TypeScript에서 배열이 포함된 맵 초기화
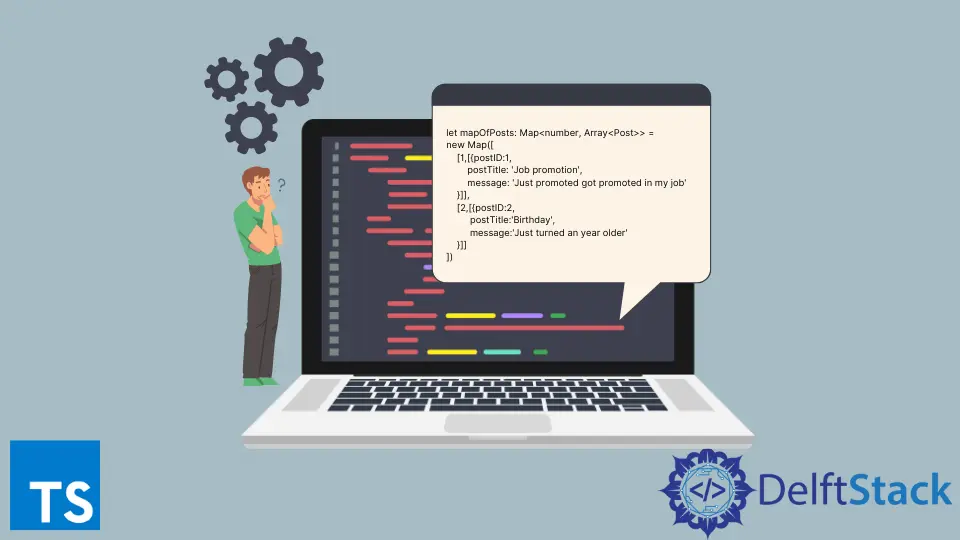
Map은 키-값 쌍의 형태로 데이터를 유지하는 데이터 구조입니다. 키와 값 모두에 원하는 모든 데이터 유형을 사용할 수 있습니다.
이 튜토리얼에서는 숫자를 키로 사용하고 사용자 정의 유형의 배열을 값으로 사용하는 맵을 만드는 방법을 배웁니다.
TypeScript의 Array
클래스를 사용하여 배열이 포함된 맵 초기화
Visual Studio Code로 이동하여 map-initialization
이라는 폴더를 만들거나 원하는 이름을 사용합니다. 폴더 아래에 array-class-map.ts
라는 파일을 만듭니다.
다음 코드를 복사하여 파일에 붙여넣습니다.
type Post = {
postID: number
postTitle: string
message: string
}
let mapOfPosts: Map<number, Array<Post>> =
new Map([
[1,[{postID:1,
postTitle: 'Job promotion',
message: 'Just promoted got promoted in my job'
}]],
[2,[{postID:2,
postTitle:'Birthday',
message:'Just turned an year older'
}]]
])
mapOfPosts.forEach((post) => console.log(post))
위의 코드에서 우리는 Map에서 값으로 사용할 Post
라는 사용자 정의 개체를 정의했습니다.
Map은 일반적이기 때문에 꺾쇠 괄호의 첫 번째 매개변수는 키가 숫자
유형임을 나타냅니다. 두 번째 매개변수는 Array<Post>
라는 다른 유형을 사용하여 값이 게시물 배열임을 나타냅니다.
Array
는 꺾쇠 괄호 안에 요소 유형을 지정하여 요소 배열을 생성하는 데 사용되는 일반 클래스입니다. 우리의 경우 꺾쇠 괄호 안에 요소 유형으로 Post
를 전달했습니다.
ForEach()
는 맵 뒤에서 요소 배열을 반복하고 콘솔에 출력하는 기능적 메서드입니다.
위의 예를 실행하려면 터미널로 이동하여 이 폴더의 위치로 cd
를 입력합니다. 다음 명령을 사용하여 tsconfig.json
파일을 생성합니다.
david@david-HP-ProBook-6470b:~/Documents/map-initialization$ tsc --init
tsconfig.json
파일에 다음 구성이 있는지 확인하십시오.
{
"compilerOptions":{
"target": "es5"
"noEmitOnError": true,
}
}
Map API는 es5
에 도입되었으므로 코드에서 컴파일 오류를 방지하기 위해 라이브러리를 추가했습니다.
다음 명령을 사용하여 TypeScript 코드를 node
를 사용하여 실행할 수 있는 JavaScript 코드로 변환합니다.
david@david-HP-ProBook-6470b:~/Documents/map-initialization$ tsc
코드가 컴파일되면 array-class-map.js
라는 파일이 생성됩니다. 다음 명령을 사용하여 JavaScript 파일을 실행합니다.
david@david-HP-ProBook-6470b:~/Documents/map-initialization$ node array-class-map.js
출력:
[
{
postID: 1,
postTitle: 'Job promotion',
message: 'Just promoted got promoted in my job'
}
]
[
{
postID: 2,
postTitle: 'Birthday',
message: 'Just turned an year older'
}
]
TypeScript에서 대괄호 []
를 사용하여 배열이 포함된 맵 초기화
이 예에서는 배열에 대한 기본적인 이해가 있는 개발자라도 대부분의 개발자가 알고 있는 기본 방법을 사용합니다.
같은 폴더 아래에 maps.ts
라는 파일을 만듭니다. 다음 코드를 복사하여 파일에 붙여넣습니다.
type Post = {
postID: number
postTitle: string
message: string
}
let mapOfPosts: Map<number, Post[]> =
new Map([
[1,[{postID:1,
postTitle: 'Job promotion',
message: 'Just promoted got promoted in my job'
}]],
[2,[{postID:2,
postTitle:'Birthday',
message:'Just turned an year older'
}]]
])
mapOfPosts.forEach((post) => console.log(post))
위의 코드에서 number
유형의 키와 Post
배열을 값으로 허용하는 Map을 정의했습니다.
배열을 생성하는 기본 방법은 Post[]
로 표시된 대로 대괄호 다음에 오는 유형을 정의하는 것입니다. Map은 new
키워드를 사용하여 한 줄로 초기화되었으며 두 개의 구체적인 요소가 Map에 추가되었습니다.
이 예제는 대괄호를 사용하여 Map 값에 대한 게시물 배열을 생성한다는 점을 제외하고 이전 예제와 동일한 방식으로 작동합니다.
이 예를 실행하려면 이전 예와 동일한 접근 방식을 사용하여 다음 출력을 얻습니다.
출력:
[
{
postID: 1,
postTitle: 'Job promotion',
message: 'Just promoted got promoted in my job'
}
]
[
{
postID: 2,
postTitle: 'Birthday',
message: 'Just turned an year older'
}
]
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub