在 TypeScript 中初始化包含数组的 Map
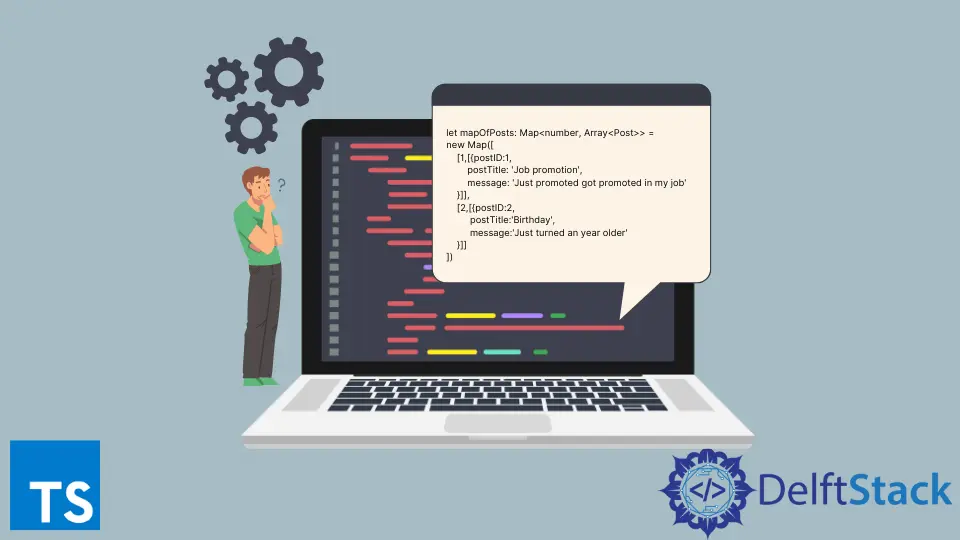
Map 是一种以键值对形式保存数据的数据结构。你可以对键和值使用你喜欢的任何数据类型。
在本教程中,我们将学习创建一个使用数字作为键和自定义类型数组作为值的 Map。
使用 TypeScript 中的 Array
类初始化包含数组的 Map
转到 Visual Studio Code 并创建一个名为 map-initialization
的文件夹或使用任何首选名称。在文件夹下创建一个名为 array-class-map.ts
的文件。
将以下代码复制并粘贴到文件中。
type Post = {
postID: number
postTitle: string
message: string
}
let mapOfPosts: Map<number, Array<Post>> =
new Map([
[1,[{postID:1,
postTitle: 'Job promotion',
message: 'Just promoted got promoted in my job'
}]],
[2,[{postID:2,
postTitle:'Birthday',
message:'Just turned an year older'
}]]
])
mapOfPosts.forEach((post) => console.log(post))
在上面的代码中,我们定义了名为 Post
的自定义对象,我们将在 Map 中将其用作值。
由于 Map 是通用的,尖括号的第一个参数表示键是 number
类型。第二个参数使用另一种名为 Array<Post>
的类型来指示该值是一个帖子数组。
Array
是一个通用类,用于通过在尖括号中指定元素的类型来创建元素数组。在我们的例子中,我们将 Post
作为尖括号中的元素类型传递。
ForEach()
是一个函数式方法,它在后台遍历 Map 中的元素数组并将它们打印到控制台。
要执行上面的示例,请转到终端并 cd
到该文件夹的位置。使用以下命令生成 tsconfig.json
文件。
david@david-HP-ProBook-6470b:~/Documents/map-initialization$ tsc --init
确保 tsconfig.json
文件具有以下配置。
{
"compilerOptions":{
"target": "es5"
"noEmitOnError": true,
}
}
Map API 是在 es5
中引入的,因此我们添加了该库以防止代码中出现任何编译错误。
使用以下命令将 TypeScript 代码转换为可以使用 node
执行的 JavaScript 代码。
david@david-HP-ProBook-6470b:~/Documents/map-initialization$ tsc
编译代码后,将生成一个名为 array-class-map.js
的文件。使用以下命令执行 JavaScript 文件。
david@david-HP-ProBook-6470b:~/Documents/map-initialization$ node array-class-map.js
输出:
[
{
postID: 1,
postTitle: 'Job promotion',
message: 'Just promoted got promoted in my job'
}
]
[
{
postID: 2,
postTitle: 'Birthday',
message: 'Just turned an year older'
}
]
在 TypeScript 中使用方括号 []
初始化包含数组的 Map
在这个例子中,我们将使用大多数开发人员都知道的基本方法,即使是对数组有基本了解的人。
在同一文件夹下创建一个名为 maps.ts
的文件。将以下代码复制并粘贴到文件中。
type Post = {
postID: number
postTitle: string
message: string
}
let mapOfPosts: Map<number, Post[]> =
new Map([
[1,[{postID:1,
postTitle: 'Job promotion',
message: 'Just promoted got promoted in my job'
}]],
[2,[{postID:2,
postTitle:'Birthday',
message:'Just turned an year older'
}]]
])
mapOfPosts.forEach((post) => console.log(post))
在上面的代码中,我们定义了一个 Map,它接受类型为 number
的键和一个 Post
数组作为值。
创建数组的基本方法是定义类型,后跟方括号,如 Post[]
所示。地图已使用 new
关键字在一行上进行了初始化,并且已将两个具体元素添加到地图中。
此示例的工作方式与上一个示例相同,除了它使用方括号为 Map 值创建帖子数组这一事实。
要执行此示例,请使用与上一个示例相同的方法来获取以下输出。
输出:
[
{
postID: 1,
postTitle: 'Job promotion',
message: 'Just promoted got promoted in my job'
}
]
[
{
postID: 2,
postTitle: 'Birthday',
message: 'Just turned an year older'
}
]
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub