How to Animate SVG in React Native
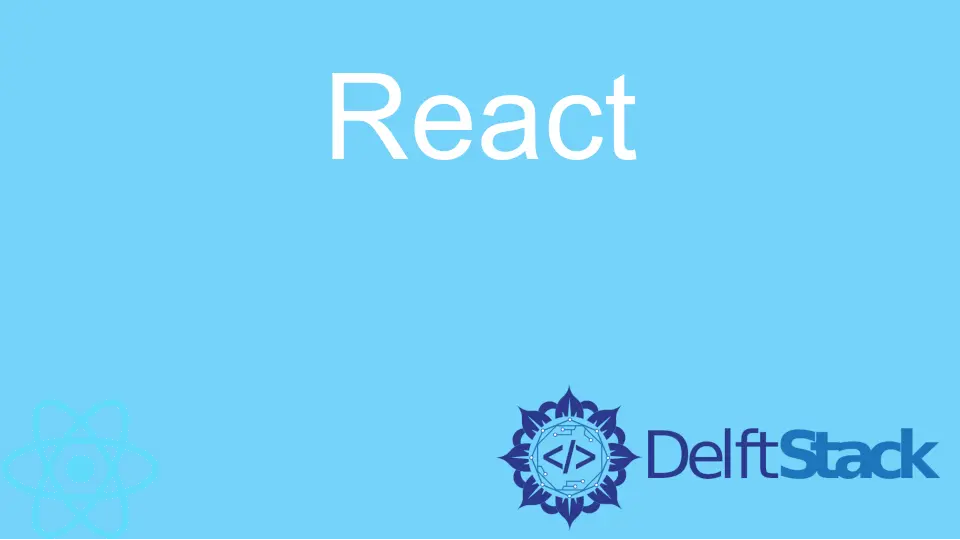
In this article, React Native developers will learn to animate SVG images using different libraries.
There is a lot of chance that your users can decrease if you can’t make the UI and UX of the app attractive. To make an attractive UX, adding animations to images is necessary.
Use the Animated
Module From React Native to Animate SVG Images
The Animated
is the module of the React Native library. We can use it to animate text, react components, and SVG images.
In the below example, we use the react-native-svg
library to create the SVG image. Users can run the below command in the project directory to install the react-native-svg
library.
npm i react-native-svg
First, we will create the SVG image using the react-native-svg
library. Here, users can see that we have imported the Circle
module from the react-native-svg
library and passed the props for the circle size and position according to our requirements.
<Svg height="100" width="100">
<Circle cx="50" cy="50" r="50" stroke="red" strokeWidth="3.5" fill="yellow" />
</Svg>
After that, we need to add the images or texts inside the Animated.View
component, which we need to animate. We can also add the styles as props in the Animated.View
component, which contains the opacity
; by changing the opacity, we can animate our circle.
Users can follow the syntax below to add the style and HTML inside the Animated.View
component.
<Animated.View
style={[
{
opacity: opacity,
},
]}
>
{/* add text or images to animate here */}
</Animated.View>
As a final step, users need to change the image’s opacity to animate it.
In the example below, we have created the Animated.View
component and added the SVG image of the Circle inside that. Also, added the opacity
inside the style.
In the below code, there are two buttons to change the opacity of the circle. When the user presses the button with the text Fade In
, it will call the function animateFadeIn()
, which sets the animation duration and new value for the opacity to 1 for the Animated
.
Similarly, When the user presses the button with the text Fade Out
, it will call the function animateFadeOut()
, which sets the animation duration and new value for the opacity to 0.
Example Code:
import React, { useRef } from "react";
import {
Animated,
Text,
View,
StyleSheet,
Button,
SafeAreaView,
} from "react-native";
import { Svg, Circle } from "react-native-svg";
export default function App() {
// animate the circle using the opacity, and the initial value of the opacity is zero
const opacity = useRef(new Animated.Value(0)).current;
const animateFadeIn = () => {
// // make opacity 0 to ` in 3000 milliseconds
Animated.timing(opacity, {
toValue: 1,
duration: 3000,
}).start();
};
const animateFadeOut = () => {
// make opacity 1 to 0 in 3000 milliseconds
Animated.timing(opacity, {
toValue: 0,
duration: 3000,
}).start();
};
return (
<SafeAreaView style={styles.container}>
<Animated.View
style={[
{
// opacity value for the animation
opacity: opacity,
},
]}
>
{/* SVG image of circle */}
<Svg height="100" width="100">
<Circle
cx="50"
cy="50"
r="50"
stroke="red"
strokeWidth="3.5"
fill="yellow"
/>
</Svg>
</Animated.View>
<View style={{ display: "flex", flexDirection: "column" }}>
<Button
style={{ marginVertical: 16 }}
title="Fade In View"
onPress={animateFadeIn}
/>
<Button
style={{ marginVertical: 16 }}
title="Fade Out View"
onPress={animateFadeOut}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: "center",
justifyContent: "center",
},
});
Output:
In the above output, users can see that when the user presses the Fade In
button, a circle appears, and when the presses the Fade Out
button, the circle disappears.
Conclusion
In this article, we learned to animate the SVG images in React-native. Furthermore, users can use the external library, which supports React-native and SVG animation, to animate the SVG images.