How to Create an Empty Array in TypeScript
- Create a New Project
- Generate TypeScript Configuration File
- Create a Class Type for the Array
- Use Explicit Type Declaration to Create an Empty Array in TypeScript
- Use Type Assertion to Create an Empty Array in TypeScript
- Use the Array Constructor to Create an Empty Array in TypeScript
- Conclusion
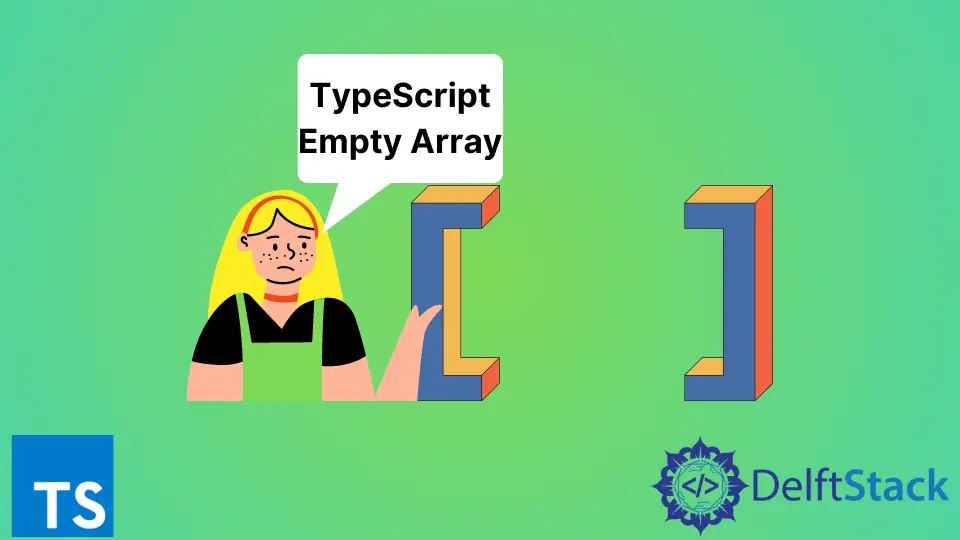
The array is a data structure that stores elements using the linear approach. This is because the order of the elements is sequential, and we can access the previous and next elements of the current element.
Arrays are commonly used when developing applications compared to other data structures. For example, when we want to fetch a collection of data items from a database or a service and display them to an application, we usually use an array to achieve this.
We first declare an array for the type fetched from the source and subscribe the array to an observable. The observable adds the items fetched from our source to the array, and then the array items are displayed on the application.
This tutorial will show us how to create an empty array in TypeScript that can later be populated with data.
Create a New Project
Open WebStorm IDEA and select File > New > Project
. On the frame that opens, select the Node.js
section located on the left side of the frame.
Change the project name from untitled
to empty-array
on the Location
section on the right side.
The node
runtime environment must be installed before creating this project so that the Node interpreter
and Package manager
sections can be read automatically from the system.
Generate TypeScript Configuration File
Once the project has been generated, open a new terminal window using the shortcut ALT+F12 and use the following command to generate the tsconfig.json
file.
~/WebstormProjects/empty-array$ tsc --init
Output:
{
"compilerOptions": {
"target": "es5",
"module": "commonjs",
"noEmitOnError": true
}
}
The only changes we have made to the configuration file is adding the noEmitOnError: true
property that ensures no JavaScript file is generated in case of a transpile error.
Create a Class Type for the Array
Create a file named Phone.js
under the empty-array
folder and copy and paste the following code into the file.
export class Phone{
constructor(private id: number,
private make: string,
private name: string,
private price: number) {
}
}
In this code, we’ve created a class named Phone
that contains the properties id
, make
, name
, and price
. We will utilize this class as the type of our array but be free to use any data type such as string or number.
The export
keyword indicates that this file is a module, and the Phone
class will be used in another application module, as shown in the following section.
Use Explicit Type Declaration to Create an Empty Array in TypeScript
Create a file named Main.js
under the folder empty-array
and copy and paste the following code into the file.
import {Phone} from "./Phone";
function usingExplicitType(): Phone[]{
return [];
}
function main(){
if (usingExplicitType().length == 0){
console.log("The array has no phone instances");
}
}
main();
In this code, we’ve created a method named usingExplicitType()
that returns an array of phone objects Phone[]
. Since we want to return an empty array, the code inside the method returns an empty array explicitly without creating any objects.
Since we know the method returns an array, we can call the length
property on it to check its length. If the array’s length equals 0, then the array has no elements.
Note that we have used the import
keyword to use the Phone
class in this module.
To verify that the code works as expected, use the following command to execute the main()
function that defines our concrete implementation.
~/WebstormProjects/empty-array$ tsc && node Main.js
The tsc
command transpile the TypeScript code to JavaScript code, and the node
command executes the Main.js
file. Ensure the output is as shown below.
The array has no phone instances
Use Type Assertion to Create an Empty Array in TypeScript
Copy and paste the below code into the Main.js
file after the usingExplicitType()
method.
function usingTypeAssertion(): Phone[]{
return [] as Phone[]; // return <Phone[]>[];
}
function main(){
if (usingTypeAssertion().length == 0){
console.log("The array has no phone instances");
}
}
main();
In this code, we’ve created a method named usingTypeAssertion()
that returns an empty array of phone objects Phone[]
.
This method uses type assertion to achieve our objective of returning an empty array of phone objects. The type assertion is indicated using the as
keyword.
The main()
method works the same way as the previous example.
Run this code using the command used in the previous example and ensure the output is as shown below.
The array has no phone instances
Use the Array Constructor to Create an Empty Array in TypeScript
Copy and paste the below code into the Main.js
file after the usingTypeAssertion()
method.
function usingArrayConstructor(): Phone[]{
return new Array<Phone>();
}
function main(){
if (usingArrayConstructor().length == 0){
console.log("The array has no phone instances");
}
}
main();
In this code, we’ve created a method named usingArrayConstructor()
that returns an array of phone objects Phone[]
as we have done in the previous examples.
Since the array in TypeScript is an object, we invoke its constructor new Array<Phone>()
using the new
keyword without passing any elements to it to return an empty array. Note that since the Array
interface uses one of its generic implementations to achieve this, we have passed Phone
as the argument of the generic parameter to ensure only empty phone objects are returned.
Run this code using the command used in the previous example and ensure the output is as shown below.
The array has no phone instances
Conclusion
This tutorial taught us how to create an empty array in TypeScript. The approaches we have covered include using explicit type declaration, type assertion, and an array constructor.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub