How to Simulate Keyboard Inputs in Python
-
Simulate Keyboard Using the
keyboard
Library in Python -
Simulate Keyboard Using the
PyAutoGUI
Library in Python
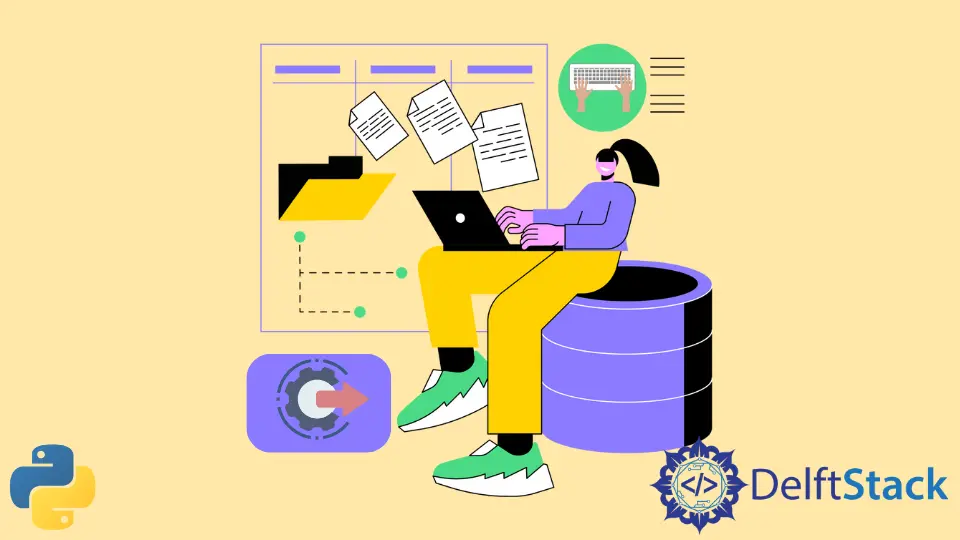
Python is used for almost anything. Using Python, we can develop backends for web applications, backends for mobile applications, and APIs using free and open-source frameworks such as Django
and Flask
.
What’s more, Python programs also create efficient machine learning models using robust libraries such as Keras
, NumPy
, Tensorflow
, and PyTorch
, which plot various kinds of plots using Matplotlib
, and much more.
In this article, we will see such use cases of Python. We will learn how to simulate or control the keyboard using Python.
We will talk about two open-source Python libraries, keyboard
and PyAutoGUI
, letting us control our keyboard using Python scripts.
Simulate Keyboard Using the keyboard
Library in Python
The keyboard
library is an open-source library to take control of your keyboard.
This library can listen to and send keyboard events, use hotkeys, support internationalization, and provide mouse support with the help of the mouse
library, which we can download using pip install mouse
or pip3 install mouse
.
To install the keyboard
library, use either of the following two pip
commands.
pip install keyboard
pip3 install keyboard
Let us understand how to use this library to control a keyboard. Refer to the following Python code for a simple example that types some text.
import keyboard
keyboard.write("Python is an amazing programming language.")
keyboard.press_and_release("enter")
keyboard.press_and_release("shift+p")
keyboard.press_and_release("y")
keyboard.press_and_release("t")
keyboard.press_and_release("h")
keyboard.press_and_release("o")
keyboard.press_and_release("n")
Output:
Python is an amazing programming language.
Python
Before running the above code, take note of your text cursor or caret. The text above inside the output box will get typed there automatically.
The write()
function will type whatever string is passed to this function as an argument. This function sends artificial keyboard events to the operating system, which gets further typed at the caret.
If any character is not available on the keyboard, explicit Unicode characters are typed instead. The press_and_release()
function sends operating system events to perform hotkeys and type characters passed as arguments.
To understand more about this library, refer to its documentation here.
Simulate Keyboard Using the PyAutoGUI
Library in Python
The PyAutoGUI
library lets us write Python scripts to control the keyboard and mouse.
This library can move the mouse cursor and click over windows and applications, send key events to type characters and execute hotkeys, take screenshots, move, resize, minimize, maximize, and locate applications on the screen, and display alert messages, etc.
To install this library, use either of the following commands.
pip install pyautogui
pip3 install pyautogui
We can use the PyAutoGUI
library for our use case. Refer to the following code for this.
import pyautogui
pyautogui.write("Python is an amazing programming language.")
Output:
Python is an amazing programming language.
As we can see, the write()
function types character of the string passed as an argument at the caret. This function can only press single character keys such as alphabets and numbers.
This means we can not press keys such as Shift, Ctrl, Command, Alt, Option, F1, and F3. We can use the keyDown()
and keyUp()
methods to press such keys.
The keyDown()
method presses a key and keeps holding it. And the keyUp()
method releases a held key.
Refer to the following Python code for an example. Do not forget to note the position of your text cursor or caret.
import pyautogui
pyautogui.keyDown("shift")
pyautogui.press("a")
pyautogui.press("b")
pyautogui.press("c")
pyautogui.keyUp("shift")
pyautogui.press("x")
pyautogui.press("y")
pyautogui.press("z")
pyautogui.keyDown("shift")
pyautogui.press("a")
pyautogui.keyUp("shift")
pyautogui.keyDown("shift")
pyautogui.press("b")
pyautogui.keyUp("shift")
pyautogui.keyDown("shift")
pyautogui.press("c")
pyautogui.keyUp("shift")
Output:
ABCxyzABC
To press keys such as Shift+F, we can also use the press()
method. This function will press whatever keys are passed as a string.
Behind the scenes, this function is just a wrapper for the keyDown()
and keyUp()
methods.
To understand more about this library, refer to its documentation here.