How to Sort Array of Objects in TypeScript
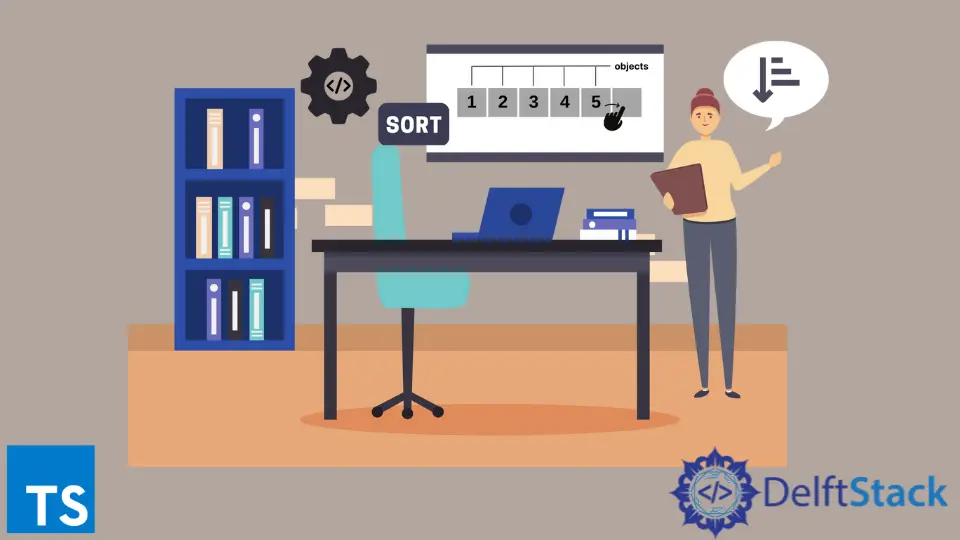
This article shows how to sort an array of objects in TypeScript.
TypeScript Objects
TypeScript is a strongly typed language. It supports various types such as primitives, arrays, and object types.
The object type is a special type that can represent data with properties. A TypeScript object looks like a JSON object, as shown in the following.
{ id: 001, username: 'rick' }
The id
and username
are the two properties of the above object. The values have been assigned to each property too.
In TypeScript, custom object types can be created using interfaces. The interfaces allow you to create object structures, as shown in the following.
interface employee {
empId: number,
empDesignation: string
}
The above employee
interface defines an employee
object with two properties, empId
and empDesignation
, which are number and string types, respectively.
TypeScript Array of Objects
TypeScript supports array types that can store a collection of elements. It can store both primitive type values and custom objects as well.
Let’s create an array of employee
objects.
let employeesList: Array<employee> = [
{empId: 20, empDesignation: 'senior engineer'},
{empId: 14, empDesignation: 'junior engineer'},
{empId: 25, empDesignation: 'program manager'},
{empId: 12, empDesignation: 'director'}
]
The above array employeesList
contains four employee
objects. Sometimes, we need to sort these objects by a given property.
It is like SQL orders data by a column or multiple columns. TypeScript supports sorting out of the box.
The in-built sort()
method can sort an array of objects.
the sort()
Method in TypeScript
The sort
method takes an array of elements and returns the sorted array. It accepts an optional function that can be used to specify the sort order of the returned array.
Syntax:
//1.
Array.sort()
//2.
Array.sort(<custom_function>)
The Array
is the array of objects we will sort. The <custom_function>
can specify the sort order.
Since the requirement is to sort an array of objects by one of its properties, we have to use the sort
method by passing a custom function that decides the sort order and object property.
Let’s sort the array by property id
.
employeesList.sort(
(firstObject: employee, secondObject: employee) =>
(firstObject.empId > secondObject.empId) ? 1 : -1
);
The arrow function has been used in the above example to specify the sort condition.
Whenever the function returns 1
, that means the secondObject
has the higher sort precedence over the firstObject
. Else, the firstObject
takes the higher sort precedence.
This would sort the employeesList
objects by their empId
property in ascending order.
Output:
The same array can be sorted in descending order with the below condition inside the arrow function.
(firstObject: employee, secondObject: employee) =>
(firstObject.empId > secondObject.empId) ? -1 : 1
There are some scenarios where you must first sort by the empId
and then empDesignation
property. If the empId
property value is the same for a given object, we can check for the empDesignation
property in the conditional operator.
let employeesList: Array<employee> = [
{empId: 20, empDesignation: 'senior engineer'},
{empId: 14, empDesignation: 'junior engineer'},
{empId: 14, empDesignation: 'developer'},
{empId: 25, empDesignation: 'program manager'},
{empId: 12, empDesignation: 'director'}
]
employeesList.sort(
(firstObject: employee, secondObject: employee) =>
(firstObject.empId > secondObject.empId) ? 1 :
(firstObject.empId === secondObject.empId) ? (
(firstObject.empDesignation > secondObject.empDesignation) ? 1 : -1 )
: -1
);
Output:
As we can see, two objects have the same empId
14
. Hence, those two objects have been sorted by the empDesignation
property.
The sort
method is very powerful when ordering objects based on their properties. It can be used for complex type sorting too.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.