How to Convert Arguments to an Array in JavaScript
-
What Is the
arguments
Object in JavaScript -
Convert the
arguments
Object to an Array Usingrest
Parameters in JavaScript -
Convert the
arguments
Object to an Array UsingArray.from()
Method in JavaScript -
Convert the
arguments
Object to an Array UsingArray literals
in JavaScript
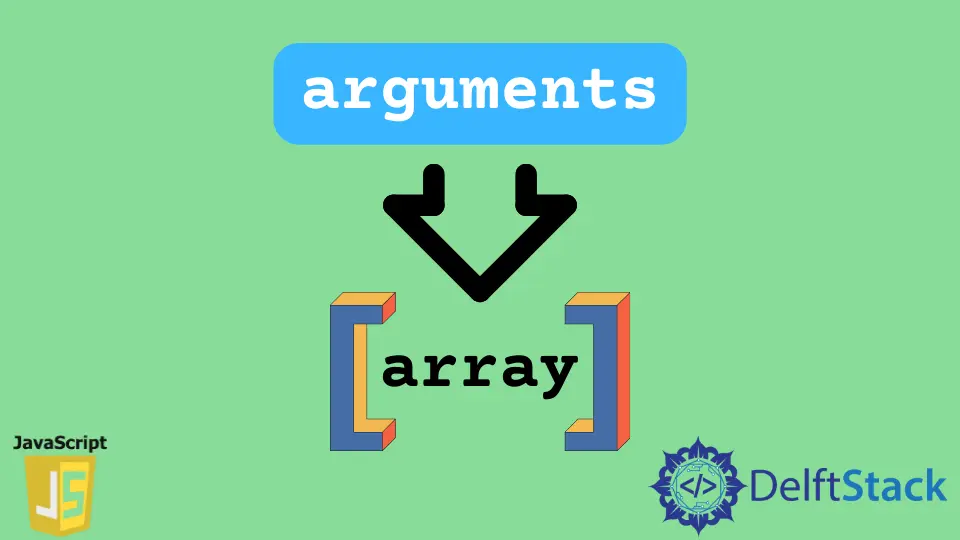
What Is the arguments
Object in JavaScript
The arguments
is an object which is present inside every function. Whatever parameters you pass to a function in JavaScript, those parameters are stored inside this arguments list in the form of an object. The keys of this object are numbers starting from zero. To access the values present inside the arguments
object, we can use these keys.
This arguments
object can be used as an array, but it doesn’t support the JavaScript functions like forEach
, sort
, filter
, and map
. So, if you want to use these functions with the arguments object, you must convert this entire object into an array.
The arguments
object can be converted into an array in many different ways. We will focus on the most popular ways like using the rest
parameters, array.from()
and array literals
. Let’s discuss each of them in detail.
Convert the arguments
Object to an Array Using rest
Parameters in JavaScript
The rest
parameter allows a function to accept a variable number of arguments as an input. It is represented by ...args
. Here, in place of args
you can give any name, and the triple dots ...
is known as the spread operator.
Now, whenever you pass ...args
as a parameter to a function, this will convert the arguments object into an array, and you can now access the arguments object using the name args
.
function func(...args) {
console.log(args);
console.log(args[0]);
}
func(1, 2, 3);
Output:
[ 1, 2, 3 ]
1
In this example, we pass the parameters 1,2,3
as an argument to a function func()
. Since we have used the rest
operator, we will get the arguments
object in the form of an array. And we can now use various methods like sort or filter on this array.
Convert the arguments
Object to an Array Using Array.from()
Method in JavaScript
Another way of converting the arguments object into an array is to use the method Array.from()
. Here, we have to pass the arguments object inside the from()
method, giving us an array. You can either store the resulting array into a variable or even directly access the elements inside this array.
function func() {
console.log(Array.from(arguments));
console.log(Array.from(arguments)[0]);
}
func(1, 2, 3);
Output:
[ 1, 2, 3 ]
1
We have also taken the same example, but the only difference is that we are using Array.from()
and passing in the arguments
object instead of using a rest
operator. You can use array indexing such as Array.from(arguments)[0]
to access the individual elements. This will return the first element of the array.
Convert the arguments
Object to an Array Using Array literals
in JavaScript
An array literals
is a list that consists of zero or more elements that start with the index number zero. In array literals
the elements are enclosed inside the square brackets []
. Here, every element can be accessed with the help of its index number.
function func() {
let args = [].slice.call(arguments);
console.log(args);
console.log(args[2]);
}
func(1, 2, 3);
Output:
[ 1, 2, 3 ]
3
To convert the arguments object into an array, we first have taken an empty array. On that array, we call the slice()
method using the call()
method. Now the slice()
method will iterate over the object which is passed. In this case, it’s the arguments
object, and then it will append all the elements inside the object into the empty array. In the end, we will store the result of this inside a variable, args
.
Since this variable now contains all the elements which the arguments
object used to contain, you can access every element with its appropriate index number using the args
variable.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn