How to Get User Input With Timeout in Python
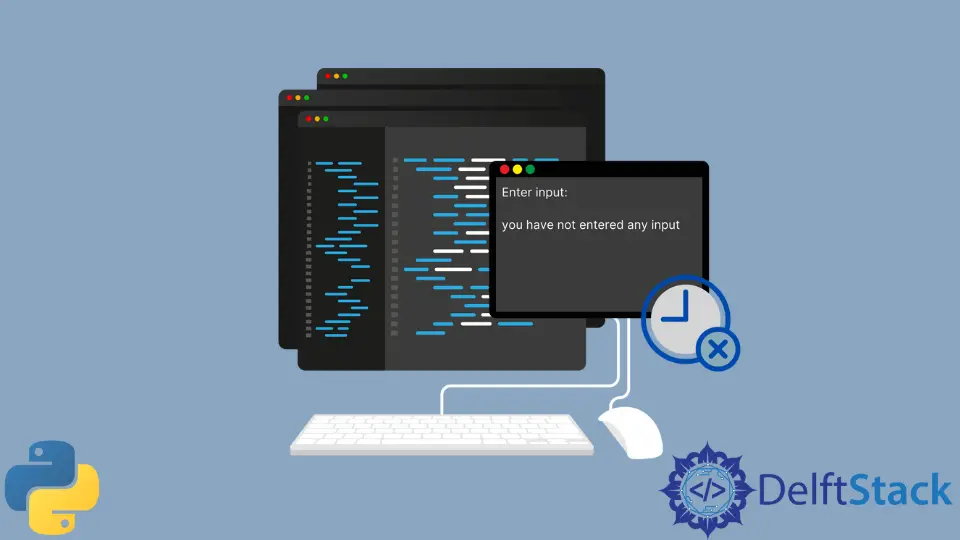
This tutorial will discuss getting input from a user with a timeout period using Python’s inputimeout
library.
Python Get User Input With Timeout
If we want to take input from a user within a specific period, like 5 seconds, we can use the inputimeout
library of Python. We can use pip
to install this library using the below line of code.
pip install inputimeout
We can run this command in PowerShell or a command prompt to install the inputimeout
library. Make sure pip
is installed and added to the PATH
before running the above command.
If pip
is not added to the path, we have to provide the full path to the pip.exe
file along with its name to use in the PowerShell
or command prompt. The example usage is shown below.
C:\Users\ammar\AppData\Local\Programs\Python\Python39\Scripts\pip.exe install inputimeout
If inputimeout
is installed without any errors, we can import the inputimeout
and the TimeoutOccured
modules to use in our code. The inputimeout()
function will take input from the user, and we can pass two arguments inside it.
The first argument, prompt
, is the message we want to show to the user while taking input; this argument is optional. The second argument, timeout
, is used to set the time in seconds, and the function will wait till the given time. After that, it will raise an exception, and we can use the exception name TimeoutOccured
, which we can use with the try-except
statement to avoid an error.
from inputimeout import inputimeout, TimeoutOccurred
try:
user_input = inputimeout(prompt="Enter input: \n", timeout=5)
except TimeoutOccurred:
user_input = "you have not entered any input"
print(user_input)
Output:
Enter input:
you have not entered any input
In the above code, we used Python’s try-except
statement to avoid the exception if the user did not enter any input in the given time. We used the name of the exception to set the input to something else, like a default value.
If the user enters input, it will be printed, and if the user does not enter any input during the given timeout, the default input will be printed. The input is saved in the user_input
variable.
Check this link for more details about the inputimeout
library.