How to Define an Array in TypeScript Interface
- Create a New Project
- Generate TypeScript Configuration File
- Create the JSON File for Our Data
-
Define a
Book
Class - Define an Interface for an Array of Books
- Consume the JSON Data Using an Array
- Conclusion
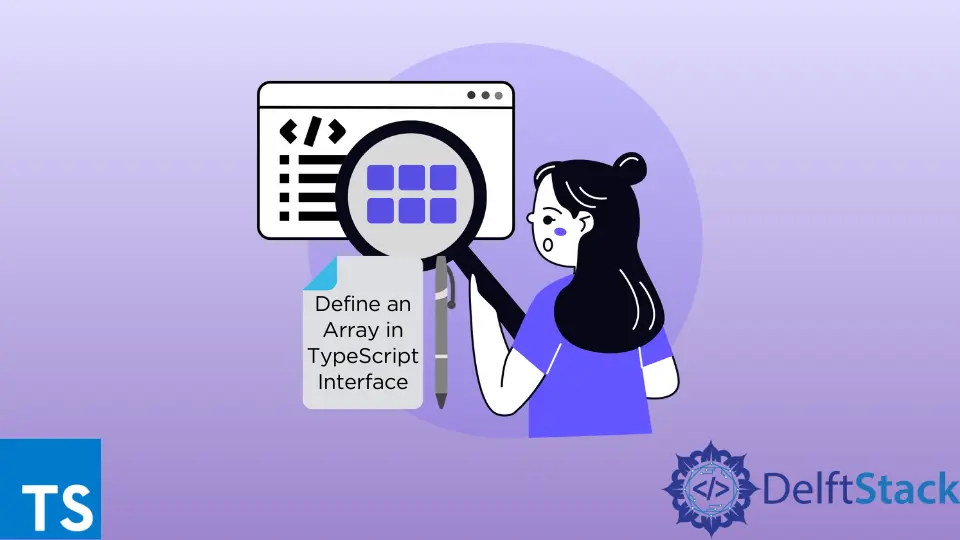
Creating an object representation of JSON data is a common development approach for developers using NoSQL databases. The reason for this is that NoSQL databases use JSON to store data.
These data can be represented in the programming language using different types of data types, such as strings, numbers, and custom objects, depending on the state of the data.
In this tutorial, we will learn how to create an array in an interface and populate this array with data stored in a JSON file. The JSON file contains an array of data, and these data will get mapped to custom TypeScript objects in our application.
Create a New Project
Open WebStorm IDEA and select File > New > Project
. Select Node.js
on the left of the window that opens, and change the project name from untitled
to array-of-strings
or enter any name preferred.
The node
runtime environment should be installed before creating the project so that the Node interpreter
and Package manager
sections can be filled automatically for you. Finally, press the Create
button to generate the project.
Generate TypeScript Configuration File
Once the project has been generated, open a new terminal window using the keyboard shortcut ALT+F12 and use the following command to generate the tsconfig.json
file.
~/WebstormProjects/array-of-strings$ tsc --init
Open tsconfig.json
and add the properties noEmitOnError: true
and resolveJsonModule: true
. The first property prevents the JavaScript files from being generated if there is a transpile error, and the second property will allow us to read the JSON file containing our data.
{
"compilerOptions": {
"target": "es5",
"module": "commonjs",
"noEmitOnError": true,
"resolveJsonModule": true,
"strict": true,
"noFallthroughCasesInSwitch": true,
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true
}
}
Create the JSON File for Our Data
Create a file named books.json
under the folder array-of-strings
and copy and paste the following JSON data into the file.
[
{
"name": "Introduction to Java",
"author": "john doe"
},
{
"name": "Advanced databases",
"author": "peter parker"
}
]
The JSON data holds an array of objects, and each object has the property name
and author
. In simple terms, each object in the JSON data represents a Book
instance which will be defined in the next section.
Define a Book
Class
Create a file named Book.ts
under the array-of-strings
folder and copy and paste the following code into the file.
class Book{
constructor(private name: string,private author: string) {
this.name = name
this.author = author
}
}
In this code, we have defined a Book
class with the properties name
and author
, which are the same properties in the JSON data. In the next section, we will use the Book
class to define an array of books in an interface.
Define an Interface for an Array of Books
Create a file named BookService.ts
under the array-of-books
folder and copy and paste the following code into the file.
export interface BookService{
books: Book[];
}
In this file, we have created an interface named BookService
that defines an array of books using the name books
. Note that any class that will implement this interface will have to implement the books
array, and the next section shows how this is done.
Consume the JSON Data Using an Array
Create a file named BookServiceImpl.ts
under the array-of-books
folder and copy and paste the following code into the file.
import bookSource from "./books.json"
import {BookService} from "./BookService";
class BookServiceImpl implements BookService{
books: Book[] = JSON.parse(JSON.stringify(bookSource));
logBooks(): void{
this.books.forEach(book => console.log(book));
}
}
function main(){
const bookService = new BookServiceImpl();
bookService.logBooks();
}
main();
In this file, we created a class named BookServiceImpl
that implements the BookService
. Note that we must implement the books
array, a requirement mentioned in the previous section.
The array consumes the data in the books.json
file, and we access the data using the import
statement with the name bookSource
. The stringify()
method converts the bookSource
to a JSON string, and the parse()
method uses the returned JSON string to create an array of books.
The logBooks()
method uses the forEach()
method to log the array of book objects to the console for testing purposes. The main()
method generates an instance of the BookServiceImpl
class and invokes the logBook()
method using this instance.
Run this code using the following command.
~/WebstormProjects/array-of-strings$ tsc && node BookServiceImpl.js
The tsc
command transpile the TypeScript files to JavaScript files, and the node
command executes the BookServiceImpl.js
file. Ensure the output is as shown below.
{ name: 'Introduction to Java', author: 'john doe' }
{ name: 'Advanced databases', author: 'peter parker' }
Conclusion
In this article, we’ve learned how to define an array in a TypeScript interface and use the array to consume data from a JSON file. Note that when developing an application that interacts with users, we usually use a database, not a file.
We have used a custom object in this tutorial, but you can use other types, including strings, numbers, and others.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub