TypeScript 中对象参数的默认值
Shuvayan Ghosh Dastidar
2023年1月30日
TypeScript
TypeScript Object
- TypeScript 中对象的默认值
- 在 TypeScript 中通过解构传递默认值
- 在 TypeScript 中使对象的某些属性具有默认值
-
在 TypeScript 中使用
Partial
关键字使所有字段都具有默认值
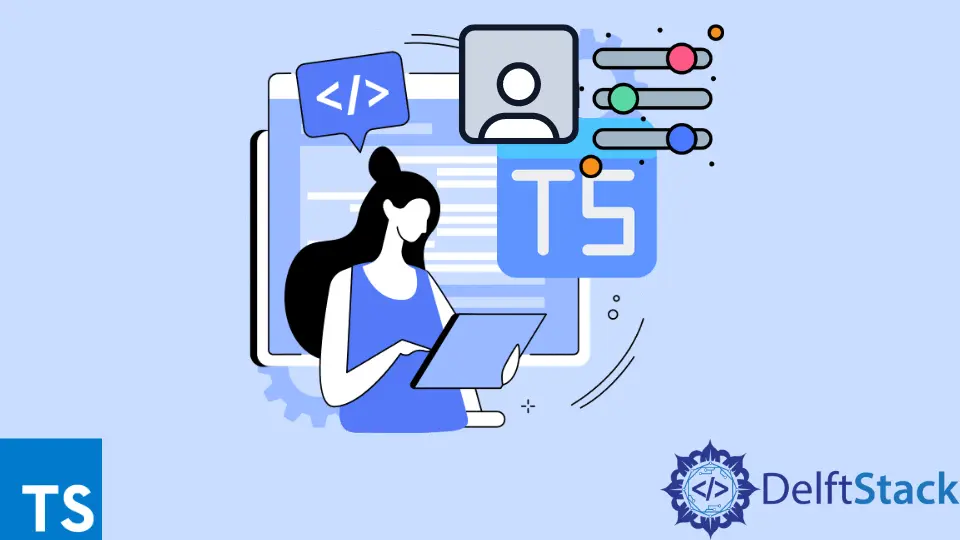
默认参数是用户希望始终合并到代码中的东西。TypeScript 具有在对象中提供默认参数和在函数中传递对象的功能。
本教程将重点介绍如何在 TypeScript 中使用默认参数。
TypeScript 中对象的默认值
如果没有值传递给函数,则可以将函数定义为使用默认值。以下代码段演示了如何实现这一点。
interface Config {
file : string ;
test : boolean;
production : boolean;
dbUrl : string;
}
function getConfig ( config : Config = { file : 'test.log', test : true,
production : false, dbUrl : 'localhost:5432' }) {
console.log(config);
}
getConfig();
getConfig({file : 'prod.log', test: false, production : true, dbUrl : 'example.com/db'});
输出:
{
"file": "test.log",
"test": true,
"production": false,
"dbUrl": "localhost:5432"
}
{
"file": "prod.log",
"test": false,
"production": true,
"dbUrl": "example.com/db"
}
上面显示了如何在 TypeScript 中使用默认值。
如果在调用函数时没有传递 Config
对象的值,getConfig
函数将使用函数定义中分配的默认值,这可以在第一次调用 getConfig
函数的情况下看到。
在对函数的第二次调用中,默认值被传递给函数的值覆盖。
在 TypeScript 中通过解构传递默认值
TypeScript 还支持通过解构对象的属性将默认值传递给函数。以下代码段显示了它是如何实现的。
interface Person {
firstName : string ;
lastName : string ;
}
function getPerson( {firstName = 'Steve', lastName = 'Jobs' } : Person ) {
console.log( firstName + ' ' + lastName);
}
getPerson({} as Person);
getPerson({ firstName : 'Tim', lastName : 'Cook'});
输出:
"Steve Jobs"
"Tim Cook"
因此,与前面的示例一样,有两次调用函数 getPerson
,一次使用默认值,另一次使用传递给函数的值。不同之处在于默认情况下,要传递一个空对象 {}
,否则 TypeScript 将抛出错误 - Expected 1 arguments, but got 0
。
在 TypeScript 中使对象的某些属性具有默认值
在为对象分配属性或将对象传递给函数时,我们可以使对象的某些属性具有默认值,而其他属性是必需的。这可以通过使用 ?
来完成运算符。
以下代码段显示了它是如何完成的。
interface Config {
file? : string ;
test? : boolean;
production : boolean;
dbUrl : string;
}
function getConfig( { file = 'api.log', test = true, production, dbUrl} : Config ) {
var config : Config = {
file,
test,
production,
dbUrl
};
console.log(config);
}
getConfig({production : true, dbUrl : 'example.com/db'});
输出:
{
"file": "api.log",
"test": true,
"production": true,
"dbUrl": "example.com/db"
}
在 TypeScript 中使用 Partial
关键字使所有字段都具有默认值
Partial
关键字是一个有用的工具,可以使对象的所有属性成为可选的,并且可用于在函数定义的情况下使对象的所有字段都具有默认值。使用上面的示例。
interface Config {
file : string ;
test : boolean;
production : boolean;
dbUrl : string;
}
function getConfig( { file = 'api.log', test = true, production = false, dbUrl = 'localhost:5432'} : Partial<Config> ) {
var config : Config = {
file,
test,
production,
dbUrl
};
console.log(config);
}
getConfig({production : true});
输出:
{
"file": "api.log",
"test": true,
"production": true,
"dbUrl": "localhost:5432"
}
因此,在上面的示例中,我们可以看到所有字段都有默认值,并且 production
属性的值已被覆盖。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe