How to Convert Byte to Int in Java
- Convert Byte to Int Using Type Casting
-
Convert Byte to Int Using
Integer.valueOf()
-
Convert Byte to Int Using
Byte.intValue()
-
Convert Byte to Int Using
BigInteger
Class - Convert Byte to Int Using Bitwise Operations
-
Convert Byte to Int Using
Byte.toUnsignedInt()
- Conclusion
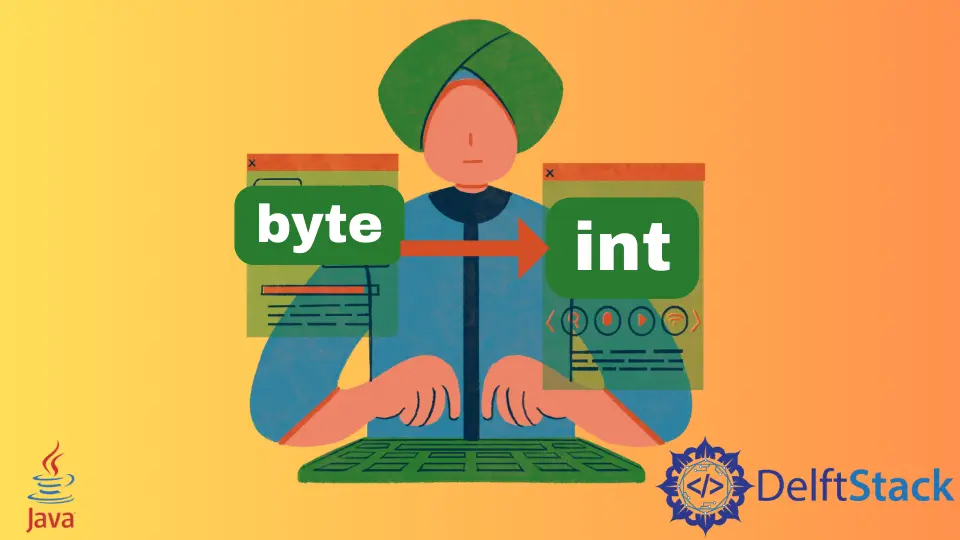
In this article, we’re going to discuss how you can convert a byte into an int data type.
A byte is an 8-bit signed data type, ranging from -128 to 127, while an int is a 32-bit signed data type with a broader range of -231 to 231-1.
Ints are used for larger numeric values, offering increased precision compared to bytes. Unless there is concern about memory, an integer is the default data type used to store integral values.
Converting a byte to an int in Java is a fundamental operation crucial for accurate data representation. While it may seem straightforward, there are nuances to consider, especially when dealing with signed and unsigned values.
There are techniques that offer concise and efficient approaches, ensuring precision in numeric conversions.
Convert Byte to Int Using Type Casting
Converting a byte to int using type casting in Java involves directly assigning the byte value to an int variable. This simple process retains the original byte value, facilitating compatibility between the two data types while expanding the range for larger integer values.
Take into consideration that converting a byte to int through type casting may lead to loss of precision as it doesn’t handle potential overflow or sign extension issues. This method is straightforward but requires caution with larger byte values to avoid unintended behavior in the resulting int.
Let’s start by examining a simple yet illustrative code example that demonstrates how to convert a byte to an int using type casting:
public class ByteToIntConversion {
public static void main(String[] args) {
// Step 1: Declare and initialize a byte variable
byte byteValue = 127;
// Step 2: Convert byte to int using type casting
int intValue = (int) byteValue;
// Step 3: Display the results
System.out.println("Original Byte Value: " + byteValue);
System.out.println("Converted Int Value: " + intValue);
}
}
In this example, we start the process by declaring and initializing a byte variable named byteValue
, which can be any valid byte within the range of -128 to 127.
Moving to the next step, we employ type casting with (int)
to explicitly convert the byte value to an integer.
The final step involves displaying the original byte value and the converted int value using System.out.println()
, providing a visual confirmation of the successful byte-to-int conversion.
Output:
Original Byte Value: 127
Converted Int Value: 127
This output confirms that the byte-to-int conversion using type casting was successful, and the values remain consistent.
Convert Byte to Int Using Integer.valueOf()
Converting a byte to an int using Integer.valueOf()
involves invoking this method with the byte value as an argument. This approach simplifies the process, providing a straightforward and reliable conversion and maintaining compatibility between the two data types in Java.
Using the Integer.valueOf()
method in Java ensures a reliable transition, handling potential data loss or unexpected behavior providing a convenient and precise conversion mechanism.
The syntax for converting a byte to an int using the valueOf
method in Java is as follows:
Integer.valueOf(byteValue)
Replace the byteValue
with the actual byte value you want to convert to an int.
Let’s dive into a comprehensive example that demonstrates how to convert a byte to an int using the Integer.valueOf()
method:
public class ByteToIntConversion {
public static void main(String[] args) {
// Step 1: Declare and initialize a byte variable
byte byteValue = 127;
// Step 2: Convert byte to int using Integer.valueOf()
int intValue = Integer.valueOf(byteValue);
// Step 3: Display the results
System.out.println("Original Byte Value: " + byteValue);
System.out.println("Converted Int Value: " + intValue);
}
}
To initiate the process, we declare and initialize a byte variable named byteValue
, assigned the value of 127
.
Subsequently, we utilize the Integer.valueOf()
method for byte-to-int conversion, allowing a seamless transition between the two data types. The method takes a byte parameter and returns an Integer
object, which is then automatically unboxed to an int.
The results are then displayed using System.out.println()
, confirming the successful conversion of the original byte value to its corresponding integer representation.
Output:
Original Byte Value: 127
Converted Int Value: 127
This output confirms the success of the byte-to-int conversion using the Integer.valueOf()
method. Incorporating this method into your Java programming arsenal will contribute to writing more concise and readable code while maintaining the accuracy of your numeric data representation.
Convert Byte to Int Using Byte.intValue()
The Byte.intValue()
method emerges as a straightforward approach for this specific transformation. This method is part of the Byte
class in Java and simplifies the process of converting a byte to its integer counterpart.
Converting a byte to an int using the Byte.intValue()
method in Java facilitates a quick and effective transformation without the need for additional casting.
There is no intValue()
method directly in the Byte
class. To convert a byte to an int, you can use the intValue()
method of the Byte
class after converting the byte to an int using the byteValue()
method.
Here’s the syntax:
byteObject.byteValue()
This will return a primitive byte, and then you can convert it to an int using the intValue()
method of the Byte
class:
Byte.valueOf(byteObject.byteValue()).intValue()
Replace byteObject
with the actual Byte
object you want to convert to an int.
Let’s delve into a comprehensive example that illustrates the simplicity and effectiveness of the Byte.intValue()
method in converting a byte to an integer:
public class ByteToIntConversion {
public static void main(String[] args) {
// Step 1: Declare and initialize a byte variable
byte byteValue = 85;
// Step 2: Convert byte to int using Byte.intValue()
int intValue = Byte.valueOf(byteValue).intValue();
// Step 3: Display the results
System.out.println("Original Byte Value: " + byteValue);
System.out.println("Converted Int Value: " + intValue);
}
}
Navigating through our process, we kick off with the establishment of a byte variable named byteValue
and its assignment of a value of 85
. This byte value aligns within the acceptable byte range (-128 to 127).
Proceeding to a critical phase, we utilize Byte.valueOf(byteValue)
to create a Byte
object, and then, the intValue()
method is invoked directly on this object. This method seamlessly furnishes the integer representation of the byte, eliminating the need for explicit casting.
To confirm our conversion, we turn to the trusty System.out.println()
to showcase both the original byte value and the transformed int value.
Output:
Original Byte Value: 85
Converted Int Value: 85
This output serves as a testament to the effectiveness of the byte-to-int conversion using the Byte.intValue()
method. By incorporating this method into your Java programming toolkit, you streamline the process of converting bytes to integers, enhancing code readability and efficiency.
Convert Byte to Int Using BigInteger
Class
The BigInteger
class is an immensely powerful tool for handling arbitrary-precision integers.
Converting a byte to an int using the BigInteger
class in Java ensures precision in numeric representation. This method is particularly valuable when handling arbitrary-precision integers, preventing data loss, and accurately converting bytes to integers.
Let’s delve into a practical example that demonstrates how the BigInteger
class can be employed for byte-to-int conversion:
import java.math.BigInteger;
public class ByteToIntConversion {
public static void main(String[] args) {
// Step 1: Declare and initialize a byte variable
byte byteValue = 42;
// Step 2: Convert byte to int using BigInteger
int intValue = new BigInteger(new byte[] {byteValue}).intValue();
// Step 3: Display the results
System.out.println("Original Byte Value: " + byteValue);
System.out.println("Converted Int Value: " + intValue);
}
}
We first establish a byte variable named byteValue
with a value of 42
, representing a signed byte within the acceptable range (-128 to 127).
Moving to a crucial juncture, we leverage the BigInteger
class to create an instance using the byte value. The intValue()
method is then employed to extract the accurate integer representation, addressing potential precision concerns.
Finally, we confirm the triumph of our conversion by displaying both the original byte value and the resulting int value using System.out.println()
.
Output:
Original Byte Value: 42
Converted Int Value: 42
This output attests to the success of the byte-to-int conversion using the BigInteger
class.
Convert Byte to Int Using Bitwise Operations
When it comes to converting a byte to an int, developers often turn to bitwise operations. These operations provide a low-level but highly efficient mechanism for manipulating individual bits, making them a valuable tool for byte-to-int conversion.
Converting a byte to an int using bitwise operations in Java involves bitwise AND
with 0xFF
. This method ensures accurate conversion, treating the byte as an unsigned entity and preventing sign extension issues during the process.
Let’s dive into a comprehensive example that illustrates the application of bitwise operations for converting a byte to an integer:
public class ByteToIntConversion {
public static void main(String[] args) {
// Step 1: Declare and initialize a byte variable
byte byteValue = 127;
// Step 2: Convert byte to int using Bitwise Operations
int intValue = byteToUnsignedInt(byteValue);
// Step 3: Display the results
System.out.println("Original Byte Value: " + byteValue);
System.out.println("Converted Int Value: " + intValue);
}
// Custom method for byte to unsigned int conversion using bitwise operations
private static int byteToUnsignedInt(byte byteValue) {
return byteValue & 0xFF;
}
}
We commence by declaring a byte variable, byteValue
, and assigning it a value of 127
. This byte value encapsulates a signed byte within the acceptable range of -128 to 127.
Progressing to a crucial stage, we introduce a custom method, byteToUnsignedInt
, specifically designed for byte-to-int conversion using bitwise operations. Within this method, bitwise AND
(&
) with 0xFF
is employed, strategically clearing higher-order bits and treating the byte as unsigned for an accurate conversion to an integer.
We rely on the steadfast System.out.println()
to showcase both the original byte value and the transformed int value, affirming the success of our conversion process.
Output:
Original Byte Value: 127
Converted Int Value: 127
This output serves as a testament to the effectiveness of the byte-to-int conversion using bitwise operations. By incorporating this approach into your Java programming toolkit, you gain a deeper understanding of low-level bit manipulation and ensure precision in numeric representation.
Convert Byte to Int Using Byte.toUnsignedInt()
When dealing with bytes as unsigned values, the standard casting methods might not suffice. We’ll explore the Byte.toUnsignedInt()
method—a specialized tool in the Java arsenal for converting bytes to unsigned integers.
Converting a byte to an int using the Byte.toUnsignedInt()
method in Java provides a dedicated solution for handling unsigned byte values. This method is crucial when working with raw byte data, ensuring accurate numeric representation and preventing unexpected behavior during the conversion process.
The syntax for converting a byte to an unsigned int using the toUnsignedInt()
method in Java is as follows:
Byte.toUnsignedInt(byteValue)
Replace byteValue
with the actual byte value you want to convert to an unsigned int.
Understanding this method is vital for scenarios where unsigned byte values need to be accurately represented as integers.
Let’s start examining this practical example that demonstrates the usage of Byte.toUnsignedInt()
:
public class ByteToUnsignedIntConversion {
public static void main(String[] args) {
// Step 1: Declare and initialize a byte variable with an unsigned value
byte unsignedByteValue = (byte) 0xFF;
// Step 2: Convert unsigned byte to int using Byte.toUnsignedInt()
int unsignedIntValue = Byte.toUnsignedInt(unsignedByteValue);
// Step 3: Display the results
System.out.println("Original Unsigned Byte Value: " + Byte.toUnsignedInt(unsignedByteValue));
System.out.println("Converted Unsigned Int Value: " + unsignedIntValue);
}
}
We initiate the process by declaring and initializing a byte variable named unsignedByteValue
with the hexadecimal value 0xFF
, representing an unsigned byte with all bits set to 1.
Using 0xFF
in converting a byte to an int in Java ensures that only the last 8 bits of the byte are considered, effectively treating the byte as unsigned. This prevents sign extension and ensures accurate representation in the resulting int.
The pivotal step involves using the Byte.toUnsignedInt()
method for byte-to-int conversion; it takes a byte as a parameter and returns its unsigned representation as an int, ensuring the byte’s unsigned representation is accurately maintained as an int.
Validating our conversion, we employ System.out.println()
to display both the original unsigned byte value and the corresponding converted unsigned int value.
Output:
Original Unsigned Byte Value: 255
Converted Unsigned Int Value: 255
This output verifies the success of the byte-to-unsigned-int conversion using the Byte.toUnsignedInt()
method. By incorporating this method into your Java toolkit, you ensure precision when dealing with unsigned byte values, enhancing the robustness of your numeric data handling.
Conclusion
Converting a byte to an int in Java is a fundamental operation with implications for accurate data representation.
While type casting provides a simple approach, caution is necessary to avoid precision loss, especially with larger byte values. Techniques such as using Integer.valueOf()
, Byte.intValue()
, BigInteger
class, bitwise operations, and Byte.toUnsignedInt()
offer concise and efficient alternatives, catering to various scenarios and ensuring precision in numeric conversions.
Developers can choose the method that best fits their specific requirements, striking a balance between simplicity and accuracy in byte-to-int conversions.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Byte
- How to Convert Java String Into Byte
- How to Convert Int to Byte in Java
- How to Write Byte to File in Java