How to Sorting Array in TypeScript
-
Use the
sort()
Method to Sort Array in TypeScript - Sorting Numeric Array in TypeScript
- Sorting Array of Objects in TypeScript
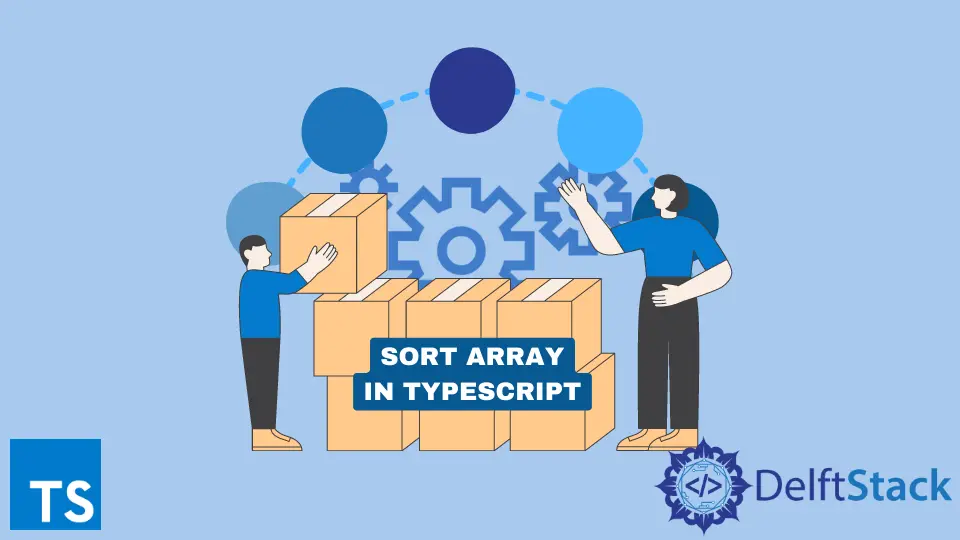
TypeScript allows different operations to be performed on the array, and sorting is one of the operations.
This tutorial will discuss the sorting of the array in typescript.
Use the sort()
Method to Sort Array in TypeScript
Sorting is done using the sort()
method. This method sorts the elements present in the array and returns the sorted string elements as an output to the user.
The sort()
method returns the result in ascending order by default. The array type is to be defined as string[]
.
Example:
const myArray: string[] = ['A', 'B', 'D', 'X', 'C', 'F'];
myArray.sort();
console.log(`My sorted array is = ${myArray}`);
Output:
My sorted array is = A,B,C,D,F,X
The sort()
method returns the string array, and the array elements are given in alphabetical order as an output. Now lets us take another example of a string array.
Example:
const myFruits: string[] = ['Apple', 'Peach', 'Strawberry', 'Banana', 'Mango'];
myFruits.sort();
console.log(`My sorted fruits is = ${myFruits}`);
Output:
My sorted fruits is = Apple,Banana,Mango,Peach,Strawberry
Notice all the fruits are sorted in alphabetical order.
Sorting Numeric Array in TypeScript
Sorting the numeric array in typescript is not as simple as it looks above because the sort()
method returns the string array. A logical operation is performed to sort the numeric array in ascending and descending order.
Example:
const numArray: number[] = [6,5,8,2,1];
numArray.sort();
console.log(`The sorted numebrs are = ${numArray}`);
Output:
The sorted numebrs are = 1,2,5,6,8
The above example works fine, but what happens if we add a two or three-digit number that has the last digit 0. Let’s take an example.
Example:
const numArray: number[] = [60,5,8,2,1];
numArray.sort();
console.log(`The sorted numebrs are = ${numArray}`);
Output:
The sorted numebrs are = 1,2,5,60,8
The above snippet shows that even though 60
is greater than 8
, it still comes first because the sort()
method returns an array of strings. In the string comparison, 60
comes before 8
.
To fix this issue, we have to use a comparison function in the sort()
method, and this function will decide whether we want to arrange the array in ascending order or descending order.
Example:
const numArray: number[] = [60,5,8,2,1];
numArray.sort(function(x,y) {
return x-y
});
console.log(`The sorted numebrs are = ${numArray}`);
Output:
The sorted numebrs are = 1,2,5,8,60
The results are displayed in ascending order. To display the results in descending order, follow the example below.
Example:
const numArray: number[] = [60,5,8,2,1];
numArray.sort(function(x,y) {
return y-x
});
console.log(`The sorted numebrs are = ${numArray}`);
Output:
The sorted numebrs are = 60,8,5,2,1
Now the results are displayed in descending order. The function(x,y)
operates on the logic of whether we want the numbers to be arranged in ascending or descending order.
Sorting Array of Objects in TypeScript
TypeScript allows us to sort the objects in the array.
Example:
var numArray: { num: number; }[] = [{ num: 7}, { num: 9 }, {num: 3}, {num: 6}, {num: 1}];
numArray.sort(function(x,y) {
if (x.num > y.num) {
return 1;
}
if (x.num < y.num) {
return -1;
}
return 0;
});
console.log('The sorted array of objects is as follow:');
console.log(numArray);
Output:
The sorted array of objects is as follow:
[{
"num": 1
}, {
"num": 3
}, {
"num": 6
}, {
"num": 7
}, {
"num": 9
}]
The code example shows the sorted array of objects. In the conditions, return 1
shows that the first go after the second.
The return -1
shows the first go before the second, and return 0
shows they are equivalent. The result is shown in ascending order.
To get the result in descending order, replace the -1
with 1
in the condition and vice versa.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn