在 TypeScript 中排序数组
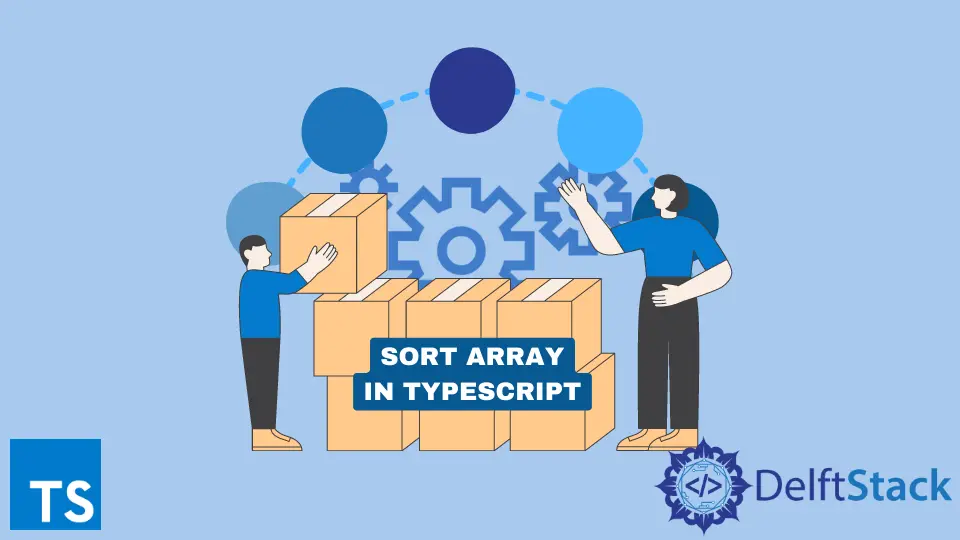
TypeScript 允许对数组进行不同的操作,排序是其中一种操作。本教程将讨论 typescript 中数组的排序。
在 TypeScript 中使用 sort()
方法对数组进行排序
排序是使用 sort()
方法完成的。此方法对数组中存在的元素进行排序,并将排序后的字符串元素作为输出返回给用户。
sort()
方法默认按升序返回结果。数组类型定义为 string[]
。
例子:
const myArray: string[] = ['A', 'B', 'D', 'X', 'C', 'F'];
myArray.sort();
console.log(`My sorted array is = ${myArray}`);
输出:
My sorted array is = A,B,C,D,F,X
sort()
方法返回字符串数组,数组元素按字母顺序给出作为输出。现在让我们再举一个字符串数组的例子。
例子:
const myFruits: string[] = ['Apple', 'Peach', 'Strawberry', 'Banana', 'Mango'];
myFruits.sort();
console.log(`My sorted fruits is = ${myFruits}`);
输出:
My sorted fruits is = Apple,Banana,Mango,Peach,Strawberry
请注意,所有水果均按字母顺序排序。
在 TypeScript 中对数字数组进行排序
在 TypeScript 中对数字数组进行排序并不像上面看起来那么简单,因为 sort()
方法返回字符串数组。执行逻辑运算以按升序和降序对数值数组进行排序。
例子:
const numArray: number[] = [6,5,8,2,1];
numArray.sort();
console.log(`The sorted numebrs are = ${numArray}`);
输出:
The sorted numebrs are = 1,2,5,6,8
上面的例子可以正常工作,但是如果我们添加一个最后一位为 0 的两位或三位数字会发生什么。让我们举个例子。
例子:
const numArray: number[] = [60,5,8,2,1];
numArray.sort();
console.log(`The sorted numebrs are = ${numArray}`);
输出:
The sorted numebrs are = 1,2,5,60,8
上面的代码片段表明,即使 60
大于 8
,它仍然排在第一位,因为 sort()
方法返回一个字符串数组。在字符串比较中,60
在 8
之前。
为了解决这个问题,我们必须在 sort()
方法中使用一个比较函数,这个函数将决定我们是要按升序还是降序排列数组。
例子:
const numArray: number[] = [60,5,8,2,1];
numArray.sort(function(x,y) {
return x-y
});
console.log(`The sorted numebrs are = ${numArray}`);
输出:
The sorted numebrs are = 1,2,5,8,60
结果以升序显示。要按降序显示结果,请按照以下示例进行操作。
例子:
const numArray: number[] = [60,5,8,2,1];
numArray.sort(function(x,y) {
return y-x
});
console.log(`The sorted numebrs are = ${numArray}`);
输出:
The sorted numebrs are = 60,8,5,2,1
现在结果以降序显示。function(x,y)
根据我们是否希望数字按升序或降序排列的逻辑进行操作。
在 TypeScript 中对对象数组进行排序
TypeScript 允许我们对数组中的对象进行排序。
例子:
var numArray: { num: number; }[] = [{ num: 7}, { num: 9 }, {num: 3}, {num: 6}, {num: 1}];
numArray.sort(function(x,y) {
if (x.num > y.num) {
return 1;
}
if (x.num < y.num) {
return -1;
}
return 0;
});
console.log('The sorted array of objects is as follow:');
console.log(numArray);
输出:
The sorted array of objects is as follow:
[{
"num": 1
}, {
"num": 3
}, {
"num": 6
}, {
"num": 7
}, {
"num": 9
}]
代码示例显示了已排序的对象数组。在条件中,return 1
表明第一个在第二个之后。
return -1
表示第一次在第二次之前,return 0
表示它们是相等的。结果以升序显示。
要按降序获得结果,请将条件中的 -1
替换为 1
,反之亦然。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn