检查 TypeScript 数组中是否存在字符串
Shuvayan Ghosh Dastidar
2023年1月30日
TypeScript
TypeScript Array
-
使用
includes()
方法检查 TypeScript 数组中是否存在字符串 -
使用
indexOf()
方法检查 TypeScript 数组中是否存在字符串 -
使用
find()
方法检查 TypeScript 数组中是否存在字符串 -
使用
some()
方法检查 TypeScript 数组中是否存在字符串
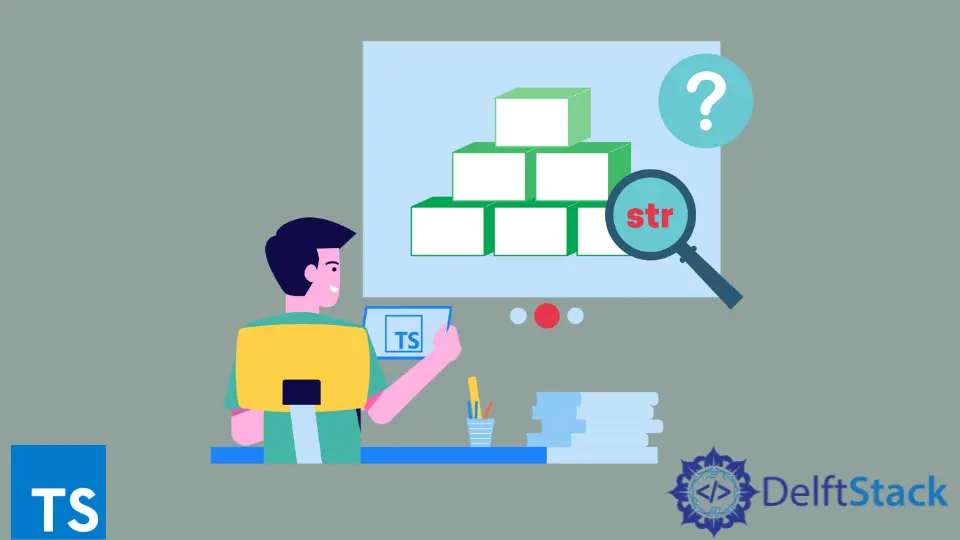
本文将演示如何使用数组原型中存在的各种内置函数来搜索数组中的字符串。
使用 includes()
方法检查 TypeScript 数组中是否存在字符串
includes()
方法确定目标元素是否存在于数组中。它接收目标元素并返回一个布尔值来表示数组中的值。
在检查相等时,该算法认为所有零值都相等,而不管符号如何。 (-0、+0 都被认为等于 0),但 false
不等于 0。它使用 sameValueZero
算法来搜索数组中是否存在元素。
以下代码段显示了如何使用 includes()
方法。
var fruitsArray : string[] = ['apple', 'orange', 'lichi', 'banana'];
if(fruitsArray.includes('orange')){
console.log('orange is present in array');
}
输出:
"orange is present in array"
includes()
方法区分大小写,因此我们在传递值进行搜索时应该小心。另一个参数确定从数组中的哪个索引开始搜索。
下面的代码段演示了这一点。
var fruitsArray : string[] = ['apple', 'orange', 'lichi', 'banana'];
if(!fruitsArray.includes('orange', 2)){
console.log('orange is not present in array after index = 2');
}
输出:
"orange is not present in the array after index = 2"
使用 indexOf()
方法检查 TypeScript 数组中是否存在字符串
indexOf()
方法的行为类似于 includes()
方法。它们的区别在于搜索算法,它通过严格相等运算符或 ===
检查相等性。
以下代码段显示了如何查找数组中某个元素的所有匹配项。
var fruitsArray : string[] = ['apple', 'orange', 'lichi', 'orange', 'banana'];
var targetFruit : string = 'orange';
var foundIndices : number[] = [];
let idx : number = fruitsArray.indexOf(targetFruit);
while(idx !== -1){
foundIndices.push(idx);
idx = fruitsArray.indexOf(targetFruit, idx + 1);
}
console.log(foundIndices);
输出:
[1, 3]
indexOf()
和 includes()
方法都是相似的。但是,在 indexOf()
的情况下检查 NaN
的存在失败,而 includes()
成功确定它的存在。
另一个区别是这两种方法的返回类型,分别是元素第一次出现的索引和布尔值。
参考下面的代码。
var numbers : number[] = [ 1, 2, NaN, 3];
console.log(numbers.includes(NaN));
console.log(numbers.indexOf(NaN));
输出:
true
-1
使用 find()
方法检查 TypeScript 数组中是否存在字符串
如果成功执行,find()
方法返回目标元素的第一次出现,否则返回 undefined
。
var fruitsArray : string[] = ['apple', 'orange', 'lichi', 'banana'];
if(fruitsArray.find(e => e === 'orange')){
console.log('orange is present in array');
}
输出:
"orange is present in array"
使用 some()
方法检查 TypeScript 数组中是否存在字符串
some()
方法检查数组中是否至少有一个元素通过了传递给它的谓词函数。以下代码段演示了它如何在数组中搜索字符串。
var fruitsArray : string[] = ['apple', 'orange', 'lichi', 'banana'];
const search = (targetElement : string) => (arrElement : string) => arrElement === targetElement;
console.log(fruitsArray.some(search('orange')));
console.log(fruitsArray.some(search('grapes')));
输出:
true
false
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe