在 TypeScript 中对对象数组进行排序
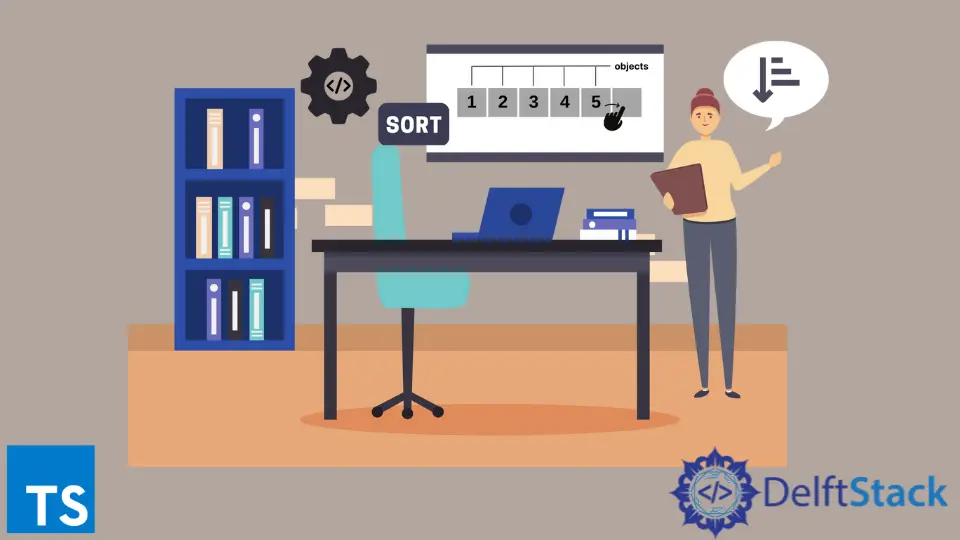
本文展示了如何在 TypeScript 中对对象数组进行排序。
TypeScript 对象
TypeScript 是一种强类型语言。它支持各种类型,例如基元、数组和对象类型。
对象类型是一种特殊类型,可以用属性来表示数据。TypeScript 对象看起来像一个 JSON 对象,如下所示。
{ id: 001, username: 'rick' }
id
和 username
是上述对象的两个属性。这些值也已分配给每个属性。
在 TypeScript 中,可以使用接口创建自定义对象类型。这些接口允许你创建对象结构,如下所示。
interface employee {
empId: number,
empDesignation: string
}
上面的 employee
接口定义了一个 employee
对象,它有两个属性 empId
和 empDesignation
,它们分别是数字和字符串类型。
TypeScript 对象数组
TypeScript 支持可以存储元素集合的数组类型。它也可以存储原始类型值和自定义对象。
让我们创建一个 employee
对象数组。
let employeesList: Array<employee> = [
{empId: 20, empDesignation: 'senior engineer'},
{empId: 14, empDesignation: 'junior engineer'},
{empId: 25, empDesignation: 'program manager'},
{empId: 12, empDesignation: 'director'}
]
上面的数组 employeesList
包含四个 employee
对象。有时,我们需要按给定属性对这些对象进行排序。
这就像 SQL 按一列或多列排序数据。TypeScript 支持开箱即用的排序。
内置的 sort()
方法可以对对象数组进行排序。
TypeScript 中的 sort()
方法
sort
方法接受一个元素数组并返回排序后的数组。它接受一个可选函数,该函数可用于指定返回数组的排序顺序。
语法:
//1.
Array.sort()
//2.
Array.sort(<custom_function>)
Array
是我们将排序的对象数组。 <custom_function>
可以指定排序顺序。
由于要求是按其属性之一对对象数组进行排序,因此我们必须通过传递决定排序顺序和对象属性的自定义函数来使用 sort
方法。
让我们按属性 id
对数组进行排序。
employeesList.sort(
(firstObject: employee, secondObject: employee) =>
(firstObject.empId > secondObject.empId) ? 1 : -1
);
上例中使用了箭头函数来指定排序条件。
每当函数返回 1
时,这意味着 secondObject
的排序优先级高于 firstObject
。否则,firstObject
具有更高的排序优先级。
这将按其 empId
属性按升序对 employeesList
对象进行排序。
输出:
可以使用箭头函数内的以下条件对相同的数组进行降序排序。
(firstObject: employee, secondObject: employee) =>
(firstObject.empId > secondObject.empId) ? -1 : 1
在某些情况下,你必须先按 empId
排序,然后再按 empDesignation
属性排序。如果给定对象的 empId
属性值相同,我们可以检查条件运算符中的 empDesignation
属性。
let employeesList: Array<employee> = [
{empId: 20, empDesignation: 'senior engineer'},
{empId: 14, empDesignation: 'junior engineer'},
{empId: 14, empDesignation: 'developer'},
{empId: 25, empDesignation: 'program manager'},
{empId: 12, empDesignation: 'director'}
]
employeesList.sort(
(firstObject: employee, secondObject: employee) =>
(firstObject.empId > secondObject.empId) ? 1 :
(firstObject.empId === secondObject.empId) ? (
(firstObject.empDesignation > secondObject.empDesignation) ? 1 : -1 )
: -1
);
输出:
如我们所见,两个对象具有相同的 empId``14
。因此,这两个对象已按 empDesignation
属性排序。
当根据对象的属性对对象进行排序时,sort
方法非常强大。它也可以用于复杂类型的排序。
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.