React Native Text Styles
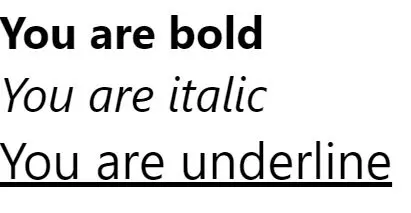
In React Native, we may want to make text bold, italic, or underlined. This article will look at creating text in React Native bold, italic, or underlined text.
React Native Text Styles
We may use styles in React Native to make text bold, italic, or underlined.
Syntax:
const styles = StyleSheet.create({
bold: {fontWeight: 'bold', fontSize: 30},
italic: {fontStyle: 'italic', fontSize: 32},
underline: {textDecorationLine: 'underline', fontSize: 34},
});
As you can see, we make a stylesheet that contains bold
, italic
, and underline
. The style fontWeight
is set as bold
to make the text bold.
Similarly, for italic, italic is a style of text that tells us the text is slanted towards the right. The style fontStyle
is set as italic
to make the text italic.
Likewise, to underline the text, we set the style of text by using textdecorationLine
as underline.
Code Example:
import React from 'react';
import {StyleSheet, Text, View} from 'react-native';
const App = () => {
return (
<View><Text style = {styles.bold}>You are
bold</Text>
<Text style={styles.italic}>You are italic</Text>
<Text style = {styles.underline}>You are underline</Text>
</View>);
};
const styles = StyleSheet.create({
bold: {fontWeight: 'bold', fontSize: 30},
italic: {fontStyle: 'italic', fontSize: 32},
underline: {textDecorationLine: 'underline', fontSize: 34},
});
export default App;
First, we import the requirements for this example from React Native. Then, the primary function creates a view component, including the text component.
To make text decorative, we have used some styles I mentioned in the previous code. We created a stylesheet containing the fontWeight
, fontStyle
, and text decoration lines as bold, italic, and underline, respectively.
Bold, italic, and underlined text should be sorted from top to bottom.
Output:
We may use styles in React Native to make text bold, italic, or underlined.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn