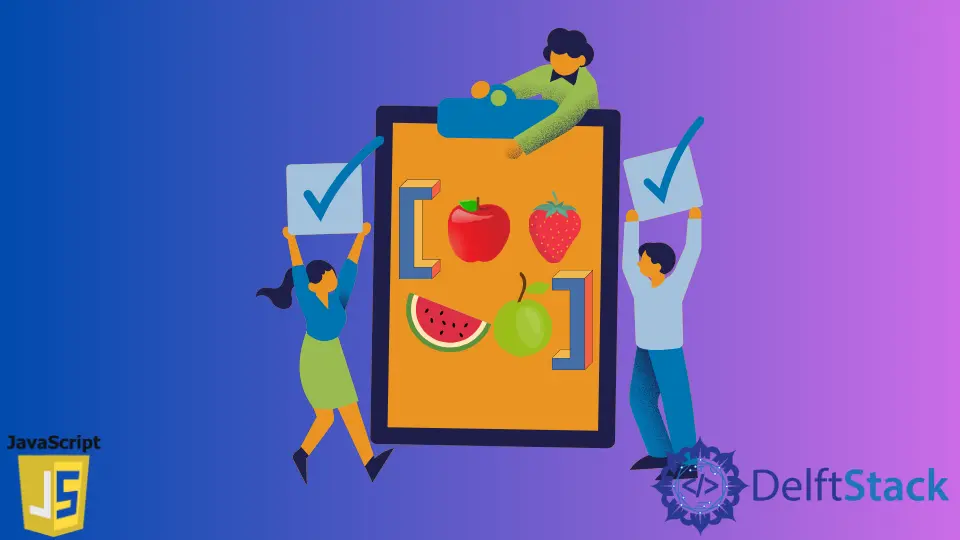
Often we find ourselves in a situation to check if array contains value in JavaScript. Even though the search functionality can be coded with basic JavaScript syntaxes using loops, it is good to know the inbuilt functionalities available for array search in JavaScript, their usage, return types and the behaviour. It makes our task much easier, as we need to code less. The functionality will be efficient and less bug-prone with in-built functions. Let us look at ways to search for an element in an array.
Using the .indexOf()
Function to Check if Array Contains Value in JavaScript
The .indexOf()
function is a commonly used function in javascript. It works well while searching for an element in an array or even a string.
Syntax
indexOf(element, index)
Parameter
It takes the element, to search, as a parameter. And an optional parameter that tells the function, the index from where the search must be started (starting from 0
). If we provide a negative value, it will mean to search starting from the end of the array at the specified position.
Return Value
The function returns the index of the search element starting from 0
in the array if it could find the element. Else, it will return -1
, indicating that it could not find the element. If there are more than one matches in an array, then the .indexOf()
function will return the index of the first match.
Browser Support
The .indexOf()
function is supported for all major browser versions.
Usage
The usage of the indexOf()
function on an array is as follows.
let fruits = ['Apple', 'Mango', 'Pear', 'Peach'];
console.log(fruits.indexOf('Pear'));
console.log(fruits.indexOf('Tomato'))
Output:
2
-1
For the search feature, we check if the return type of the .indexOf(searchText)
is greater than -1
. If so, then the search result should be true
else false
. Let us look at the same example discussed above to check if an element exists in the array.
function isFruit(fruitName) {
let fruits = ['Apple', 'Mango', 'Pear', 'Peach'];
if (fruits.indexOf(fruitName) > -1) {
return true;
} else {
return false;
}
}
isFruit('Pear');
isFruit('Cat');
Output:
true
false
Using the .includes()
Function to Check if Array Contains Value in JavaScript
The includes()
function of JavaScript checks whether a given element is present in an array. It returns a boolean value. Hence, it is best suited in if
condition checks.
Syntax
includes(keyword)
includes(keyword, index)
Parameter
The function takes two parameters. Usually, we use this function in the form .includes(searchString)
. The searchString
parameter is the element we wish to search, and the index
parameter refers to the array index starting from which to search. The index
parameter is optional.
Return Value
.includes()
returns true
if the searchString
is found as an element of the array. If not found, the function returns false.
Browser Support
The .includes()
function works in almost all web browsers except Internet Explorer. Hence, if your project is supported on IE, you may have to go for the indexOf()
function.
Usage
Let us look at applying the includes()
in an array.
let fruits = ['Apple', 'Mango', 'Pear', 'Peach'];
console.log(fruits.includes('Pear'));
console.log(fruits.includes('Tomato'))
Output:
true
false
The includes()
function can be directly used in if
conditions as it returns a boolean value as output. The above example can be used in conditional if
as follows. Just be aware as this code may not work in IE as .includes()
is not supported in it.
function isFruit(fruitName) {
let fruits = ['Apple', 'Mango', 'Pear', 'Peach'];
if (fruits.includes(fruitName)) {
return true;
} else {
return false;
}
}
isFruit('Pear');
isFruit('Cat');
Output:
true
false
Using the .find()
Function to Find Element in an Array in JavaScript
Another way to check if an element is present in an array is by using the .find()
function. As compared to the .indexOf()
and .includes()
functions, .find()
takes a function as a parameter and executes it on each element of the array. It will return the first value which is satisfied by the condition as mentioned in the parameter function.
Syntax
.find((keyword) => {/* Some condition checks */})
.find((keyword, index) => {/* Some condition checks */})
Parameter
The Array.find()
function takes a function as its input parameter. Usually, we use arrow functions as parameters. And these arrow functions may contain condition checks like item => item > 21
. Another optional parameter is the index
that represents the current iteration index. The .find()
executes the arrow function on each element of the array. Hence, it provides the iterator as the argument to the arrow function for helping with further conditional checks.
Return Value
.find()
is a bit different from the indexOf()
, includes()
functions of javascript. It returns the value of the element if found, else the function returns undefined.
Browser Support
Like the .includes()
function, .find()
works in almost all web browsers except internet explorer. Hence, we should be sure that our project is not supported for IE before planning to use such functions for Array operations.
Usage
Refer to the following usage in an array.
let age = [21, 43, 23, 1, 34, 12, 8];
console.log(age.find(i => i > 20));
console.log(age.find(i => i > 21))
Output:
21
43
We may be a bit surprised by the output of age.find(i => i > 21)
, as it returns 43
instead of 23
which was the next higher number to 21
in the age
array. Note that the .find()
function returns the next element that satisfies the condition mentioned in the array function in chronological order. To search an element with an if
block, we can use the following code.
function findPeerAge(myAge) {
let age = [21, 43, 23, 1, 34, 12, 8];
if (age.find(i => (i === myAge))) {
return true;
} else {
return false;
}
}
findPeerAge(23);
findPeerAge(40);
true
false
Using the .filter()
Function to Search Elemnt in an Array in JavaScript
The .filter()
function is mainly used in array processing operations. Especially when it comes to filtering elements, satisfying a criteria, out of an array. It returns an array of elements satisfying the criteria defined in the function passed as an argument.
Syntax
Follows same syntax as Array.find()
.
.filter((keyword) => {/* Some condition checks */})
.filter((keyword, index) => {/* Some condition checks */})
Parameter
The Array.filter()
function accepts an inline function or arrow function as a parameter. The arrow function will be holding the filter criteria. The .filter()
function executes the arrow function on each element of the array and returns the elements that satisfy the conditions mention ins the arrow function. The arrow function will have the keyword
as input and the current iteration as index
.
Return Value
Unlike the array search functions we have seen so far, the .filter()
returns an array containing the elements satisfying the conditions mentioned in the arrow function. If element is found, the returned array will contain the required elements, else an empty array will be returned by the function.
Browser Support
The .filter()
function is supported by all the browsers even including the Internet Explorer. So you can use .filter()
function without bothering for the expected browser support.
Usage: The Usage of This Function Is as Follows
let age = [21, 43, 23, 1, 34, 12, 8];
console.log(age.filter(i => i > 20));
console.log(age.filter(i => i > 21));
Output:
[21, 43, 23, 34]
[43, 23, 34]
As we see from the result, the filter()
function returns an array. If we have to search for an element in an array, we can use the filter()
function as depicted in the following code sample. We use the .length
attribute of the array returned by the filter()
function to determine if it could find the element or not. If the function returns an empty array, it signifies it could not find the search keyword.
function findAge(myAge) {
let age = [21, 43, 23, 1, 34, 12, 8];
if (age.filter(i => (i === myAge)).length) {
return true;
} else {
return false;
}
}
findAge(23);
findAge(40);
Output:
true
false
Using Simple for
Loop to Search for an Element
We can find an element in an array with the basic methods of javascript, the if
condition and the for
loop. The following code will return true if the element is found, else will return false. If there are more than one occurances of the same element, the function returns only the first match.
function findAge(myAge, ageAry) {
let elementFound = false;
for (let i = 0; i < ageAry.length; i++) {
if (ageAry[i] === myAge) {
return true;
}
}
return elementFound;
}
let srcArray = [21, 43, 23, 1, 34, 12, 8];
findAge(23, srcArray);
findAge(40, srcArray);
findAge(8, srcArray);
findAge(23, srcArray);
Output:
true
false
true
true
Note
.indexOf()
,.includes()
functions look for an exact element match. We cannot use it for searching partial strings. It means providing part of an element as the search parameter will not work.- We can implement partial element search with the
Array.filter()
and theArray.find()
functions as they allow us to custom define the search criteria.