How to Set the Border Color in React-Native
-
Set the Border Color Using the
StyleSheet
in React-Native -
Set the Border Color Using the
style prop
in React-Native
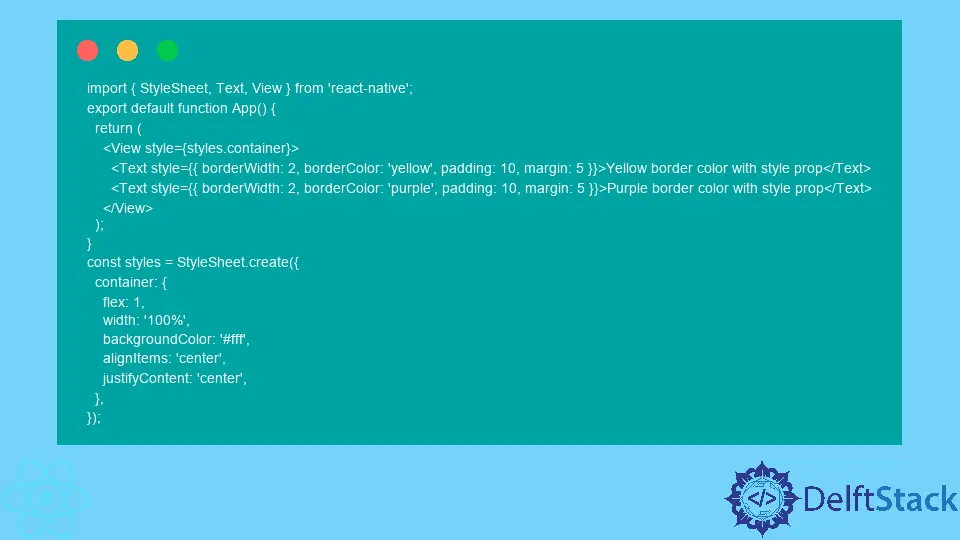
This article will teach us to set and use the border color in a react-native application. The border is the outer layer of an element that can be customized with different border widths and colors.
We can even set the colors for all four sides of an element. The react-native uses the Text
component to show any text in the application.
This article will use react-native to develop a text element’s border and border color. Different ways to set the border’s color in the react-native
exist; we will discuss the most popular ways for the same.
Set the Border Color Using the StyleSheet
in React-Native
The StyleSheet
needs to be imported from the react-native package. It is used to create styles
for different react-native elements.
Using it, we can create our custom styles and add them accordingly to our application.
Syntax of the StyleSheet
:
const styles = StyleSheet.create({
style1: {
// Put your style here
// For example:
backgroundColor: '#ffffff',
color: '#000000'
},
style2: {
// Put your style here
// For example:
width: '100%',
},
});
After declaring the StyleSheet
in our react-native application, we need to create a style
with the border
and border-color
properties and use it in a Text
.
In the below example, we use the StyleSheet
of react-native to set the border color of a Text
component. Firstly, we imported the StyleSheet
and created multiple styles
named redBorder
, blueBorder
, and greenBorder
.
Each style
contains some styling, such as borderWidth
and borderColor
. Then, we created three different Text
components and put our created styles
in them.
Finally, the padding
and margins
are added just for better visualization.
import { StyleSheet, Text, View } from 'react-native';
export default function App() {
return (
<View style={styles.container}>
<Text style={styles.redBorder}>It has red border color</Text>
<Text style={styles.blueBorder}>It has blue border color</Text>
<Text style={styles.greenBorder}>It has green border color</Text>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
width: '100%',
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
},
redBorder: {
borderWidth: 1,
borderColor: 'red',
padding: 10,
margin: 5
},
blueBorder: {
borderWidth: 1,
borderColor: 'blue',
padding: 10,
margin: 5
},
greenBorder: {
borderWidth: 1,
borderColor: 'green',
padding: 10,
margin: 5
}
});
Output:
In the above output, users can see three texts with three border colors: red, blue, and green.
Set the Border Color Using the style prop
in React-Native
The style prop
is used to set styles
for different elements of react-native. It takes an object containing the styles
the user wants to apply in the component.
For example, to specify the border color using the style prop
, we need to provide the style prop
value containing our desired border color and border-width
.
In the below example, we use the style prop
of the Text
component of react-native to set the border color of that component. Firstly, we need to create the Text
components, so we have created two Text
components in this example.
Each component uses the style prop
, and in the style prop
, we defined the borderWidth
that needs to be set and the borderColor
. The padding
and margin
are used to visualize the content better.
import { StyleSheet, Text, View } from 'react-native';
export default function App() {
return (
<View style={styles.container}>
<Text style={{ borderWidth: 2, borderColor: 'yellow', padding: 10, margin: 5 }}>Yellow border color with style prop</Text>
<Text style={{ borderWidth: 2, borderColor: 'purple', padding: 10, margin: 5 }}>Purple border color with style prop</Text>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
width: '100%',
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
},
});
Output:
In the above output, users can see two texts with two different border colors, yellow and purple, set up by the style prop
.
This article taught us to set different border colors in the react-native. Apart from these methods discussed in this article, there are many other ways to set the border color, but these are the easiest and most popular ways to color any component’s border.