How to Convert Array to String in Ruby
-
Use
to_s
to Convert an Array to a String in Ruby -
Use
inspect
to Convert an Array to a String in Ruby -
Use
join
to Convert an Array to a String for Complex Output Format in Ruby -
Use the
*
(Splat) Operator to Convert an Array to a String for Complex Output Format in Ruby - Conclusion
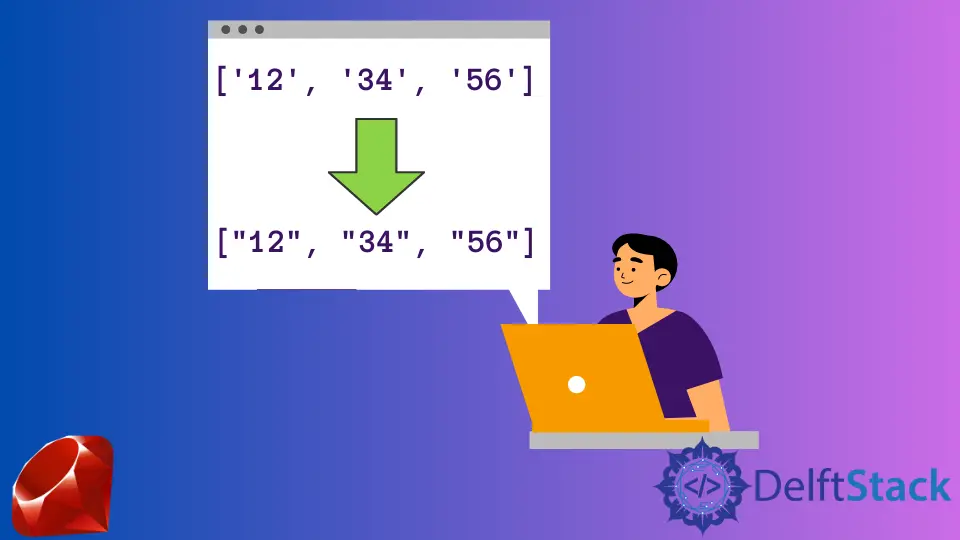
Ruby, known for its elegance and flexibility, provides several methods to convert an array into a string.
This article explores various techniques, discussing their use cases and nuances.
Use to_s
to Convert an Array to a String in Ruby
In Ruby, the to_s
method serves as a fundamental tool for converting various objects, including arrays, into their string representations.
The syntax of the to_s
method is pretty straightforward:
object.to_s
Here, object
represents an instance of any Ruby class. When invoked on an array, to_s
transforms the array into a string, encapsulating its elements within square brackets.
It’s important to note that the to_s
method, while convenient, does not provide options for customizing the separator between elements.
Let’s walk through a complete working example to illustrate the application of the to_s
method:
array = [1, 2, 3]
result = array.to_s
puts result
In this example, we start by defining an array array
containing the elements 1
, 2
, and 3
. The magic happens when we invoke the to_s
method on this array.
It seamlessly transforms the array into a string representation, encapsulating the elements within square brackets. A simple puts
statement outputs the converted string to the console.
Upon running this code, the output will be:
[1, 2, 3]
This shows the default behavior of the to_s
method when applied to an array. It provides a quick and convenient way to obtain a string representation of the entire array.
While powerful, keep in mind that if customization of the separator or individual element transformations is required, alternative methods like join
or string interpolation may be more suitable.
Use inspect
to Convert an Array to a String in Ruby
Another array-to-string conversion method we can use is the inspect
method. While to_s
provides a basic string representation, inspect
offers a more detailed view of an object, including arrays.
The syntax of the inspect
method is as follows:
object.inspect
Much like to_s
, inspect
is a default method available for all Ruby objects. When applied to an array, it provides a more comprehensive string representation, including detailed information about each element.
Here’s a complete code example showcasing the use of the inspect
method:
array = [1, 'hello', :symbol, [4, 5, 6]]
result = array.inspect
puts result
In this example, we initialize an array array
with a diverse set of elements, including integers, strings, symbols, and even another nested array. The crucial step is invoking the inspect
method on this array.
Unlike to_s
, inspect
doesn’t just stop at encapsulating the elements within square brackets; it goes further by providing detailed information about each element.
When you run this code, the output will be:
[1, "hello", :symbol, [4, 5, 6]]
Here, the inspect
method meticulously includes the type of each element and, in the case of nested arrays, recursively applies itself to maintain a clear and informative representation.
The inspect
method is a valuable tool when you need not only a string representation of an array but also additional insights into the array’s structure. Its detailed output makes it particularly useful for debugging and understanding the composition of complex arrays.
Use join
to Convert an Array to a String for Complex Output Format in Ruby
An alternative way to convert an array to a string in Ruby is using the join
method. This enables us to tailor the string representation to our exact specifications.
The join
method in Ruby is designed to concatenate elements of an array into a single string using a specified separator. The syntax is quite simple:
array.join(separator)
Here, array
is the array we want to convert, and separator
is the string that will be placed between each pair of elements in the final string.
Let’s put theory into practice with a simple code example:
array = [12, 23, 34]
result = array.join(', ')
puts result
In this example, we start by defining an array array
with elements 12
, 23
, and 34
. With just one line of code, we concatenate the array elements into a string, separated by a comma and a space (", "
) using the join
method.
The resulting string is stored in the variable result
. A straightforward puts
statement outputs the converted string to the console.
Executing this code yields the following output:
12, 23, 34
Use the *
(Splat) Operator to Convert an Array to a String for Complex Output Format in Ruby
The *
(splat) operator is also a concise and expressive way to convert arrays to strings. The splat operator, denoted by an asterisk (*
), is applied before an array to spread its elements.
In the context of array-to-string conversion, the syntax is straightforward:
array * separator
Here, array
represents the array we wish to convert, and separator
is the string that will be inserted between each pair of elements in the resulting string.
Let’s take a look at how the splat operator streamlines array-to-string conversion:
array = [1, 2, 3]
result = array * ', '
puts result
In this example, we initialize an array array
containing the elements 1
, 2
, and 3
. Also, with just one line of code, the *
operator spreads the array elements into a string, separated by a comma and a space (", "
).
Executing this code produces the following output:
1, 2, 3
Conclusion
Ruby’s array-to-string conversion methods provide a range of options to suit different scenarios. By understanding each method’s strengths and use cases, you can choose the one that best fits the requirements of your specific task, ensuring clean and efficient code in your Ruby projects.
Related Article - Ruby Array
- %i and %I in Ruby
- How to Combine Array to String in Ruby
- How to Square Array Element in Ruby
- How to Merge Arrays in Ruby
- How to Convert Array to Hash in Ruby
- How to Remove Duplicates From a Ruby Array