在 JavaScript 中将参数转换为数组
-
什么是 JavaScript 中的
arguments
对象 -
在 JavaScript 中使用
rest
参数将arguments
对象转换为数组 -
在 JavaScript 中使用
Array.from()
方法将arguments
对象转换为数组 -
在 JavaScript 中使用
数组文字
将arguments
对象转换为数组
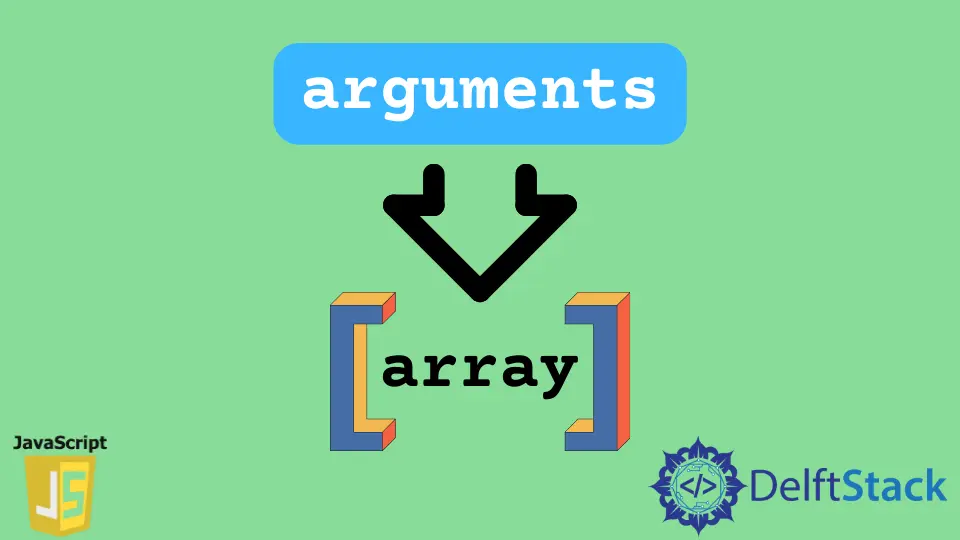
什么是 JavaScript 中的 arguments
对象
arguments
是一个存在于每个函数中的对象。无论你在 JavaScript 中传递给函数的什么参数,这些参数都以对象的形式存储在此参数列表中。这个对象的键是从零开始的数字。要访问 arguments
对象中存在的值,我们可以使用这些键。
这个 arguments
对象可以用作数组,但它不支持 JavaScript 函数,如 forEach
、sort
、filter
和 map
。因此,如果你想将这些函数与参数对象一起使用,你必须将整个对象转换为一个数组。
arguments
对象可以通过多种不同的方式转换为数组。我们将关注最流行的方法,例如使用 rest
参数、array.from()
和 array literals
。让我们详细讨论它们中的每一个。
在 JavaScript 中使用 rest
参数将 arguments
对象转换为数组
rest
参数允许函数接受可变数量的参数作为输入。它由 ...args
表示。在这里,代替 args
,你可以给出任何名称,三个点 ...
被称为扩展运算符。
现在,每当你将 ...args
作为参数传递给函数时,这会将参数对象转换为数组,你现在可以使用名称 args
访问参数对象。
function func(...args) {
console.log(args);
console.log(args[0]);
}
func(1, 2, 3);
输出:
[ 1, 2, 3 ]
1
在这个例子中,我们将参数 1,2,3
作为参数传递给函数 func()
。由于我们使用了 rest
运算符,我们将以数组的形式获得 arguments
对象。我们现在可以在这个数组上使用各种方法,比如排序或过滤。
在 JavaScript 中使用 Array.from()
方法将 arguments
对象转换为数组
另一种将参数对象转换为数组的方法是使用方法 Array.from()
。在这里,我们必须在 from()
方法中传递参数对象,从而为我们提供一个数组。你可以将结果数组存储到变量中,甚至可以直接访问该数组中的元素。
function func() {
console.log(Array.from(arguments));
console.log(Array.from(arguments)[0]);
}
func(1, 2, 3);
输出:
[ 1, 2, 3 ]
1
我们也采用了相同的示例,但唯一的区别是我们使用 Array.from()
并传入 arguments
对象而不是使用 rest
运算符。你可以使用诸如 Array.from(arguments)[0]
之类的数组索引来访问各个元素。这将返回数组的第一个元素。
在 JavaScript 中使用数组文字
将 arguments
对象转换为数组
array literals
是一个由零个或多个以索引号零开头的元素组成的列表。在 array literals
中,元素包含在方括号 []
内。在这里,每个元素都可以借助其索引号来访问。
function func() {
let args = [].slice.call(arguments);
console.log(args);
console.log(args[2]);
}
func(1, 2, 3);
输出:
[ 1, 2, 3 ]
3
要将参数对象转换为数组,我们首先采用了一个空数组。在该数组上,我们使用 call()
方法调用 slice()
方法。现在 slice()
方法将遍历传递的对象。在这种情况下,它是 arguments
对象,然后它将对象内的所有元素附加到空数组中。最后,我们将把 this 的结果存储在一个变量 args
中。
由于此变量现在包含 arguments
对象曾经包含的所有元素,因此你可以使用 args
变量访问每个元素及其适当的索引号。
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn