How to Set the Text Color in React Native
- Using the StyleSheet API
- Inline Styling
- Dynamic Styling Based on State
- Using External Stylesheets
- Conclusion
- FAQ
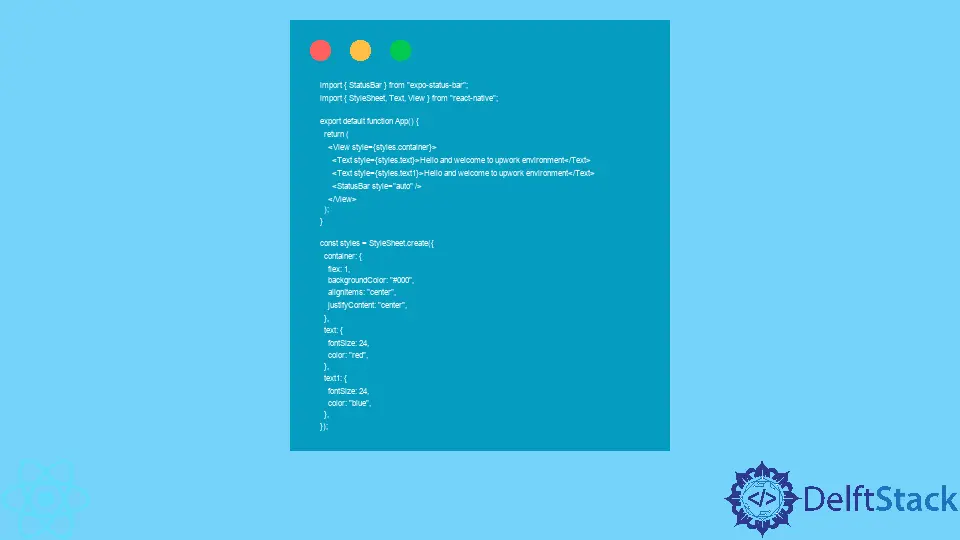
When developing mobile applications with React Native, one of the essential aspects is styling your text. The color of your text can significantly affect the user experience and overall aesthetics of your app.
In this article, we will explore various methods to set the text color in React Native. Whether you’re building a simple app or a complex one, understanding how to manipulate text color will enhance your design capabilities. We’ll cover straightforward techniques, provide code examples, and explain how each method works. By the end of this article, you’ll have a solid grasp of setting text color in your React Native applications.
Using the StyleSheet API
One of the most common methods to set the text color in React Native is by using the built-in StyleSheet API. This approach allows you to define styles in a centralized manner, making your code cleaner and more maintainable. The StyleSheet API is efficient and helps in optimizing the performance of your application.
Here’s a simple example:
import React from 'react';
import { Text, View, StyleSheet } from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello, World!</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#f5fcff',
},
text: {
color: 'blue',
fontSize: 20,
},
});
export default App;
In this example, we create a simple React Native application that displays “Hello, World!” in blue text. The StyleSheet.create
method is used to define a styles
object, which holds the styles for both the container and the text. By setting the color
property to ‘blue’, we change the text color accordingly. This method is highly recommended for its clarity and ease of use, especially when managing larger applications with multiple components.
Inline Styling
Another way to set text color in React Native is through inline styling. This method allows you to apply styles directly to the component without creating a separate style sheet. While this can be convenient for small projects or quick prototypes, it may lead to less maintainable code in larger applications.
Here’s how you can use inline styling:
import React from 'react';
import { Text, View } from 'react-native';
const App = () => {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center', backgroundColor: '#f5fcff' }}>
<Text style={{ color: 'green', fontSize: 20 }}>Hello, World!</Text>
</View>
);
};
export default App;
In this snippet, we set the text color to green directly within the Text
component using the style
prop. This method is straightforward and can be useful for quick changes or small components. However, as your project scales, you may find it beneficial to switch back to using the StyleSheet API for better organization and readability.
Dynamic Styling Based on State
In some cases, you may want to change the text color dynamically based on certain conditions, such as user interactions or state changes. React Native makes it easy to achieve this by combining state management with inline styles or the StyleSheet API.
Here’s an example of how to implement dynamic styling:
import React, { useState } from 'react';
import { Text, View, Button, StyleSheet } from 'react-native';
const App = () => {
const [isRed, setIsRed] = useState(false);
return (
<View style={styles.container}>
<Text style={{ color: isRed ? 'red' : 'black', fontSize: 20 }}>
Hello, World!
</Text>
<Button title="Change Color" onPress={() => setIsRed(!isRed)} />
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#f5fcff',
},
});
export default App;
In this example, we use the useState
hook to manage the isRed
state. The text color changes between red and black each time the button is pressed. This dynamic approach allows for a more interactive user experience, making your application feel more responsive and engaging.
Using External Stylesheets
For larger applications, you may want to separate your styles into external stylesheets. This method keeps your components clean and allows for better organization, especially when working with multiple screens or components that share styles.
Here’s how to do it:
import React from 'react';
import { Text, View } from 'react-native';
import styles from './styles'; // Assuming styles.js is in the same directory
const App = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello, World!</Text>
</View>
);
};
export default App;
In a separate file named styles.js
, you would have:
import { StyleSheet } from 'react-native';
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#f5fcff',
},
text: {
color: 'purple',
fontSize: 20,
},
});
export default styles;
By exporting the styles
object from a separate file, you can easily import and use it in your components. This method promotes reusability and keeps your code organized, especially when you have multiple components that require similar styling.
Conclusion
Setting the text color in React Native is a fundamental skill that can greatly enhance the visual appeal of your application. Whether you choose to use the StyleSheet API, inline styles, dynamic styling, or external stylesheets, each method has its own advantages. By understanding these techniques, you can create more engaging and visually appealing applications. As you continue to develop your skills in React Native, remember that clean and organized code will lead to better maintainability and a smoother development process.
FAQ
-
How can I change the text color in React Native?
You can change the text color in React Native using the StyleSheet API, inline styles, or by using dynamic styling based on state. -
What is the best practice for styling in React Native?
The best practice is to use the StyleSheet API for better organization, readability, and performance, especially in larger applications. -
Can I change text color dynamically in React Native?
Yes, you can change the text color dynamically by using state management in combination with inline styles or the StyleSheet API. -
Is it better to use inline styles or external stylesheets?
For small components, inline styles can be convenient, but for larger applications, external stylesheets are preferred for better organization and maintainability. -
What are some common text colors used in React Native?
Common text colors include hex codes like #000000 (black), #FFFFFF (white), #FF0000 (red), and named colors like ‘blue’, ‘green’, and ‘purple’.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn