TypeScript를 사용하여 개체를 배열로 푸시
- 프로젝트 초기화
-
array.push
메서드를 사용하여 TypeScript로 개체를 배열로 밀어넣기 -
array.concat
메서드를 사용하여 TypeScript로 개체를 배열로 밀어넣기 - 확산 연산자를 사용하여 TypeScript로 객체를 배열로 밀어넣기
- 개체를 변경하지 않고 확산 연산자를 사용하여 TypeScript를 사용하여 개체를 배열에 밀어넣기
- 결론
- 참조
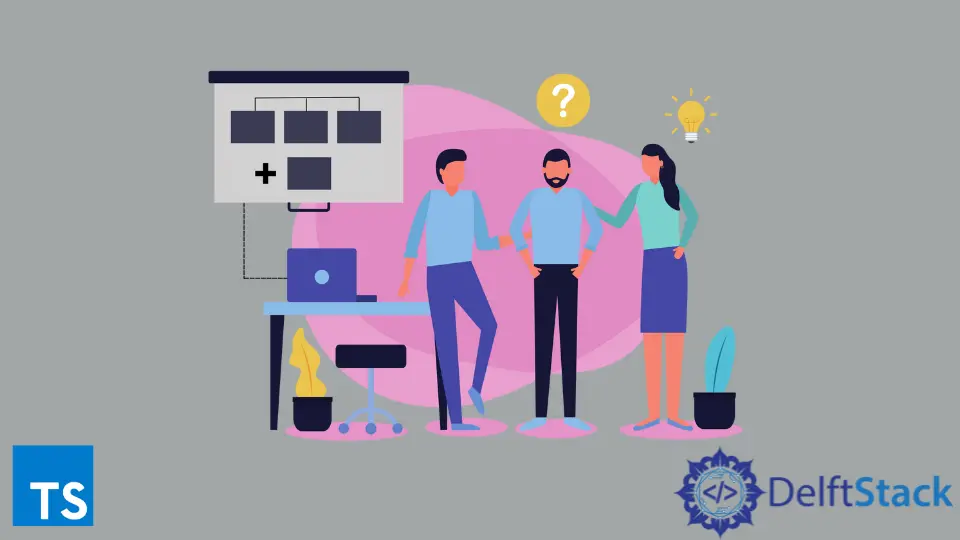
이 가이드를 읽고 나면 TypeScript를 사용하여 개체를 배열에 푸시하는 방법을 알게 될 것입니다.
프로젝트 초기화
우리는 vanilla-ts
를 사용하여 새 프로젝트를 빠르게 시작하기 위해 Vite를 사용할 것입니다. 필요한 패키지를 설치한 후 가이드의 나머지 부분에서 사용할 두 개의 개체 인터페이스를 만들 것입니다.
export interface Comment {
rating: number;
comment: string;
author: string;
date: string;
}
export interface Dish {
id: number;
name: string;
image: string;
category: string;
description: string;
comments: Comment[];
}
보시다시피 내부에 배열이 있는 comments
속성이 있는 Dish
개체가 있습니다. 이 배열은 Comment
인터페이스를 따르는 개체에 맞을 수 있습니다.
목표는 comments
속성에 새 댓글을 푸시하는 것입니다. 이를 보여주기 위해 다음 개체를 예로 사용합니다.
import {Dish, Comment} from "./interfaces";
export let pastaDish: Dish = {
id: 124,
name: "Carbonara Pasta",
image: "pasta-carbonara.jpg",
category: "Italian Food",
description:
"Italian pasta dish originating in Lazio based on eggs, cheese, extra virgin olive oil, pancetta or guanciale, and bacon with black pepper.",
comments: [],
};
export let pastaComment: Comment = {
rating: 5,
comment: "Very tasty!",
author: "Juan Diego",
date: "04/14/2022",
};
array.push
메서드를 사용하여 TypeScript로 개체를 배열로 밀어넣기
배열에서 호출될 때 push
는 배열 끝에 하나 이상의 요소를 추가합니다. 새 배열을 반환하지 않고 원래 배열을 편집합니다.
comments
속성에서 이 메서드를 호출하여 새 요소를 추가할 수 있습니다.
import {Dish, Comment} from "./interfaces";
import {pastaDish, pastaComment} from "./pastaDish";
const addNewComment = (dish: Dish, newComment: Comment) => {
dish.comments.push(newComment);
console.log(dish.comments);
};
addNewComment(pastaDish, pastaComment);
예상 출력:
[
{
rating: 5,
comment: "Very tasty!",
author: "Juan Diego",
date: "04/14/2022",
},
];
여기서 무슨 일이 일어나고 있는지 분석해 보겠습니다. dish
와 newComment
를 인수로 사용하는 addNewComment
라는 새 함수를 만듭니다.
그런 다음 comments
속성에 액세스하고 newComment
를 유일한 인수로 사용하여 push
메서드를 호출합니다. 이것으로 우리는 이미 개체를 배열로 푸시했습니다.
array.concat
메서드를 사용하여 TypeScript로 개체를 배열로 밀어넣기
이 솔루션은 잘 작동하지만 array.push
메서드는 dish
개체를 변경합니다. 즉, 원래 dish
개체가 변경되며 이는 나쁜 습관으로 간주됩니다.
돌연변이는 부작용입니다. 프로그램에서 변경되는 사항이 적을수록 추적할 항목이 적어져 프로그램이 더 간단해집니다. (페데리코 크뉘셀)
변형 문제를 해결하기 위해 array.concat
메서드를 사용하도록 코드를 리팩토링할 수 있습니다. array.concat
은 array.push
와 유사하게 작동하지만 원래 배열을 변경하는 대신 새 배열을 반환합니다.
const addNewComment = (dish: Dish, newComment: Comment) => {
const editedComments = dish.comments.concat(newComment);
};
알다시피 이 메서드를 사용하여 새 comments
배열을 생성하지만 반환할 새 dish
객체가 없습니다. 이를 해결하기 위해 source
개체의 속성을 target
개체로 복사하고 수정된 대상 개체를 반환하는 Object.assign
전역 메서드를 사용할 수 있습니다.
첫 번째 인수는 대상이 되고 다른 인수는 소스가 됩니다. 이 경우 빈 개체 {}
를 대상으로 사용하고 editedComments
및 dish
를 소스로 사용합니다.
import {Dish, Comment} from "./interfaces";
import {pastaDish, pastaComment} from "./pastaDish";
const addNewComment = (dish: Dish, newComment: Comment) => {
const editedComments = dish.comments.concat(newComment);
const editedDish = Object.assign({}, dish, {comments: editedComments});
console.log(editedDish.comments);
return editedDish;
};
addNewComment(pastaDish, pastaComment);
{}
를 첫 번째 인수로 추가하는 것을 잊어버리면 editedComments
가 dish
에 복사되고 원래 dish
객체를 변경합니다.
{}
를 사용하여 새 개체를 생성하고 comments
속성 내부에 editedComments
를 할당하여 두 개체에서 속성 깊이와 이름을 동일하게 만듭니다. 이러한 방식으로 assign
메서드는 성공적으로 editedComments
를 복사할 수 있습니다.예상 출력:
[
{
rating: 5,
comment: "Very tasty!",
author: "Juan Diego",
date: "04/14/2022",
},
];
확산 연산자를 사용하여 TypeScript로 객체를 배열로 밀어넣기
객체를 배열에 추가하는 또 다른 방법은 ES6 스프레드 연산자를 사용하는 것입니다. 스프레드 연산자는 세 개의 점 ...
으로 표시되며 무엇보다도 개체 또는 배열의 속성을 확장하는 데 사용할 수 있습니다.
스프레드 연산자를 사용하여 새 주석으로 새 배열을 만든 다음 이를 dish.comments
에 할당할 수 있습니다.
import {Dish, Comment} from "./interfaces";
import {pastaDish, pastaComment} from "./pastaDish";
const addNewComment = (dish: Dish, newComment: Comment) => {
const {comments} = dish;
dish.comments = [...comments, newComment];
console.log(dish.comments);
};
addNewComment(pastaDish, pastaComment);
예상 출력:
[
{
rating: 5,
comment: "Very tasty!",
author: "Juan Diego",
date: "04/14/2022",
},
];
우선, 우리는 object destructuring을 사용하여 dish
에서 comments
속성에 액세스합니다. 그런 다음 스프레드 연산자를 사용하여 newComment
를 마지막 요소로 사용하여 새 배열에서 comments
요소를 확장하고 이를 원래 dish.comments
속성에 할당합니다.
개체를 변경하지 않고 확산 연산자를 사용하여 TypeScript를 사용하여 개체를 배열에 밀어넣기
알다시피, 우리는 또한 이전 접근 방식으로 dish
원래 값을 변경합니다. 그러나 편집된 comments
속성을 사용하여 새 dish
객체를 생성하고 반환하면 이 문제를 쉽게 해결할 수 있습니다.
다행스럽게도 스프레드 연산자를 한 번 더 사용하여 dish
객체의 복사본을 생성함으로써 이를 달성할 수 있습니다.
import {Dish, Comment} from "./interfaces";
import {pastaDish, pastaComment} from "./pastaDish";
const addNewComment = (dish: Dish, newComment: Comment) => {
const {comments} = dish;
const editedDish = {...dish, comments: [...comments, newComment]};
console.log(editedDish.comments);
return editedDish;
};
addNewComment(pastaDish, pastaComment);
예상 출력:
[
{
rating: 5,
comment: "Very tasty!",
author: "Juan Diego",
date: "04/14/2022",
},
];
먼저 빈 중괄호 {}
를 사용하여 새 개체를 만듭니다. 그런 다음 스프레드 연산자 ...
를 사용하여 새 개체 내부의 dish
속성을 확장하지만 명시적으로 새 comments
속성을 추가하여 새 값을 할당할 수 있습니다. 기존 주석과 newComment
의 배열.
{} // New Object
{...dish} // New Dish
{...dish, comments: []} // New Dish With Empty Comments
{...dish, comments: [...comments, newComment]} // New Dish With New Comments
결론
이전 접근 방식을 사용할 수 있습니다. 그러나 변이가 없는 것을 사용하는 것이 좋은 방법으로 간주되므로 사용하는 것이 좋습니다. 반면 스프레드 연산자를 사용하는 것은 ECMAScript 6와 함께 나왔기 때문에 문제가 될 수 있으므로 이전 레거시 브라우저에서는 작동하지 않을 수 있습니다.
따라서 브라우저 접근성이 큰 관심사인 경우 array.push
및 array.concat
을 사용하거나 Babel을 사용하여 코드를 컴파일하십시오.
참조
- 모질라 문서 네트워크. (N.D) Array.prototype.push() - JavaScript | MDN. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/push에서 2022년 4월 14일에 검색함
- 크뉘셀 F.(2017). 배열, 객체 및 돌연변이. https://medium.com/@fknussel/arrays-objects-and-mutations-6b23348b54aa에서 2022년 4월 14일에 검색함
- Mozilla 문서 네트워크. (N.D) Array.prototype.concat() - JavaScript | MDN. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/concat에서 2022년 4월 14일에 검색함
- Mozilla 문서 네트워크. (N.D) Array.prototype.assign() - JavaScript | MDN. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/assign에서 2022년 4월 14일에 검색함
- 모질라 문서 네트워크. (N.D) 스프레드 구문(…) - JavaScript | MDN. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Spread_syntax에서 2022년 4월 14일에 검색함
Juan Diego Rodríguez (also known as Monknow) is a front-end developer from Venezuela who loves to stay updated with the latest web development trends, making beautiful websites with modern technologies. But also enjoys old-school development and likes building layouts with vanilla HTML and CSS to relax.
LinkedIn