React Native setTimeout Method
- Understanding setTimeout in React Native
- Implementing setTimeout for Delayed Page Loading
- Enhancing User Experience with setTimeout
- Conclusion
- FAQ
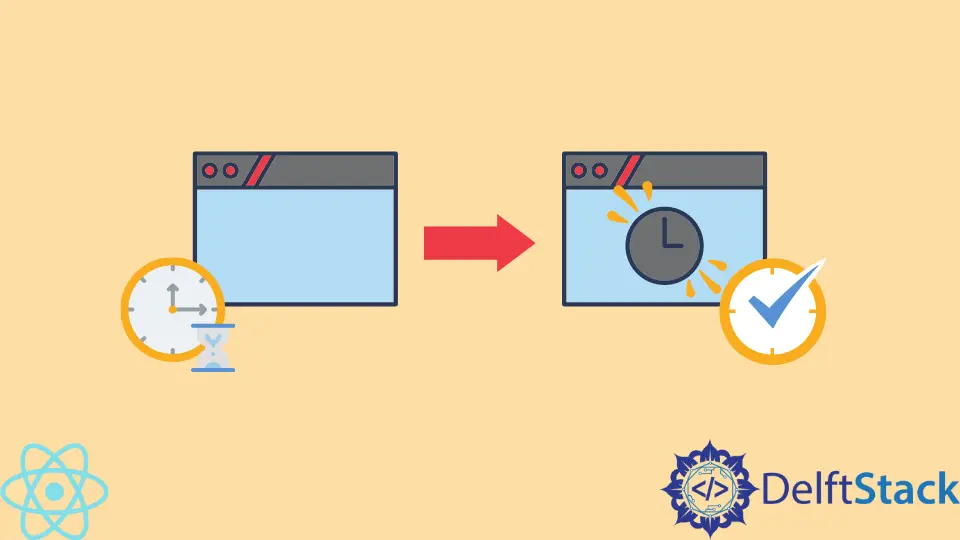
In the fast-paced world of mobile app development, user experience is paramount. One way to enhance this experience is by controlling the loading times of pages within your React Native application. The setTimeout
method is a powerful tool that allows developers to introduce delays in their code execution.
This article will guide you through using the setTimeout
method to delay the loading of pages in React Native. By the end, you will have a clear understanding of how to implement this method effectively, ensuring a smoother and more engaging user experience.
Understanding setTimeout in React Native
The setTimeout
function is a built-in JavaScript method that executes a specified function after a designated period. In React Native, this can be particularly useful for creating loading screens or delaying the rendering of components until certain conditions are met.
To use setTimeout
, you need to pass two parameters: a callback function that contains the code you want to execute and the time delay in milliseconds. For example, if you want to delay a function by 2 seconds, you would set it to 2000 milliseconds.
Here’s a simple example of how it works:
setTimeout(() => {
console.log('This message is delayed by 2 seconds');
}, 2000);
Output:
This message is delayed by 2 seconds
In this example, the message will only appear after a 2-second pause, demonstrating how setTimeout
can control timing in your React Native applications.
Implementing setTimeout for Delayed Page Loading
To delay the loading of a page in your React Native application, you can integrate the setTimeout
method within the component’s lifecycle. This approach allows you to manage the visibility of your main content effectively.
Here’s a basic implementation using functional components and hooks:
import React, { useState, useEffect } from 'react';
import { View, Text, ActivityIndicator } from 'react-native';
const DelayedLoadingScreen = () => {
const [loading, setLoading] = useState(true);
useEffect(() => {
const timer = setTimeout(() => {
setLoading(false);
}, 3000);
return () => clearTimeout(timer);
}, []);
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
{loading ? (
<ActivityIndicator size="large" color="#0000ff" />
) : (
<Text>Welcome to the App!</Text>
)}
</View>
);
};
export default DelayedLoadingScreen;
Output:
Welcome to the App!
In this code, we use the useState
hook to manage the loading state. Initially, the loading
state is set to true, which displays an ActivityIndicator
. The useEffect
hook sets up a timer that changes the loading
state to false after 3 seconds, allowing the main content to render. This method provides a smooth transition from a loading screen to the main app interface.
Enhancing User Experience with setTimeout
Using setTimeout
not only helps in delaying content but also enhances user experience by providing visual feedback during loading times. Users are less likely to feel frustrated when they see a loading indicator, as it assures them that the app is processing their request.
You can further customize the loading experience by incorporating animations or different loading indicators. For instance, you could use libraries like Lottie for more engaging animations. Here’s a quick example of how to integrate a Lottie animation with a delay:
import React, { useState, useEffect } from 'react';
import { View } from 'react-native';
import LottieView from 'lottie-react-native';
const AnimatedLoadingScreen = () => {
const [loading, setLoading] = useState(true);
useEffect(() => {
const timer = setTimeout(() => {
setLoading(false);
}, 3000);
return () => clearTimeout(timer);
}, []);
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
{loading ? (
<LottieView source={require('./loading.json')} autoPlay loop />
) : (
<Text>Welcome to the App!</Text>
)}
</View>
);
};
export default AnimatedLoadingScreen;
Output:
Welcome to the App!
In this example, we replace the ActivityIndicator
with a Lottie animation. This not only makes the loading screen visually appealing but also provides a more dynamic experience for users. The use of setTimeout
still controls when the main content appears, ensuring that the app remains responsive.
Conclusion
The setTimeout
method in React Native is a versatile tool that can significantly improve the user experience by managing loading times effectively. By incorporating this method, you can create seamless transitions between loading screens and your main content. Whether you choose to use simple loading indicators or engaging animations, the key is to keep users informed and engaged during wait times. As you continue to develop your React Native applications, consider how the setTimeout
method can enhance your overall design and functionality.
FAQ
-
what is the purpose of setTimeout in React Native?
setTimeout is used to delay the execution of a function, allowing developers to manage loading times and enhance user experience. -
can setTimeout be used in class components?
Yes, setTimeout can be used in class components by setting the state in the component lifecycle methods like componentDidMount. -
how do I clear a timeout in React Native?
You can clear a timeout by using the clearTimeout function, typically in the cleanup function of the useEffect hook or componentWillUnmount in class components. -
is setTimeout blocking or non-blocking?
setTimeout is non-blocking; it allows the rest of the code to execute while waiting for the specified delay to complete. -
how can I create a loading screen using setTimeout?
You can create a loading screen by setting a loading state to true, displaying a loading indicator, and using setTimeout to change the state after a specified delay.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn