How to Align Text Vertically in React-Native
-
Use the
justifyContent
andflex
Properties to Align Text Vertically at the Center in React-Native -
Use the
alignItems
andflex
CSS Properties to Align Text Vertically at the Center in React-Native
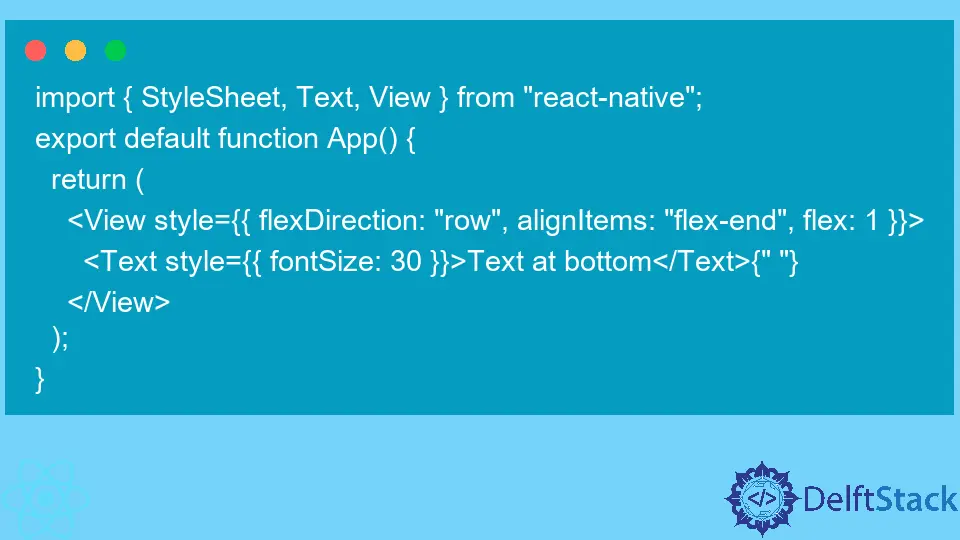
In this article, we will learn to align the text vertically in React-native. Using the methods below, we can align any component in React-native, not just texts.
Use the justifyContent
and flex
Properties to Align Text Vertically at the Center in React-Native
Users can customize the CSS of any component and can align it vertically. We can use the justifyContent
and flex
properties of CSS to align the text vertically center or at the bottom or any other position.
In the example below, we have created the container using the View
component, which contains the Text
component as a child component. Also, we have created the center
style and applied it to the View
component.
In the center
style, we have added the flex: 1
, representing how the View
component should grow. As we have only a single View
component with CSS flex
property, it will take 100% height, and justifyContent: center
will center the contents of the View
component by considering its size according to the flex
property.
Example Code:
import { StyleSheet, Text, View } from "react-native";
export default function App() {
return (
<View style={styles.center}>
<Text>This is vertically Center Text.</Text>
</View>
);
}
const styles = StyleSheet.create({
center: {
flex: 1,
justifyContent: "center",
},
});
Output:
In the above output, users can see that text is aligned center vertically.
Use the alignItems
and flex
CSS Properties to Align Text Vertically at the Center in React-Native
As the justifyContent
CSS property, alignItems
also allows us to center the text vertically. When we use the center
value for the alignItems
CSS property, it aligns the texts vertically center.
Users also need to use the property with the alignItems
CSS property to set how the container should grow. In simple terms, the flex
property defines the component’s height, and according to the height, the alignItems
property aligns the text.
In the example below, we have created the two Text
components and applied the flexDirection: ‘row’
CSS to the parent View
component to show all Text
components in a single row.
Also, in the parent View
component, we have applied the flex:1
style, which sets the component’s height to 100%, and alignItems: center
to vertically center the texts.
Example Code:
import { StyleSheet, Text, View } from "react-native";
export default function App() {
return (
<View style={{ flexDirection: "row", alignItems: "center", flex:1, }}>
<View>
{" "}
<Text style={{fontSize:30,}}>Hi</Text>{" "}
</View>
<View>
{" "}
<Text style={{fontSize:30,}}>There</Text>{" "}
</View>
</View>
);
}
Output:
If users want to align the text at the bottom, they need to change the values of the alignItems
CSS property to flex-end
and the top change value to flex-start
.
To align text at the bottom, users can follow the below example code.
import { StyleSheet, Text, View } from "react-native";
export default function App() {
return (
<View style={{ flexDirection: "row", alignItems: "flex-end", flex: 1 }}>
<Text style={{ fontSize: 30 }}>Text at bottom</Text>{" "}
</View>
);
}
Output:
In the above output, users can see that as we have set the value of alignItems
to flex-end
, it aligns the text at the bottom.
Users learned various methods to align texts vertically in this article. Users can use the justifyContent
or alignItems
CSS property with the flex
CSS property.
Here, we only used the flex
property to set the container height. Users can also try setting height manually.
Related Article - React Native
- How to Set Font Weight in React Native
- How to Align Text in React-Native
- How to Animate SVG in React Native
- How to Set the Border Color in React-Native
- React Native Foreach Loop