How to Take Input of Unspecified Length in Python
-
Use
stdin
to Take Input of Unspecified Length in Python -
Use the
while
Loop to Take Input of Unspecified Length in Python -
Use an Equivalent of C++
cin
Function to Take Input of Unspecified Length in Python
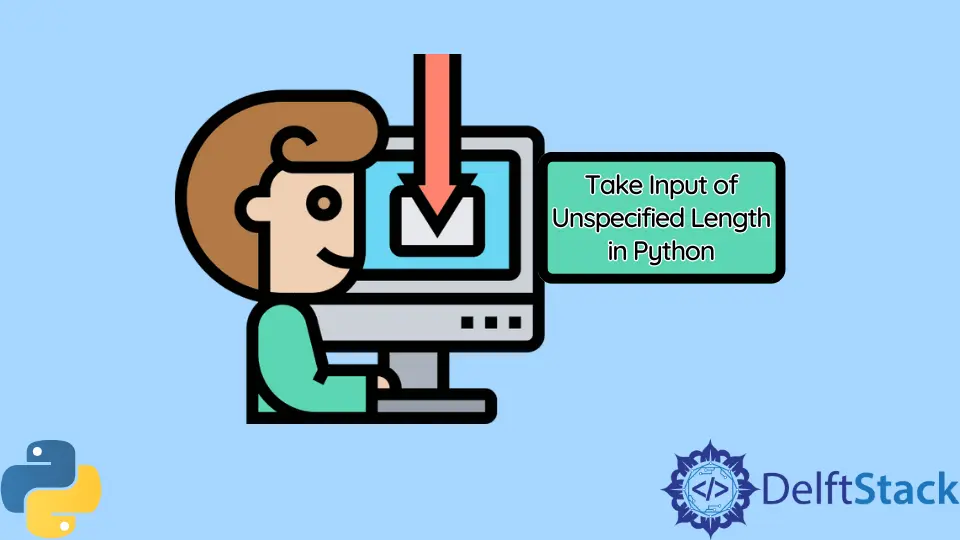
This article will introduce how we can take multiple inputs in Python when the input length is unknown.
Use stdin
to Take Input of Unspecified Length in Python
To take input using the sys
module, we can use sys.stdin
.
The Python stdin
stands for standard input, and it enables us to take input from the command line directly. While taking input, stdin
calls the input()
method internally and adds a \n
after each line.
Example:
import sys
print("Input something")
for x in sys.stdin:
if "end" == x.rstrip():
break
print(f"Either input something or input end to quit: {x}")
print("Exit")
Output:
Input something
Pasta
Either input something or input end to quit: Pasta
Watermelon
Either input something or input end to quit: Watermelon
Banana
Either input something or input end to quit: Banana
Noodles
Either input something or input end to quit: Noodles
end
Exit
Here, we first import the sys
module. Then, we use a for
loop to take input continuously.
Inside this for
loop, we have an if
statement to check if the input equals the string end
. If it is, then we break out of the loop.
Note that we can specify any string, like quit
or q
, in place of end
. We have to tell the user what to input to exit.
Note that we can also take input of integer data type in this code. However, it is better to explicitly change the input to an integer since Python converts every input to string.
We can use the int()
function to do this.
import sys
print("Give an input")
for val in sys.stdin:
if "end" == val.rstrip():
break
val = int(val)
print(f"Either input something or input end to quit: {val}")
print("Exit")
Output:
Give an input
10
Either input something or input end to quit: 10
12
Either input something or input end to quit: 12
Dumbledore
Traceback (most recent call last):
File "tutorial.py", line 6, in <module>
x = int(x)
ValueError: invalid literal for int() with base 10: 'Dumbledore\n'
As shown above, we can only input an integer data type or get an error.
Use the while
Loop to Take Input of Unspecified Length in Python
When we do not know the number of times we have to take the input, we can use the while
loop.
Example:
while True:
x = input("Grocery: ")
if x == "done":
break
Output:
Grocery: Vinegar
Grocery: Soya sauce
Grocery: done
Here, we use the True
keyword to define a condition that will evaluate to true each time. This way, the user gets the input prompt continuously.
However, this program will never terminate if we don’t define a break condition. Therefore, inside the while
block, we define a break
condition that will bring the control out of the loop when it receives the value done
.
Note that in place of the string done
, we can use anything like quit
, bye
, 0
, etc.
Example:
while True:
print("Input 0 to exit")
x = int(input("Enter the number: "))
if x == 0:
print("Bye")
break
Output:
Input 0 to exit
Enter the number: 4
Input 0 to exit
Enter the number: 5
Input 0 to exit
Enter the number: 3
Input 0 to exit
Enter the number: 0
Bye
This example tells the user to input 0
to exit. Instead of using the True
keyword with the while
loop, we can also specify a condition that evaluates true.
Use an Equivalent of C++ cin
Function to Take Input of Unspecified Length in Python
Let us make our auxiliary function in Python that works similarly to the below C++ code.
while (cin >> var) {
// code here
}
In C++, we can use the above method to take the input of unspecified length. Note that in C++, cin>>var
works in two ways, as an input operator and as a Boolean expression.
In Python, the equivalent for taking an input is var = input()
, and it only acts as an input channel. We will use a list object named ‘var’ to simulate Python’s cin>>var
behavior.
Example:
# Function that works like the cin function in C++
def cin(var):
try:
var[0] = int(input())
return True
except:
return False
# main function similar to that of C++
def main():
var = [0]
while cin(var):
print("Value:", var[0])
main()
Output
5
Value: 5
7
Value: 7
3
Value: 3
9
Value: 9
6
Value: 6
4
Value: 4
>>>
First, know that var
is a list object. Thus, if we change the value of var[0]
in the auxiliary method, the change will occur in the main
method.
Inside the cin
function, we take the input in the variable, var[0]
. Also, using the try
statement, we return True
each time we get an integer input.
Note that the code stops executing in the above code if we press the Enter key instead of giving input. Not just that, typing anything other than an integer will stop the code execution.
Inside the main
function, we continuously use a while
loop to call the cin
function. In this example, we take integer input, but we can change that according to our needs.