How to Detect Keypress in Python
-
Detect KeyPress Using the
keyboard
Module in Python -
Detect KeyPress Using the
pynput
Module in Python -
Detect KeyPress Using the
readchar
Module in Python - Conclusion
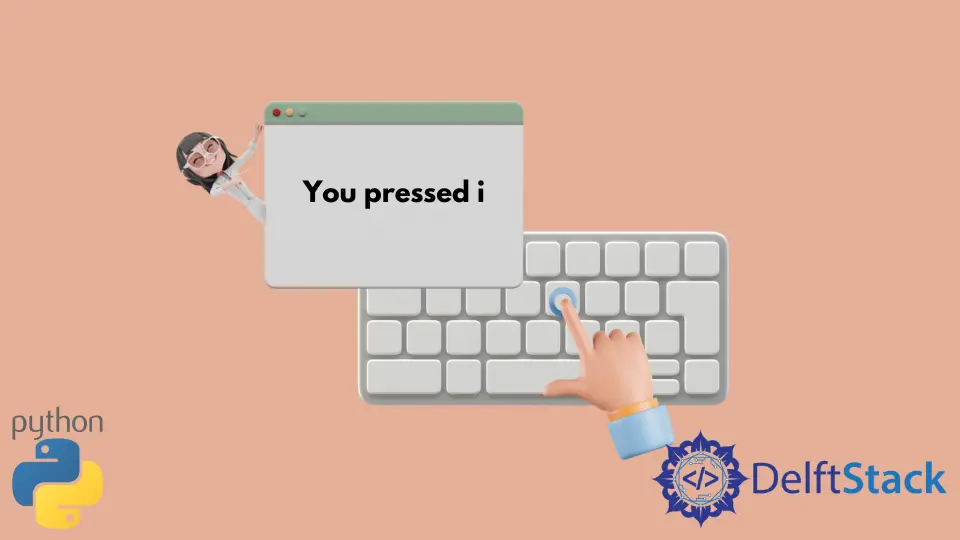
If you need access to hardware like input devices such as the keyboard, there are modules available in Python that can make your lives much easier. Using such modules, you can easily perform the task you want without dealing with the complexities of the system.
In this article, you will learn how to detect keypress using modules in Python. There are many modules used to detect keypress in Python, and out of which, the three most popular and widely used modules are keyboard
, pynput
, and readchar
.
Detect KeyPress Using the keyboard
Module in Python
The keyboard
module allows us to take full control of the keyboard and comes with various predefined methods to choose from. These methods make it much easier for us to work with the keyboard and detect the user’s physical keypresses on the keyboard.
To install the keyboard
module, execute the below command inside your command prompt or terminal.
pip3 install keyboard
Example
import keyboard
def on_key_event(event):
if event.event_type == "down":
print(f"Key {event.name} pressed")
keyboard.hook(on_key_event)
# This will block the script and wait until 'esc' is pressed
keyboard.wait("esc")
In this script, we first import the keyboard
library. We then define a function on_key_event
, which will be called every time a key event occurs.
Inside this function, we check if the event type is 'down'
, meaning a key press. If it is, we print out the name of the key that was pressed.
The keyboard.hook(on_key_event)
line sets up the hook with our on_key_event
function. This means our function will be called for every keyboard event (both key press and release).
However, in our function, we only take action on key press events.
The last line, keyboard.wait('esc')
, is crucial as it keeps our script running and waits until the escape key (ESC) is pressed. Without this line, the script would end immediately, not allowing us to capture any key events.
Output:
Detect KeyPress Using the pynput
Module in Python
The pynput
module is used to detect and control input devices, mainly mouse and keyboard. But in this tutorial, you will only see how to use this module for detecting keypress on the keyboard.
Before using this module, you first have to install it using the command below.
pip3 install pynput
To use this module for detecting keypress, you first have to import keyboard
from the pynput
module.
Example
from pynput import keyboard
def on_press(key):
try:
print(f"Key {key.char} pressed")
except AttributeError:
print(f"Special key {key} pressed")
def on_release(key):
print(f"Key {key} released")
if key == keyboard.Key.esc:
# Stop listener
return False
# Collect events until released
with keyboard.Listener(on_press=on_press, on_release=on_release) as listener:
listener.join()
In our script, we start by importing the keyboard
module from the pynput
library. We define two functions, on_press
and on_release
, to handle key press and release events, respectively.
In the on_press
function, we print out the key that was pressed. If it’s a special key (like Ctrl, Alt, etc.), it won’t have a char
attribute, and we handle this using a try
-except
block.
The on_release
function prints out which key was released, and if the escape key is pressed, it returns False
, which stops the listener from listening to more key events.
The keyboard.Listener
is used as a context manager that starts the listening process. The listener.join()
method is called to wait until the listener stops, which happens when the escape key is pressed.
Output:
Detect KeyPress Using the readchar
Module in Python
While there are multiple methods to capture key inputs in Python, the readchar
library offers a simple and efficient way to detect single keypresses. This library is especially useful for creating interactive CLI applications that require immediate user input without the need to press the Enter key.
pip3 install readchar
Example
import readchar
def detect_keypress():
print("Press any key (Press 'q' to quit):")
while True:
key = readchar.readkey()
if key == "q":
print("Exiting...")
break
else:
print(f"Key '{key}' pressed")
detect_keypress()
In our script, we first import the readchar
library. The function detect_keypress
is defined to continuously detect and respond to keypress events.
It prompts the user to press any key, entering a loop that waits for a keypress.
The readchar.readkey()
function captures a single keypress and stores it in the variable key
. If the key is q
, the program prints Exiting...
and breaks the loop, effectively ending the program.
For any other key, the script prints out the key that was pressed.
Output:
Conclusion
In the realm of Python programming, the ability to detect keypresses opens the door to creating more dynamic and interactive applications. This article has navigated through the usage of three pivotal Python modules - keyboard
, pynput
, and readchar
.
Each module caters to different needs: keyboard
for comprehensive keyboard control, pynput
for handling both keyboard and mouse inputs, and readchar
for simple, direct key detection. These tools are invaluable for developing applications ranging from CLI-based games to responsive software that requires real-time user input.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn