How to Iterate Over Array of Objects in TypeScript
- Arrays in TypeScript
-
Iterate Over an Array of Objects Using the
forEach
Method in TypeScript -
Iterate Over an Array of Objects Using the
for...of
Statement in TypeScript -
Iterate Over an Array of Objects Using the
for...in
Statement in TypeScript -
Iterate Over an Array of Objects Using the
for
Loop in TypeScript
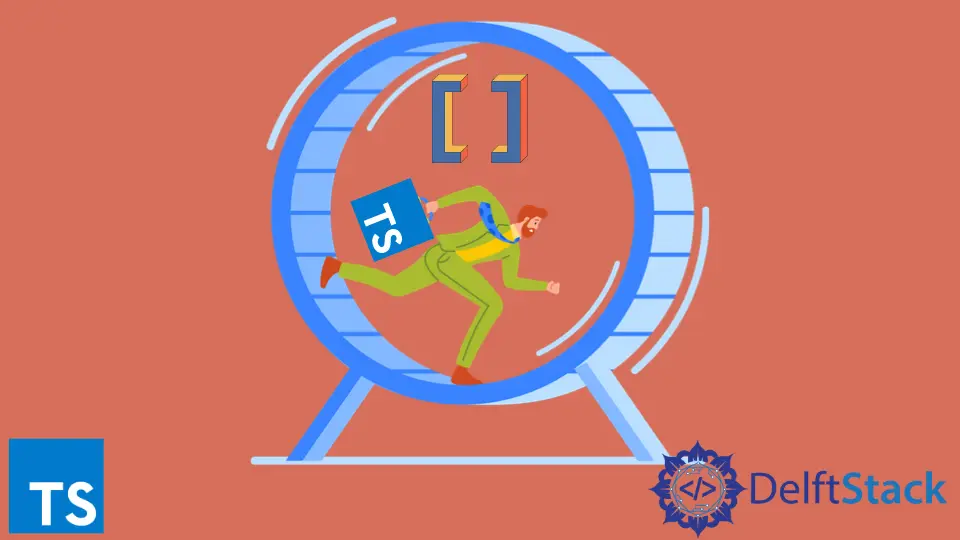
This article will explain how we can iterate over an array of objects in TypeScript.
Arrays in TypeScript
TypeScript arrays are user-defined. It is a special data type that can store multiple values of various data types such as strings, numbers, etc.
An array is a fixed-size data structure. The array of elements is stored in index-based storage.
The first element is stored at index 0 and increments by one for each added element.
Index | Value/Element |
---|---|
0 | 12 |
1 | 25 |
2 | 100 |
3 | 45 |
TypeScript uses the following syntax to declare and initialize an array.
let carBrands: string[] = ["Audi", "BMW", "Toyota", "Mercedes"];
Access Array Elements
The array of elements can be accessed like below using the index of an element.
Example code:
console.log(carBrands[0]);
console.log(carBrands[1]);
console.log(carBrands[2]);
console.log(carBrands[3]);
Output:
Audi
BMW
Toyota
Mercedes
There are several ways you iterate over an array of elements in TypeScript.
- The
forEach
method - The
for
loop - The
for...of
statement - The
for...in
statement
Iterate Over an Array of Objects Using the forEach
Method in TypeScript
This method executes a function on each element or object in the TypeScript array. The forEach
method can be declared as shown in the following.
Syntax:
your_array.forEach(callback function);
Let’s declare an array of strings.
let carBrands: string[] = ["Audi", "BMW", "Toyota", "Mercedes"];
We will copy all the array elements from carBrands
to another array using the forEach
method. Let’s declare an empty array called copyOfCarBrands
.
let copyOfCarBrands: string[] = [];
Let’s use the forEach
method to iterate over each element in the carBrands
array and push it to the new copyOfCarBrands
array.
carBrands.forEach(function(brand){
copyOfCarBrands.push(brand);
});
Finally, let’s print the copyOfCarBrands
array.
console.log(copyOfCarBrands);
Output:
[ 'Audi', 'BMW', 'Toyota', 'Mercedes' ]
We can use the forEach
method with multi-type arrays too. Let’s define a multi-type array called multiValues
and log each item to the console.
let multiValues: (string | number)[] = ['Asia', 1000, 'Europe', 340, 20];
multiValues.forEach(function(value){
console.log(value);
});
Output:
Asia
1000
Europe
340
20
There are a couple of drawbacks to the forEach
method.
- This method can only be used with arrays.
- There is no way to break the loop.
Iterate Over an Array of Objects Using the for...of
Statement in TypeScript
The for...of
loop statement can access elements of an array and return them. It can be used as shown below.
Syntax:
for (let variable of givenArray ) {
statement;
}
In each iteration, variable
will be assigned to an array element of givenArray
.
Let’s declare an array.
let fruits: string[] = ["Apple", "Grapes", "Mangoe", "Banana"];
The for...of
loop can be used below to access each element in the fruits
array.
for (let fruitElement of fruits) {
console.log(fruitElement);
}
Output:
Apple
Grapes
Mangoe
Banana
Iterate Over an Array of Objects Using the for...in
Statement in TypeScript
The for...in
loop syntax is almost similar to for...of
. But, it returns the index of array elements in each iteration.
This loop can be used as shown in the following.
Syntax:
for (let variable in givenArray ) {
statement;
}
The variable
will be assigned to the index of each element in each iteration. givenArray
is the iterable array.
Let’s define an array of numbers.
let numberArr: number[] = [100,560,300,20];
We can use the returned index value to access each element of numberArr
.
for (let variable in numberArr ) {
console.log(numberArr[variable]);
}
In each iteration, the variable
is assigned to the index of each element in numberArr
. Therefore, we can access the element value by numberArr[index]
.
In this case, the index
value would be variable
in each iteration.
Output:
100
560
300
20
Iterate Over an Array of Objects Using the for
Loop in TypeScript
The for
loop is the most common way to iterate over array elements. It can be used as below.
Syntax:
for (let index; index<arraySize; index++) {
statement;
}
The index
value increments in each iteration and the loop continues until the condition index < arraySize
is satisfied.
Let’s create an array of strings.
let oceanArr: string[] = ['Atlantic', 'Indian', 'Pacific'];
We can iterate over each element in oceanArr
and print as shown below.
for (let index=0; index<oceanArr.length; index++) {
console.log(oceanArr[index]);
}
Output:
Atlantic
Indian
Pacific
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.
Related Article - TypeScript Array
- How to Create an Empty Array in TypeScript
- How to Define an Array in TypeScript Interface
- How to Sort Array of Objects in TypeScript
- How to Initialize a Map Containing Arrays in TypeScript
- How to Initialize an Array in TypeScript