React-Native SVG アニメーション
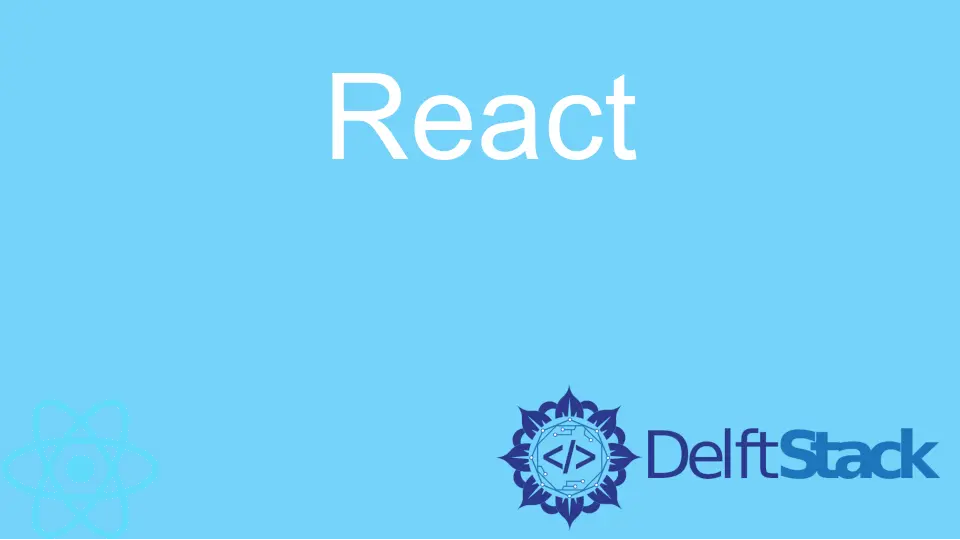
この記事では、React Native 開発者は、さまざまなライブラリを使用して SVG 画像をアニメーション化する方法を学習します。
アプリのUIやUXを魅力的にしないと、ユーザーが減ってしまう可能性が大いにあります。 魅力的なUXを作るためには、画像にアニメーションを追加することが必要です。
React Native の Animated
モジュールを使用して SVG 画像をアニメーション化する
Animated
は React Native ライブラリのモジュールです。 これを使用して、テキストのアニメーション化、コンポーネントの反応、SVG 画像を作成できます。
以下の例では、react-native-svg
ライブラリを使用して SVG イメージを作成します。 ユーザーは、プロジェクト ディレクトリで以下のコマンドを実行して、react-native-svg
ライブラリをインストールできます。
npm i react-native-svg
まず、react-native-svg
ライブラリを使用して SVG イメージを作成します。 ここで、ユーザーは react-native-svg
ライブラリから Circle
モジュールをインポートし、要件に従って円のサイズと位置の小道具を渡したことがわかります。
<Svg height="100" width="100">
<Circle cx="50" cy="50" r="50" stroke="red" strokeWidth="3.5" fill="yellow" />
</Svg>
その後、アニメーション化する必要がある Animated.View
コンポーネント内に画像またはテキストを追加する必要があります。 opacity
を含む Animated.View
コンポーネントにスタイルを小道具として追加することもできます。 不透明度を変更することで、円をアニメーション化できます。
ユーザーは以下の構文に従って、Animated.View
コンポーネント内にスタイルと HTML を追加できます。
<Animated.View
style={[
{
opacity: opacity,
},
]}
>
{/* add text or images to animate here */}
</Animated.View>
最後のステップとして、ユーザーは画像の不透明度を変更してアニメーション化する必要があります。
以下の例では、Animated.View
コンポーネントを作成し、その中に円の SVG 画像を追加しました。 また、スタイル内に opacity
を追加しました。
以下のコードには、円の不透明度を変更するための 2つのボタンがあります。 ユーザーがテキスト Fade In
を含むボタンを押すと、関数 animateFadeIn()
が呼び出され、アニメーションの継続時間と不透明度の新しい値が Animated
の 1 に設定されます。
同様に、ユーザーがテキスト Fade Out
のボタンを押すと、関数 animateFadeOut()
が呼び出され、アニメーションの長さと不透明度の新しい値が 0 に設定されます。
コード例:
import React, { useRef } from "react";
import {
Animated,
Text,
View,
StyleSheet,
Button,
SafeAreaView,
} from "react-native";
import { Svg, Circle } from "react-native-svg";
export default function App() {
// animate the circle using the opacity, and the initial value of the opacity is zero
const opacity = useRef(new Animated.Value(0)).current;
const animateFadeIn = () => {
// // make opacity 0 to ` in 3000 milliseconds
Animated.timing(opacity, {
toValue: 1,
duration: 3000,
}).start();
};
const animateFadeOut = () => {
// make opacity 1 to 0 in 3000 milliseconds
Animated.timing(opacity, {
toValue: 0,
duration: 3000,
}).start();
};
return (
<SafeAreaView style={styles.container}>
<Animated.View
style={[
{
// opacity value for the animation
opacity: opacity,
},
]}
>
{/* SVG image of circle */}
<Svg height="100" width="100">
<Circle
cx="50"
cy="50"
r="50"
stroke="red"
strokeWidth="3.5"
fill="yellow"
/>
</Svg>
</Animated.View>
<View style={{ display: "flex", flexDirection: "column" }}>
<Button
style={{ marginVertical: 16 }}
title="Fade In View"
onPress={animateFadeIn}
/>
<Button
style={{ marginVertical: 16 }}
title="Fade Out View"
onPress={animateFadeOut}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: "center",
justifyContent: "center",
},
});
出力:
上記の出力では、ユーザーが Fade In
ボタンを押すと円が表示され、Fade Out
ボタンを押すと円が消えることがわかります。
まとめ
この記事では、React-native で SVG 画像をアニメーション化する方法を学びました。 さらに、ユーザーは React-native と SVG アニメーションをサポートする外部ライブラリを使用して、SVG 画像をアニメーション化できます。