How to Push an Object Into an Array With TypeScript
- Initialize the Project
-
Use the
array.push
Method to Push an Object Into an Array With TypeScript -
Use the
array.concat
Method to Push an Object Into an Array With TypeScript - Use the Spread Operator to Push an Object Into an Array With TypeScript
- Use the Spread Operator Without Mutating the Object to Push an Object Into an Array With TypeScript
- Conclusion
- References
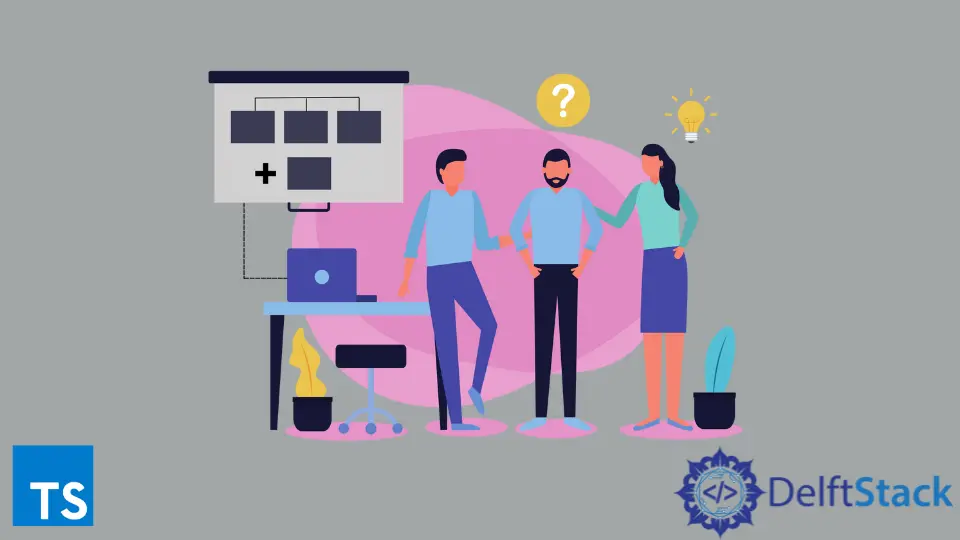
After reading this guide, you will know how to push an object into an array with TypeScript.
Initialize the Project
We will be using Vite to quickly start a new project using vanilla-ts
. After installing the necessary packages, we will be creating two object interfaces to use throughout the rest of the guide.
export interface Comment {
rating: number;
comment: string;
author: string;
date: string;
}
export interface Dish {
id: number;
name: string;
image: string;
category: string;
description: string;
comments: Comment[];
}
As you can see, there is the Dish
object, which has a comments
property with an array inside. This array can fit objects following the Comment
interface.
The goal is to push new comments to the comments
property. To show this, we will be using the following objects as examples.
import {Dish, Comment} from "./interfaces";
export let pastaDish: Dish = {
id: 124,
name: "Carbonara Pasta",
image: "pasta-carbonara.jpg",
category: "Italian Food",
description:
"Italian pasta dish originating in Lazio based on eggs, cheese, extra virgin olive oil, pancetta or guanciale, and bacon with black pepper.",
comments: [],
};
export let pastaComment: Comment = {
rating: 5,
comment: "Very tasty!",
author: "Juan Diego",
date: "04/14/2022",
};
Use the array.push
Method to Push an Object Into an Array With TypeScript
When called from an array, push
adds one or more elements at the end of the array. It doesn’t return the new array but edits the original one.
We can call this method from the comments
property to add a new element.
import {Dish, Comment} from "./interfaces";
import {pastaDish, pastaComment} from "./pastaDish";
const addNewComment = (dish: Dish, newComment: Comment) => {
dish.comments.push(newComment);
console.log(dish.comments);
};
addNewComment(pastaDish, pastaComment);
Expected output:
[
{
rating: 5,
comment: "Very tasty!",
author: "Juan Diego",
date: "04/14/2022",
},
];
Let’s break down what’s happening here: we create a new function called addNewComment
which will take a dish
and a newComment
as arguments.
Then we access the comments
property and call its push
method with the newComment
as its only argument. With this, we already pushed an object into the array.
Use the array.concat
Method to Push an Object Into an Array With TypeScript
This solution works fine, but the array.push
method mutates the dish
object. In other words, the original dish
object changes, which is considered a bad practice.
A mutation is a side effect: the fewer things that change in a program, the less there is to keep track of, which results in a simpler program. (Federico Knüssel)
We can refactor our code to use the array.concat
method to solve the mutation problem. The array.concat
works similarly to the array.push
, but instead of changing the original array, it returns a new one.
const addNewComment = (dish: Dish, newComment: Comment) => {
const editedComments = dish.comments.concat(newComment);
};
As you may notice, we create a new comments
array with this method, but we don’t have a new dish
object to return. To solve this, we can use the Object.assign
global method, which copies the properties from a source
object into a target
object and returns the modified target object.
The first argument will be our target, and the other arguments our sources. In this case, we will use an empty object {}
as our target and editedComments
and dish
as our sources.
import {Dish, Comment} from "./interfaces";
import {pastaDish, pastaComment} from "./pastaDish";
const addNewComment = (dish: Dish, newComment: Comment) => {
const editedComments = dish.comments.concat(newComment);
const editedDish = Object.assign({}, dish, {comments: editedComments});
console.log(editedDish.comments);
return editedDish;
};
addNewComment(pastaDish, pastaComment);
If we forget to add {}
as the first argument, editedComments
will be copied into dish
, and we will mutate the original dish
object.
{}
and assign editedComments
inside a comments
property to make the property depth and name equal in both objects. In this way, the assign
method can successfully copy the editedComments
.Expected output:
[
{
rating: 5,
comment: "Very tasty!",
author: "Juan Diego",
date: "04/14/2022",
},
];
Use the Spread Operator to Push an Object Into an Array With TypeScript
Another way to add an object to an array is using the ES6 spread operator. The spread operator is denoted with three dots ...
and can be used - among other things - to expand an object or array’s properties.
We can use the spread operator to create a new array with the new comments and then assign it to the dish.comments
.
import {Dish, Comment} from "./interfaces";
import {pastaDish, pastaComment} from "./pastaDish";
const addNewComment = (dish: Dish, newComment: Comment) => {
const {comments} = dish;
dish.comments = [...comments, newComment];
console.log(dish.comments);
};
addNewComment(pastaDish, pastaComment);
Expected output:
[
{
rating: 5,
comment: "Very tasty!",
author: "Juan Diego",
date: "04/14/2022",
},
];
First of all, we use object destructuring to access the comments
property from dish
. We then use the spread operator to expand the comments
elements on a new array with the newComment
as its last element and assign it to the original dish.comments
property.
Use the Spread Operator Without Mutating the Object to Push an Object Into an Array With TypeScript
As you may notice, we also mutate the dish
original value with the previous approach. However, we can easily fix this by creating and returning a new dish
object with the edited comments
property.
Fortunately, we can achieve this by using the spread operator one more time to create a copy of the dish
object.
import {Dish, Comment} from "./interfaces";
import {pastaDish, pastaComment} from "./pastaDish";
const addNewComment = (dish: Dish, newComment: Comment) => {
const {comments} = dish;
const editedDish = {...dish, comments: [...comments, newComment]};
console.log(editedDish.comments);
return editedDish;
};
addNewComment(pastaDish, pastaComment);
Expected output:
[
{
rating: 5,
comment: "Very tasty!",
author: "Juan Diego",
date: "04/14/2022",
},
];
First, we create a new object using empty braces {}
; then we expand the dish
properties inside of the new object by using the spread operator ...
, but we explicitly add a new comments
property so we can assign it a new value, which in this case will be an array of the existing comments plus the newComment
.
{} // New Object
{...dish} // New Dish
{...dish, comments: []} // New Dish With Empty Comments
{...dish, comments: [...comments, newComment]} // New Dish With New Comments
Conclusion
You can use any previous approaches; however, we recommend using the non-mutating ones since it is considered a good practice. On the other side, using the spread operator can become a problem since it came out with ECMAScript 6, so it may not work in old legacy browsers.
Therefore if browser accessibility is a big concern, stick to array.push
and array.concat
, or compile your code using Babel.
References
- Mozilla Documentation Network. (N.D) Array.prototype.push() - JavaScript | MDN. Retrieved 14 April 2022, from https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/push
- Knüssel F. (2017). Arrays, Objects and Mutations. Retrieved 14 April 2022, from https://medium.com/@fknussel/arrays-objects-and-mutations-6b23348b54aa
- Mozilla Documentation Network. (N.D) Array.prototype.concat() - JavaScript | MDN. Retrieved 14 April 2022, from https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/concat
- Mozilla Documentation Network. (N.D) Array.prototype.assign() - JavaScript | MDN. Retrieved 14 April 2022, from https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/assign
- Mozilla Documentation Network. (N.D) Spread syntax (…) - JavaScript | MDN. Retrieved 14 April 2022, from https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Spread_syntax
Juan Diego Rodríguez (also known as Monknow) is a front-end developer from Venezuela who loves to stay updated with the latest web development trends, making beautiful websites with modern technologies. But also enjoys old-school development and likes building layouts with vanilla HTML and CSS to relax.
LinkedInRelated Article - TypeScript Object
- How to Merge Objects TypeScript
- How to Iterate Over Array of Objects in TypeScript
- Default Value for Object Parameters in TypeScript
- How to Dynamically Assign Properties to an Object in TypeScript
- How to Declare Array of Objects in TypeScript