How to Get Multiple-Line Input in Python
-
Using the
raw_input()
Function to Get Multiline Input From a User in Python -
Using
sys.stdin.read()
Function to Get Multiline Input From a User in Python -
Using a
loop
to Get Multiline Input From a User in Python - Conclusion
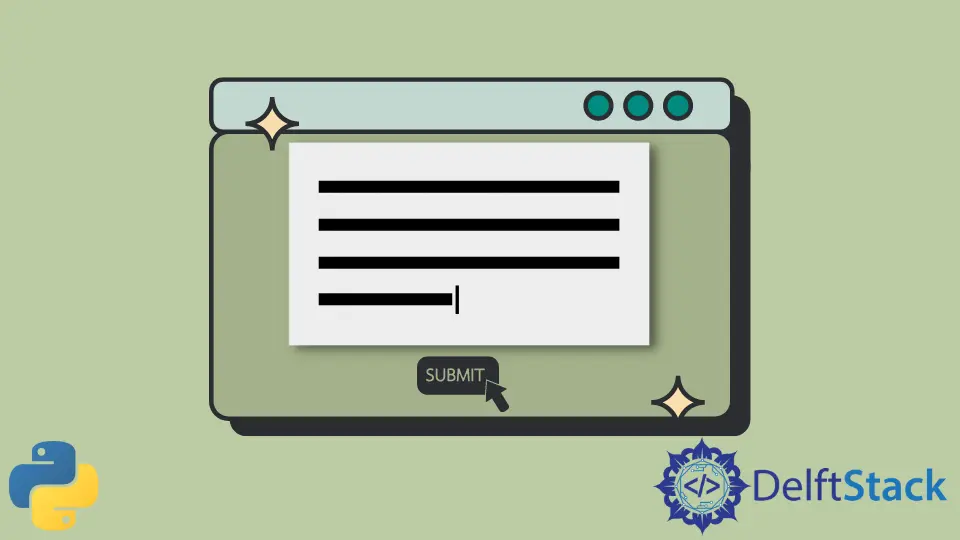
The program sometimes may require an input that is vastly longer than the default single line input.
This tutorial demonstrates the various ways available to get multiline input from a user in Python.
This comprehensive guide offers clear, practical code examples, making it a valuable resource for Python programmers at all levels, ensuring they are well-equipped to handle multiline user input in diverse programming contexts.
Using the raw_input()
Function to Get Multiline Input From a User in Python
The raw_input()
function can be utilized to take in user input from the user in Python 2. However, the use of this function alone does not implement the task at hand.
The primary purpose of using iter()
with raw_input()
is to create a loop that continuously accepts input from the user until a specific termination condition is met.
Syntax and Explanation
The iter()
function in Python is a built-in function that allows the creation of an iterator from an iterable object. When used with two arguments, iter(callable, sentinel)
, it calls the callable object until the sentinel value is returned.
callable
: In our case, this israw_input()
, which reads a line from the input.sentinel
: This is the value that, when returned by the callable, causes the iterator to stop.
Let us move on to show how to implement this function in the correct way in Python.
The following code uses the raw_input()
function to get multiline input from a user in Python.
print "Enter your text (type 'stop' to end):"
for line in iter(raw_input, 'stop'):
print "You entered:", line
In this code, we initiate a loop that continually prompts the user for input. The iter()
function takes raw_input
as the callable.
Each time raw_input
is called, it waits for the user to enter a line of text. The second argument to iter()
, 'stop'
, is our sentinel value.
This means the loop will continue until the user types stop
.
Inside the loop, each input line is immediately printed back to the user with a preceding message "You entered:"
. This immediate feedback loop can be useful in interactive applications where the user’s input is processed or acknowledged as soon as it’s entered.
Output:
Further, after the introduction of Python 3, the raw_input()
function became obsolete and was replaced by the new input()
function.
Therefore, if using Python 3 or higher, we can utilize the input()
function instead of the raw_input()
function.
The above code can be simply tweaked in order to make it usable in Python 3.
print("Enter your text (type 'END' to finish):")
for line in iter(input, "END"):
print("Received:", line)
In our code, we’ve set up an iterative loop that continuously gathers user input. The iter()
function is given two parameters: the input()
function and a sentinel value 'END'
.
The loop runs, repeatedly calling input()
for user input until the user enters 'END'
which stops the iteration.
Within the loop, we immediately process and display each line entered by the user with a prefixed message "Received:"
. This prompt response can be particularly beneficial in interactive applications where immediate processing or acknowledgment of user input is required.
Output:
Using sys.stdin.read()
Function to Get Multiline Input From a User in Python
The sys
module can be imported to the Python code and is mainly utilized for maintaining and manipulating the Python runtime environment.
The sys.stdin.read()
function is one such function that is a part of the sys
module and can be utilized to take multiline input from the user in both Python 2 and Python 3.
Syntax and Explanation
The sys.stdin.read()
method is part of the sys
module, so you first need to import sys
.
The method can optionally take an integer parameter n
, which specifies the number of characters to read. If n
is not provided, it reads until the end of the file or until [an EOF (End Of File) is received]({{relref “/HowTo/Python/python end of file.en.md”}}).
sys.stdin.read([n])
n
(optional): The number of characters to read. If omitted, reads until EOF.
import sys
print(
"Enter/paste your content. Press Ctrl+D (Unix) or Ctrl+Z (Windows) then Enter to save it."
)
input_text = sys.stdin.read()
print("You entered:\n" + input_text)
In this code, we first import the sys
module to access the standard input functions. We prompt the user to enter or paste their content and inform them how to signify they have finished (using Ctrl + D or Ctrl + Z).
The sys.stdin.read()
method then captures all the input until the EOF marker. After the input has been captured, we print it back out to the user, prefixed by "You entered:"
.
This approach is particularly suitable for console-based applications where users might input large blocks of text or when the input might be redirected from files.
Output:
While sys.stdin.read()
is ideal for scenarios where the input is treated as a continuous block of text, sys.stdin.readlines()
comes into play when each line of input is significant on its own, requiring a method that neatly captures and organizes these lines into a manageable format.
The sys.stdin.readlines()
method is a part of the sys
module and is designed to read all lines from the standard input until an EOF (End Of File) is reached.
This method does not require any parameters, and it returns a list where each element is a line of input.
import sys
print("Enter your text (end input with Ctrl+D on Unix or Ctrl+Z on Windows):")
lines = sys.stdin.readlines()
print("You entered:")
for line in lines:
print(line, end="")
In our script, we start by importing the sys
module to access the standard input functionalities. We prompt the user to input their text and signify the end of input with Ctrl + D (Unix/Linux/Mac) or Ctrl + Z (Windows).
The sys.stdin.readlines()
reads all the input lines until EOF and stores them as a list of strings in the variable lines
.
After the input is captured, we iterate through the lines
list and print each line. Note that we use print(line, end='')
to avoid adding extra newline characters, as each line already ends with a newline.
This method is particularly useful for applications where each line of input needs to be processed separately or stored for later use.
Output:
Using a loop
to Get Multiline Input From a User in Python
Using a loop for multiline input is particularly useful in scenarios where the number of input lines is not fixed in advance. This method is often employed in command-line applications, data entry programs, and simple text processing tools.
It allows the program to dynamically respond to user input, line by line until a specific condition is met (such as entering a particular exit
command or pressing a key combination like Ctrl + D).
print("Enter your text (type 'exit' to finish):")
user_input = ""
while True:
line = input()
if line.lower() == "exit":
break
user_input += line + "\n"
print("You entered:\n" + user_input)
In this example, we initiate an infinite loop using while True
. Within this loop, we use the input()
function to read the user’s input line by line.
The loop checks if the user enters exit
(regardless of the case, as we use lower()
for case-insensitive comparison). If exit
is detected, the loop terminates with break
.
Each line entered by the user is appended to the user_input
string, followed by a newline character to preserve the line structure. After exiting the loop, the program prints out the complete text entered by the user.
Output:
Conclusion
We explored diverse approaches, starting from the raw_input()
function in Python 2, adapting it to Python 3’s input()
function, and utilizing the iter()
function for an efficient looping mechanism. Transitioning to the sys
module, we delved into sys.stdin.read()
for reading large text blocks and sys.stdin.readlines()
for handling input line by line, each serving unique scenarios in Python programming.
Finally, the simplicity and adaptability of using a loop for dynamic multiline input collection were showcased. This versatile method caters to scenarios where the input’s length is unpredictable, proving its efficacy in real-world applications.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn