TypeScript에서 객체 배열 정렬
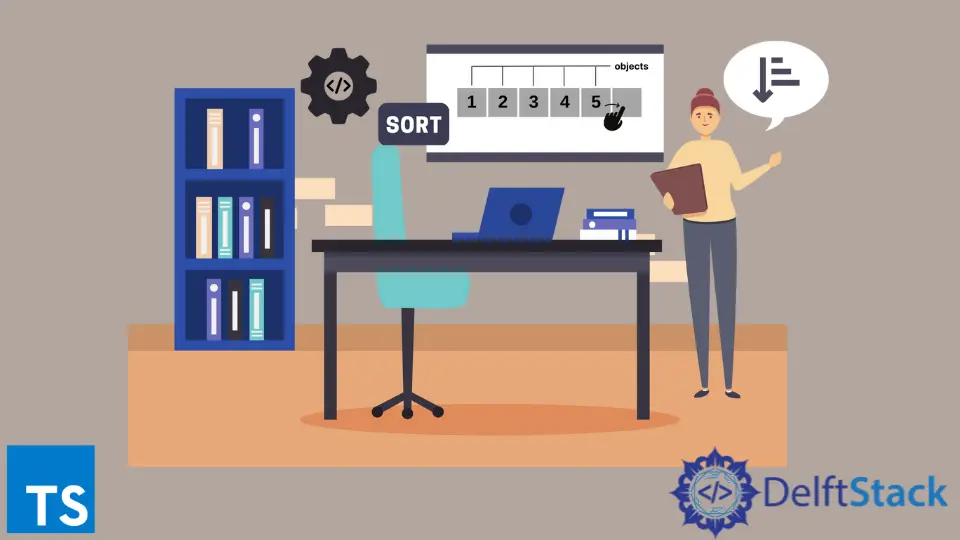
이 기사에서는 TypeScript에서 객체 배열을 정렬하는 방법을 보여줍니다.
TypeScript 객체
TypeScript는 강력한 형식의 언어입니다. 프리미티브, 배열, 객체 유형 등 다양한 유형을 지원합니다.
개체 유형은 속성을 사용하여 데이터를 나타낼 수 있는 특수 유형입니다. TypeScript 객체는 다음과 같이 JSON 객체처럼 보입니다.
{ id: 001, username: 'rick' }
id
와 username
은 위 개체의 두 속성입니다. 각 속성에도 값이 할당되었습니다.
TypeScript에서 인터페이스를 사용하여 사용자 정의 개체 유형을 만들 수 있습니다. 인터페이스를 사용하면 다음과 같이 개체 구조를 만들 수 있습니다.
interface employee {
empId: number,
empDesignation: string
}
위의 employee
인터페이스는 employee
객체를 각각 숫자 및 문자열 유형인 empId
및 empDesignation
의 두 가지 속성으로 정의합니다.
TypeScript 객체 배열
TypeScript는 요소 컬렉션을 저장할 수 있는 배열 유형을 지원합니다. 원시 유형 값과 사용자 정의 개체도 모두 저장할 수 있습니다.
employee
개체의 배열을 만들어 보겠습니다.
let employeesList: Array<employee> = [
{empId: 20, empDesignation: 'senior engineer'},
{empId: 14, empDesignation: 'junior engineer'},
{empId: 25, empDesignation: 'program manager'},
{empId: 12, empDesignation: 'director'}
]
위의 employeesList
배열에는 4개의 employee
개체가 포함되어 있습니다. 때때로 우리는 주어진 속성을 기준으로 이러한 객체를 정렬해야 합니다.
SQL이 열 또는 여러 열을 기준으로 데이터를 정렬하는 것과 같습니다. TypeScript는 기본적으로 정렬을 지원합니다.
내장 sort()
메소드는 객체 배열을 정렬할 수 있습니다.
TypeScript의 sort()
메서드
sort
메서드는 요소 배열을 가져와서 정렬된 배열을 반환합니다. 반환된 배열의 정렬 순서를 지정하는 데 사용할 수 있는 선택적 함수를 허용합니다.
통사론:
//1.
Array.sort()
//2.
Array.sort(<custom_function>)
Array
는 정렬할 객체의 배열입니다. <custom_function>
은 정렬 순서를 지정할 수 있습니다.
요구 사항은 속성 중 하나를 기준으로 객체 배열을 정렬하는 것이므로 정렬 순서와 객체 속성을 결정하는 사용자 지정 함수를 전달하여 sort
메서드를 사용해야 합니다.
id
속성을 기준으로 배열을 정렬해 보겠습니다.
employeesList.sort(
(firstObject: employee, secondObject: employee) =>
(firstObject.empId > secondObject.empId) ? 1 : -1
);
화살표 함수는 정렬 조건을 지정하기 위해 위의 예에서 사용되었습니다.
함수가 1
을 반환할 때마다 이는 secondObject
가 firstObject
보다 더 높은 정렬 우선 순위를 갖는다는 것을 의미합니다. 그렇지 않으면 firstObject
가 더 높은 정렬 우선순위를 갖습니다.
이렇게 하면 employeesList
개체가 empId
속성을 기준으로 오름차순으로 정렬됩니다.
출력:
화살표 함수 내에서 아래 조건으로 동일한 배열을 내림차순으로 정렬할 수 있습니다.
(firstObject: employee, secondObject: employee) =>
(firstObject.empId > secondObject.empId) ? -1 : 1
먼저 empId
를 기준으로 정렬한 다음 empDesignation
속성을 기준으로 정렬해야 하는 몇 가지 시나리오가 있습니다. empId
속성 값이 주어진 개체에 대해 동일한 경우 조건 연산자에서 empDesignation
속성을 확인할 수 있습니다.
let employeesList: Array<employee> = [
{empId: 20, empDesignation: 'senior engineer'},
{empId: 14, empDesignation: 'junior engineer'},
{empId: 14, empDesignation: 'developer'},
{empId: 25, empDesignation: 'program manager'},
{empId: 12, empDesignation: 'director'}
]
employeesList.sort(
(firstObject: employee, secondObject: employee) =>
(firstObject.empId > secondObject.empId) ? 1 :
(firstObject.empId === secondObject.empId) ? (
(firstObject.empDesignation > secondObject.empDesignation) ? 1 : -1 )
: -1
);
출력:
우리가 볼 수 있듯이 두 객체는 동일한 empId
14
를 갖습니다. 따라서 이 두 개체는 empDesignation
속성에 따라 정렬되었습니다.
sort
방법은 속성을 기반으로 개체를 정렬할 때 매우 강력합니다. 복잡한 유형 정렬에도 사용할 수 있습니다.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.