How to Check if Input Is Integer in Python
-
Use the
int()
Function to Check if the Input Is an Integer in Python -
Use the
isnumeric()
Method to Check if the Input Is an Integer or Not in Python -
Use the
isdigit()
Method to Check if the Input Is an Integer or Not in Python - Use Regular Expressions to Check if the Input Is an Integer in Python
- Conclusion
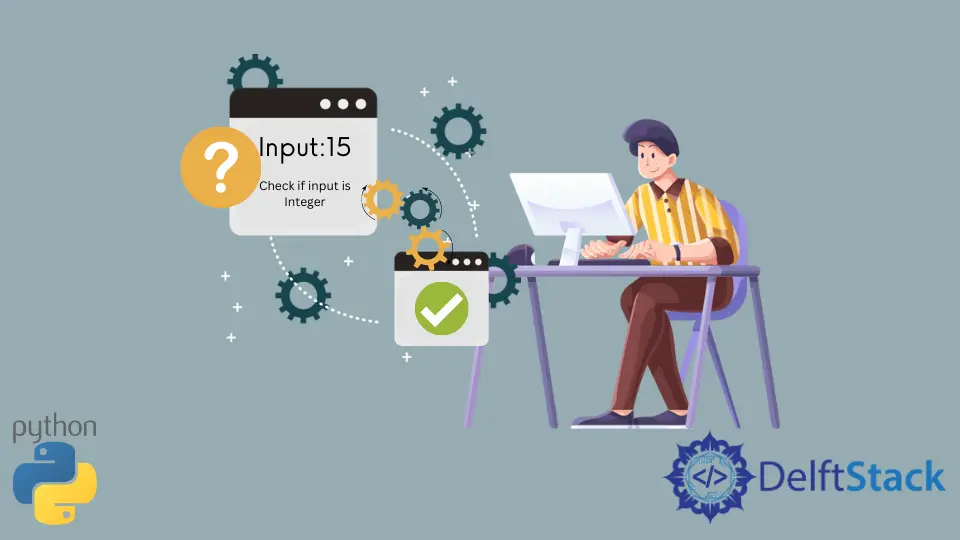
In the world of programming, user input is a cornerstone of interaction, and Python provides a versatile tool for this— the input()
function.
This function empowers users to input their preferences seamlessly. Yet, when precision is paramount, and we need to validate a specific data type, complexities arise.
The input()
function, by default, interprets user entries as strings, complicating the straightforward application of traditional type-checking methods. In essence, we find ourselves in a situation where we must examine whether the entered string contains numeric values.
This tutorial explores Python’s input handling, particularly on the challenge of validating user input for integer types. We will see how to check if a string entered by the user is of integer type or not in Python.
Use the int()
Function to Check if the Input Is an Integer in Python
When dealing with the task of verifying whether a user-entered string represents an integer, a combination of the int()
conversion function and the try-except
block proves to be a powerful and elegant solution.
The process begins with the int()
function, which attempts to convert the user-input string into an integer. The try
block encapsulates this conversion, allowing us to proceed with the assumption that the input is indeed a valid integer.
However, should the conversion encounter any issues, such as a non-integer string, a ValueError
is raised. This is where the except
block comes into play.
By catching this exception, we prevent our program from crashing and gracefully handle the situation, providing an opportunity to inform the user about the nature of the input error.
Let’s explore a practical implementation of this concept through a code example:
user_input = input("Your input: ")
try:
int_value = int(user_input)
print("The input is an integer:", int_value)
except ValueError:
print("The input is not an integer. Please enter a valid integer.")
Output:
Your input: 21
The input is an integer: 21
Here, we initiate the process by prompting the user to input a string using the input()
function, and the provided string is stored in the variable user_input
.
Moving into the try
block, we use int(user_input)
to attempt the conversion. If successful, the resulting integer value is stored in the variable int_value
, and a message confirming the input as an integer is printed.
In the event of a conversion failure, signified by the occurrence of a ValueError
, the flow of control shifts to the except
block. Within this block, we print a message notifying the user that the input is not an integer and request a valid integer input.
This dual-step mechanism ensures that our program remains resilient, handling unexpected user inputs gracefully without abrupt crashes.
Use the isnumeric()
Method to Check if the Input Is an Integer or Not in Python
While the int()
and try-except
block combination is a robust method for verifying if a user-input string represents an integer, another approach involves using the built-in isnumeric()
method. This method is applicable to string objects and can be employed to determine if the characters within the string are numeric.
This method returns True
if all characters in the string are numeric and False
otherwise.
Here’s an example of using the isnumeric()
method to check if a string entered by the user is an integer:
user_input = input("Your input: ")
if user_input.isnumeric():
print("The input is an integer:", user_input)
else:
print("The input is not an integer. Please enter a valid integer.")
Output:
Your input: 15
The input is an integer: 15
In this example, we begin by obtaining a string input from the user using the input()
function, storing it in the variable user_input
. The subsequent if
statement checks if the input string is numeric using the isnumeric()
method.
If this method returns True
, indicating that all characters in the string are numeric, a message is printed confirming that the input is an integer.
If the isnumeric()
method returns False
, the else
block is executed, printing a message to notify the user that the input is not an integer and prompting them to enter a valid integer.
This approach is particularly useful when the objective is to validate a string without the need for exception handling, offering a concise and readable solution for integer validation in user inputs.
Use the isdigit()
Method to Check if the Input Is an Integer or Not in Python
In Python, the isdigit()
method is another valuable tool for determining if a user-input string represents an integer. Similar to isnumeric()
, isdigit()
is a string method that checks if all characters in a given string are digits.
The isdigit()
method is a built-in Python method that returns True
if all the characters in the string are digits. Otherwise, it returns False
.
This makes it particularly useful for checking if a string can be safely converted to an integer. The method evaluates each character in the string, ensuring that they are all numeric digits.
Here’s an example of using the isdigit()
method to check if a user-entered string is an integer:
user_input = input("Your input: ")
if user_input.isdigit():
print("The input is an integer:", user_input)
else:
print("The input is not an integer. Please enter a valid integer.")
Output:
Your input: 28
The input is an integer: 28
Starting with the acquisition of user input through the input()
function and storing it in the variable user_input
, we proceed to the if
statement.
Here, the isdigit()
method is employed to evaluate whether the characters in the input string are all digits. If this evaluation yields True
, indicating that the string consists solely of numeric digits, a message is printed, confirming that the input is an integer.
Conversely, if the isdigit()
method returns False
, signifying the presence of non-numeric characters, the else
block is executed. Within this block, a message is printed to notify the user that the input is not an integer, prompting them to provide a valid integer.
By leveraging the isdigit()
method, we offer an additional avenue for checking if a string is an integer in Python. This method provides a concise and effective way to validate user inputs without the need for extensive exception handling, contributing to the overall clarity and readability of the code.
Use Regular Expressions to Check if the Input Is an Integer in Python
Regular expressions, often referred to as regex or regexp, are sequences of characters that define a search pattern. In Python, the re
module allows us to work with regular expressions.
To check if a string is an integer using regex, we construct a pattern that matches the expected format of an integer. The re.match()
function is then used to apply this pattern to the input string.
If a match is found, the string is considered an integer.
Here’s an example of using regular expressions to check if a user-entered string is an integer:
import re
user_input = input("Your input: ")
integer_pattern = re.compile(r"^[0-9]+$")
if integer_pattern.match(user_input):
print("The input is an integer:", user_input)
else:
print("The input is not an integer. Please enter a valid integer.")
Output:
Your input: 45
The input is an integer: 45
Beginning with the user input obtained through input()
, the re
module is imported to work with regular expressions. We define a regex pattern, ^[0-9]+$
, using re.compile()
, where ^
indicates the start of the string, [0-9]
matches any digit, and $
denotes the end of the string.
Moving to the if
statement, we use the match()
function to check if the input string matches the defined pattern. If a match is found, indicating that the string consists entirely of numeric digits, a message is printed, confirming that the input is an integer.
If no match is found, the else
block is executed, printing a message notifying the user that the input is not an integer and prompting them to provide a valid integer.
Conclusion
Validating user inputs for integers is an important task in Python, and this article has shed light on multiple strategies to achieve this goal.
Whether leveraging the int()
function with the try-except
block, using the isnumeric()
and isdigit()
methods, or employing regular expressions, each method contributes a unique perspective to integer validation.
Armed with this knowledge, you can choose the approach that best fits your specific use case, ensuring a resilient and user-friendly experience when handling integer inputs in Python.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python