Default Value for Object Parameters in TypeScript
- Default Values for Objects in TypeScript
- Pass Default Values Through Destructuring in TypeScript
- Make Certain Attributes of an Object Have Default Values in TypeScript
-
Use the
Partial
Keyword to Make All Fields Have Default Values in TypeScript
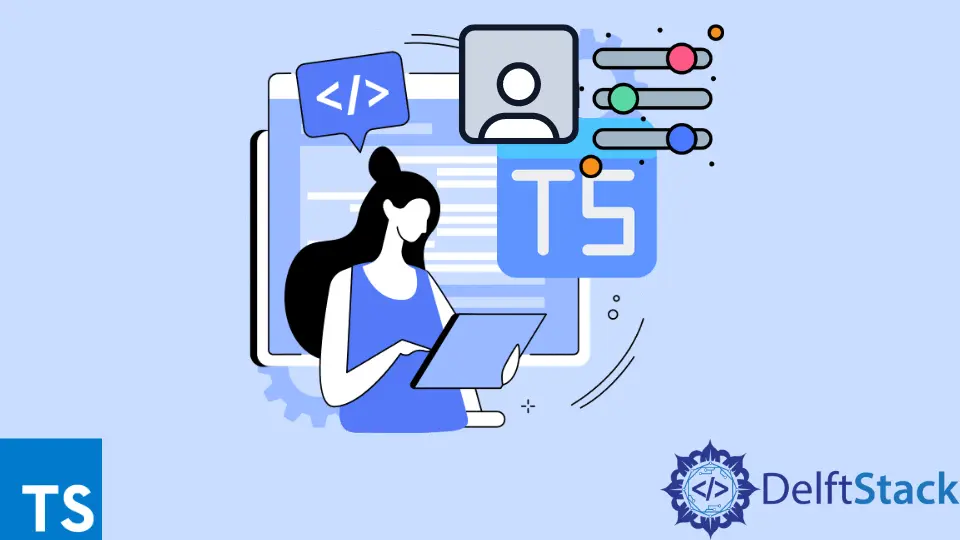
Default parameters are something that a user wants to always incorporate into the code. TypeScript has a facility for providing default parameters in objects and passing objects in functions.
This tutorial will focus on how default parameters can be used in TypeScript.
Default Values for Objects in TypeScript
A function can be defined to work with default values if, in case, no value is passed to the function. The following code segment demonstrates how this can be achieved.
interface Config {
file : string ;
test : boolean;
production : boolean;
dbUrl : string;
}
function getConfig ( config : Config = { file : 'test.log', test : true,
production : false, dbUrl : 'localhost:5432' }) {
console.log(config);
}
getConfig();
getConfig({file : 'prod.log', test: false, production : true, dbUrl : 'example.com/db'});
Output:
{
"file": "test.log",
"test": true,
"production": false,
"dbUrl": "localhost:5432"
}
{
"file": "prod.log",
"test": false,
"production": true,
"dbUrl": "example.com/db"
}
The above shows how default values can be used in TypeScript.
The getConfig
function uses the default values assigned in the function definition if no values for the Config
object are passed while calling the function, which can be seen in the case of the first call to the getConfig
function.
In the second call to the function, the default values are overridden by the values passed to the function.
Pass Default Values Through Destructuring in TypeScript
TypeScript also has the support for passing down default values to function by destructuring the attributes of an object. The following code segment shows how it can be achieved.
interface Person {
firstName : string ;
lastName : string ;
}
function getPerson( {firstName = 'Steve', lastName = 'Jobs' } : Person ) {
console.log( firstName + ' ' + lastName);
}
getPerson({} as Person);
getPerson({ firstName : 'Tim', lastName : 'Cook'});
Output:
"Steve Jobs"
"Tim Cook"
Thus like the previous examples, there are two calls to the function getPerson
, one using the default values while the other using the values passed on the function. The difference is for the default case, an empty object {}
is to be passed, or TypeScript will throw an error - Expected 1 arguments, but got 0
.
Make Certain Attributes of an Object Have Default Values in TypeScript
We can make certain attributes of an object have default values while the others are required while assigning attributes to the object or passing the object to a function. This can be done by using the ?
operator.
The following code segment shows how it can be done.
interface Config {
file? : string ;
test? : boolean;
production : boolean;
dbUrl : string;
}
function getConfig( { file = 'api.log', test = true, production, dbUrl} : Config ) {
var config : Config = {
file,
test,
production,
dbUrl
};
console.log(config);
}
getConfig({production : true, dbUrl : 'example.com/db'});
Output:
{
"file": "api.log",
"test": true,
"production": true,
"dbUrl": "example.com/db"
}
Use the Partial
Keyword to Make All Fields Have Default Values in TypeScript
The Partial
keyword is a useful tool to make all the attributes of an object optional and can be used to make all the fields of an object have default values in the case of a function definition. Use the example from above.
interface Config {
file : string ;
test : boolean;
production : boolean;
dbUrl : string;
}
function getConfig( { file = 'api.log', test = true, production = false, dbUrl = 'localhost:5432'} : Partial<Config> ) {
var config : Config = {
file,
test,
production,
dbUrl
};
console.log(config);
}
getConfig({production : true});
Output:
{
"file": "api.log",
"test": true,
"production": true,
"dbUrl": "localhost:5432"
}
Thus in the example above, we can see all the fields have default values, and the value for the production
attribute has been overridden.