How to Merge Objects TypeScript
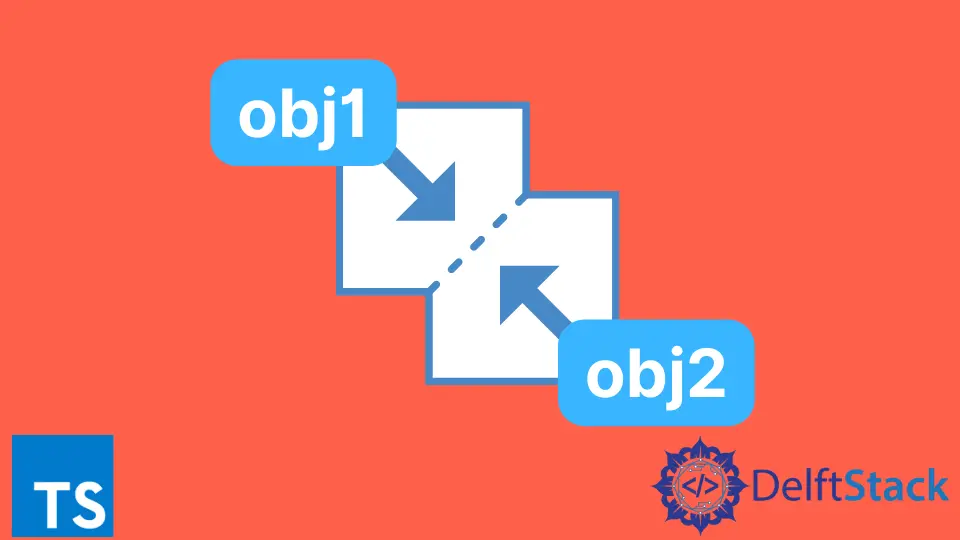
We will introduce a simple way to merge two or more objects into a single object and use all their properties in TypeScript with an example.
Merge Objects in TypeScript
When working with an application whose data is mostly stored in objects or getting some data from APIs in the form of objects, there are situations in which we need to merge some objects. We always want to simplify the problems in a short problem that can be easily solved or a solution that can be easily used without any data loss.
When we have multiple objects that could be used as a single object, we need to merge them to decrease a load of multiple objects into one for ease of use.
In this tutorial, we will go through two objects that will contain different values but need to be merged because they contain the same type of data we need to display on the frontend or save in the database. Let’s create two objects, languageOne
and languageTwo
, containing the programming languages shown below.
# typescript
var languageOne = {
PLA: 'Angular',
PLB: 'Bash',
PLC: 'CPlusPlus',
PLR: 'React'
};
var languageTwo = {
PLD: 'Dart',
PLE: 'Express',
PLF: 'FSharp',
PLS: 'SWIFT'
};
Now, if we want to combine these two objects into a single object and use that object to get the values based on the keys, we can use the following method as shown below.
# typescript
var languages = {...languageOne, ...languageTwo};
Using this line of code, we assign both objects to a single object. We can easily call the properties of any object by using the dot
and calling the property by its key, as shown below.
# typescript
console.log(languages.PLA);
console.log(languages.PLS);
Output:
As we can see from the above example, with just one line of code, we can merge as many objects as we want into a single object. But still, there is one essential question that we shouldn’t skip.
What will happen if both objects have one or more same properties?
Let’s go through another example in which we will use both objects, but we will add some properties that will exist in both objects, and let’s check how it works. The objects that we will be using are shown below.
# typescript
var languageOne = {
PLA: 'Angular',
PLB: 'Bash',
PLC: 'CPlusPlus',
PLR: 'React',
PLF: 'Flex'
};
var languageTwo = {
PLD: 'Dart',
PLE: 'Express',
PLF: 'FSharp',
PLS: 'SWIFT',
PLC: 'CSharp'
};
Now, let’s merge these objects into another using the same method we used above, as shown below.
# typescript
var languages = {...languageOne, ...languageTwo};
Now, let’s call PLC
and PLF
properties using the same method we used in the above example.
# typescript
console.log(languages.PLC);
console.log(languages.PLF);
Output:
As we can see from the above example, we got the properties from the second object only. Now, let’s reverse the objects and check the result as shown below.
# typescript
var languages = {...languageTwo, ...languageOne};
console.log(languages.PLC);
console.log(languages.PLF);
Output:
As we can see from the above example, while merging the two objects, the properties present in both objects are updated based on the order of the objects in which we are merging them. So, if we have some unique data and want to keep the whole data without updating the properties, it is not possible with this method.
But if we want to update the properties based on the order of objects, we can use this method, which will work great for you.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn