React Native setTimeout メソッド
Shiv Yadav
2024年2月15日
React Native
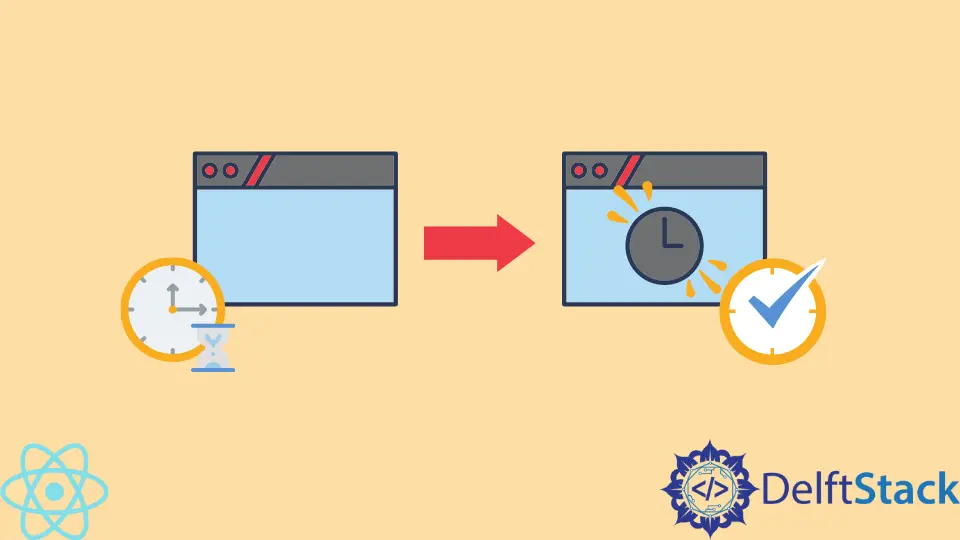
この短い記事では、React Native で setTimeout
を実装する方法を示します。
React Native で setTimeout
メソッドを使用する
数秒後にコードを実行する必要がある場合があります。 そのような状況では、React Native の JavaScript API setTimeout
を使用します。
setTimeout
メソッドは、指定された時間が経過した後に関数を実行します。
コード例:
import React from 'react';
import {Button, Image, StyleSheet, View} from 'react-native';
const App = () => {
const [image, setImage] = React.useState(null);
const showImage = () => {
setTimeout(() => {
setImage(
'https://www.casio.com/content/dam/casio/product-info/locales/us/en/timepiece/product/watch/G/GM/gm2/gm-2100-1a/assets/GM-2100-1A.png.transform/main-visual-pc/image.png');
}, 3000);
};
return (
<View style={styles.container}>
<View style={styles.imageContainer}>
<Image
source={
{ uri: image }}
style={
{ width: '100%', height: 160 }}
resizeMode='contain'
/>
</View>
<View style={styles.buttonContainer}>
<Button
title="Click On the button to see the image"
onPress={() => showImage()}
/>
</View>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 0.85,
justifyContent: 'center',
paddingHorizontal: 10,
},
buttonContainer: {
marginTop: 10,
},
imageContainer: {
justifyContent: 'center',
alignItems: 'center',
},
});
export default App;
ここでは、setTimeOut
メソッドを使用して画像の読み込みを遅らせるコードがあります。これにより、画像の読み込みが 3000 ミリ秒、正確には 3 秒遅くなります。 setTimeOut
メソッドを使用する showImage
メソッドを宣言しました。
showImage
メソッドはボタンで呼び出され、JavaScript の onPress
メソッドを使用してトリガーされます。
出力:
ボタンをクリックすると3秒後に画像が表示されます。
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Shiv Yadav
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn